Simplify Database Error Debugging with Laravel's getRawSql Method
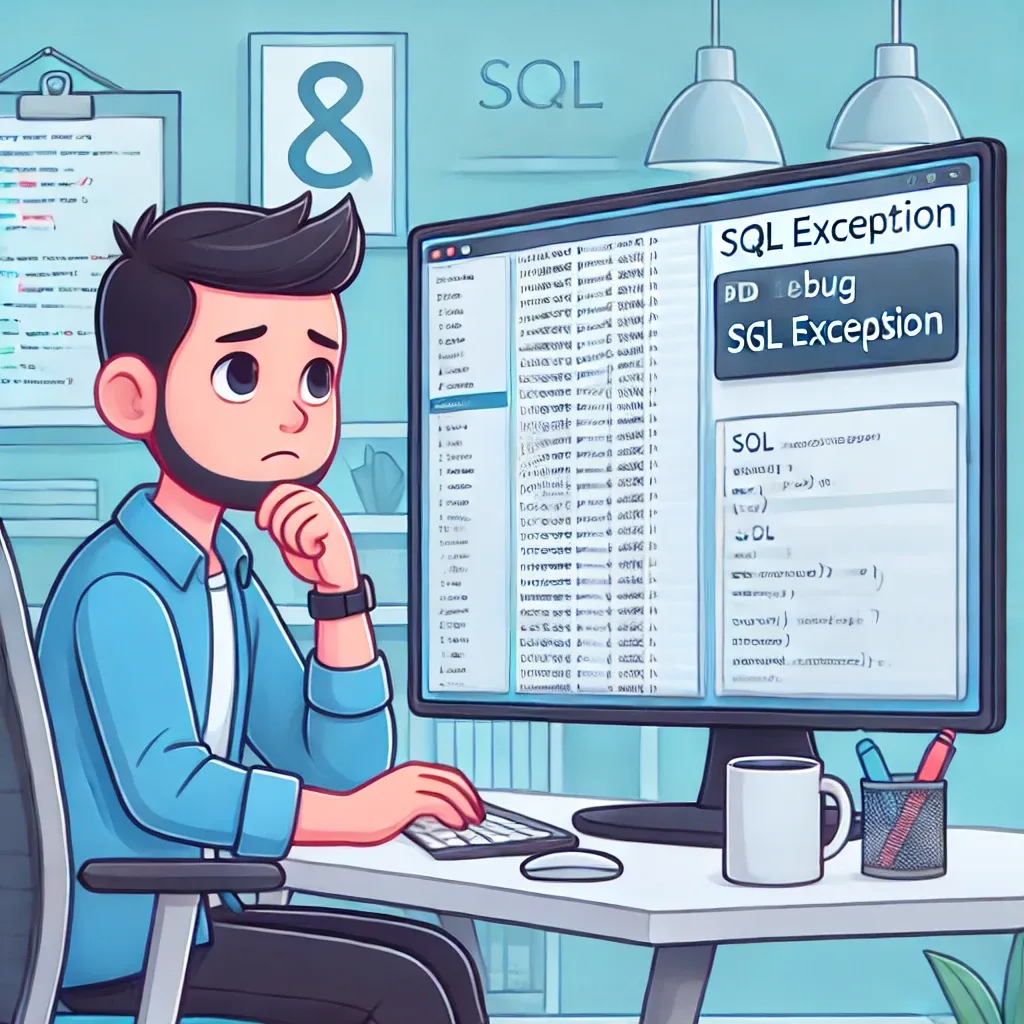
Ever struggled to see the actual SQL that caused a database error in Laravel? The new getRawSql method on QueryException gives you properly formatted queries with all bindings in place.
When debugging database issues, one of the most crucial pieces of information is the exact SQL query that caused the problem. Previously, Laravel provided the query string and bindings separately in QueryException objects, requiring developers to manually piece together the complete query or use string replacement methods that didn't always work correctly.
Let's see how the new getRawSql method works:
try {
// Some database operation
} catch (QueryException $e) {
// Get the complete SQL with bindings
$rawSql = $e->getRawSql();
// Log or display the exact query that failed
Log::error("Database error: {$e->getMessage()} | Query: {$rawSql}");
}
Real-World Example
This method is particularly useful when reporting errors to monitoring systems or logging platforms. Here's a practical example:
// In your exception handler
$this->reportable(function (QueryException $e) {
// Get the complete SQL query with bindings
$rawSql = $e->getRawSql();
// Send to error reporting service
ErrorReporter::capture([
'message' => $e->getMessage(),
'sql' => $rawSql,
'connection' => $e->getConnection()->getName(),
'code' => $e->getCode()
]);
});
You can also use it with Laravel's exception handling system:
// In your App\Exceptions\Handler class
public function register()
{
$this->reportable(function (QueryException $e) {
// Log the complete SQL
Log::channel('sql_errors')->error($e->getRawSql());
return false;
});
}
Or with the new exceptions configuration:
->withExceptions(function (Exceptions $exceptions) {
$exceptions->report(function (QueryException $e) {
// Report to error monitoring service
MyErrorServiceFacade::report('Error executing SQL: ', $e->getRawSql());
});
})
The getRawSql method provides a small but significant improvement to the developer experience, making it easier to understand and fix database errors quickly.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!