Simplify Date Queries with Laravel's New Shorthand Methods
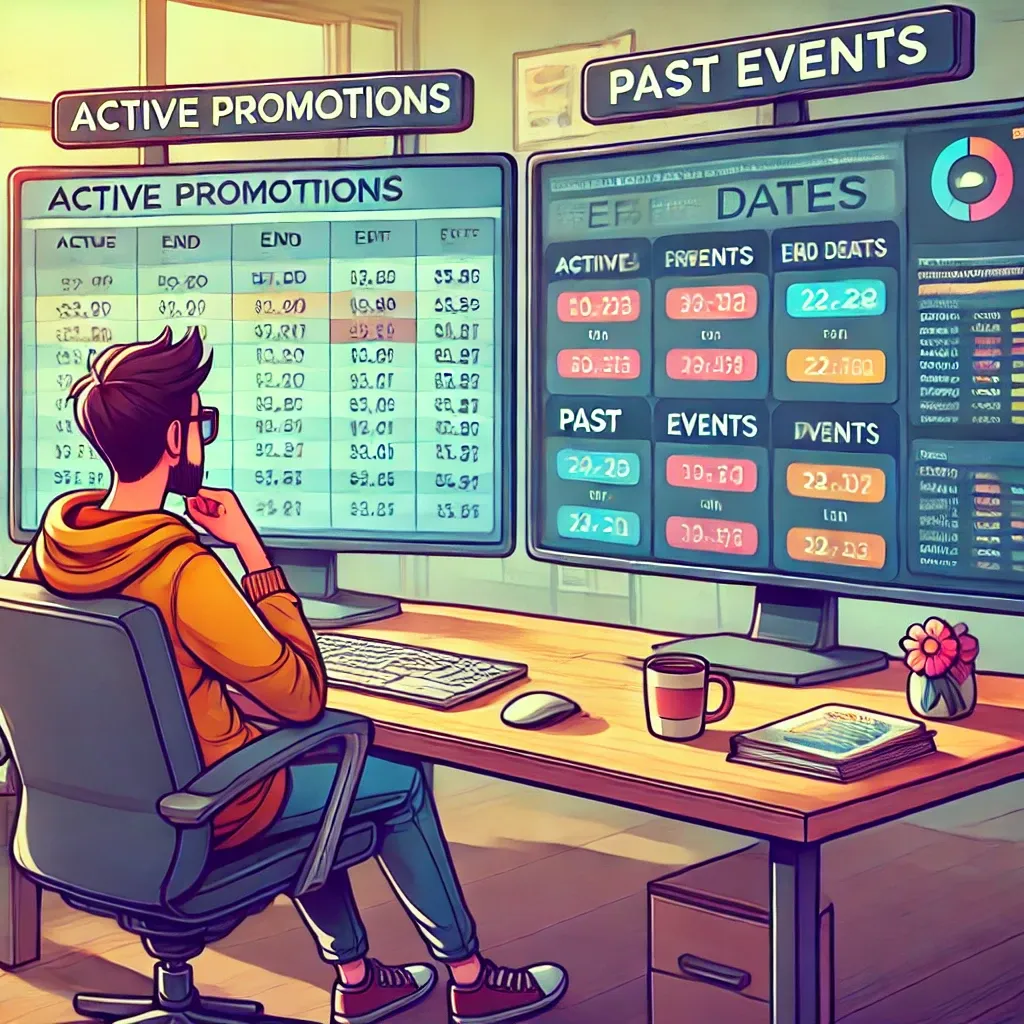
Need to simplify date-based queries in Laravel? The new date shorthand methods provide intuitive ways to work with temporal constraints in your database queries.
Working with dates in database queries often requires verbose comparisons against the current time. Laravel's new date shorthand methods make these common patterns more expressive and readable, reducing boilerplate while improving code clarity.
Let's see how they work:
// Find records with dates in the past
$expiredSubscriptions = Subscription::wherePast('expires_at')->get();
// Find records with dates in the future
$upcomingEvents = Event::whereFuture('starts_at')->get();
// Find records with today's date
$todaysAppointments = Appointment::whereToday('scheduled_for')->get();
Real-World Example
Here's how you might use these methods in a content management system:
class ContentManager
{
public function getPublishedPosts(bool $includeFuture = false)
{
$query = Post::query()
->whereNotNull('published_at');
if (!$includeFuture) {
$query->whereNowOrPast('published_at');
}
return $query->get();
}
public function getScheduledContent()
{
return collect([
'posts' => Post::whereFuture('published_at')->get(),
'events' => Event::whereFuture('starts_at')->get(),
'webinars' => Webinar::whereAfterToday('scheduled_for')->get()
]);
}
public function getArchivedContent()
{
return [
'expired_posts' => Post::wherePast('archived_at')->get(),
'past_events' => Event::whereBeforeToday('ends_at')->get(),
'old_promotions' => Promotion::whereNowOrPast('valid_until')->get()
];
}
}
These date shorthand methods provide a more expressive way to work with time-based queries. Think of them as specialized filters that handle all the date comparison logic for you, making your code more readable and maintaining focus on business logic rather than date manipulation.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!