Simplify Primary Key Extraction in Laravel with modelKeys()
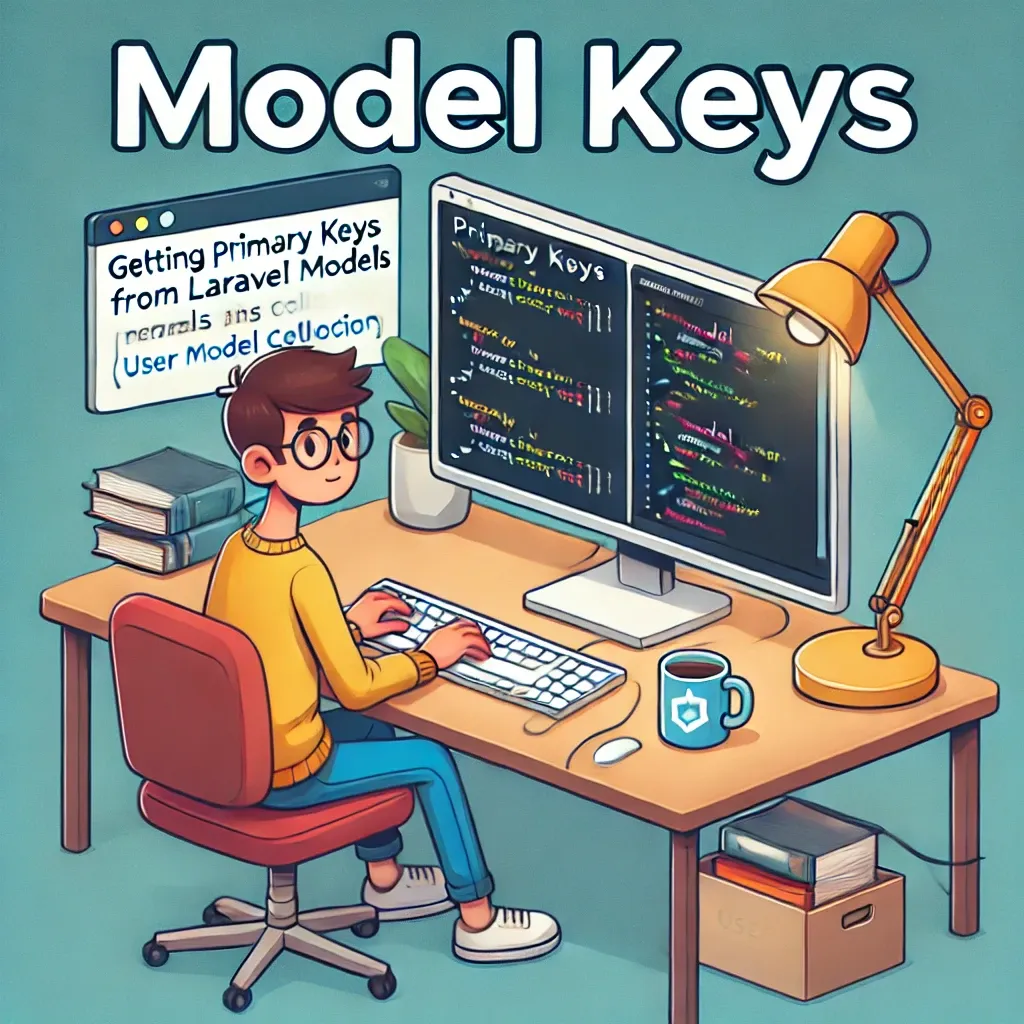
When working with Eloquent collections in Laravel, there are often scenarios where you need to quickly extract the primary keys of all models in the collection. This is where the modelKeys()
method shines, providing a clean and efficient way to accomplish this task.
Understanding modelKeys()
The modelKeys()
method is a powerful tool available on Eloquent collections. It returns an array containing the primary keys of all models in the collection. This simple yet effective method can significantly streamline your code when dealing with collections of models.
Basic Usage
Let's look at a basic example of how to use modelKeys()
:
$users = User::all();
$primaryKeys = $users->modelKeys();
// Result: [1, 2, 3, 4, 5]
In this example, $primaryKeys
will be an array containing the primary key values of all User models in the collection.
Practical Applications
Relationship Syncing: When syncing relationships, you often need just the IDs:
$tagIds = $post->tags->modelKeys();
$post->tags()->sync($tagIds);
Quick Lookups: Create efficient lookup arrays:
$productIds = Product::inStock()->get()->modelKeys();
$inStockLookup = array_flip($productIds);
// Later in your code
if (isset($inStockLookup[$productId])) {
// Product is in stock
}
Caching Strategies: Use model keys to generate cache keys:
$postIds = Post::recent()->get()->modelKeys();
Cache::put('recent_post_ids', $postIds, now()->addHours(24));
Bulk Operations: When performing bulk updates or deletes, you often need a list of IDs:
$userIds = User::where('status', 'inactive')->get()->modelKeys();
User::whereIn('id', $userIds)->delete();
The modelKeys()
method is a simple yet powerful tool in the Laravel developer's toolkit. By providing a quick and clean way to extract primary keys from collections, it can help simplify your code and make certain operations more efficient. Whether you're dealing with bulk operations, caching, or relationship management, modelKeys()
can be a valuable asset in your Laravel projects.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!