Simplify API responses with Laravel's Resource Collections
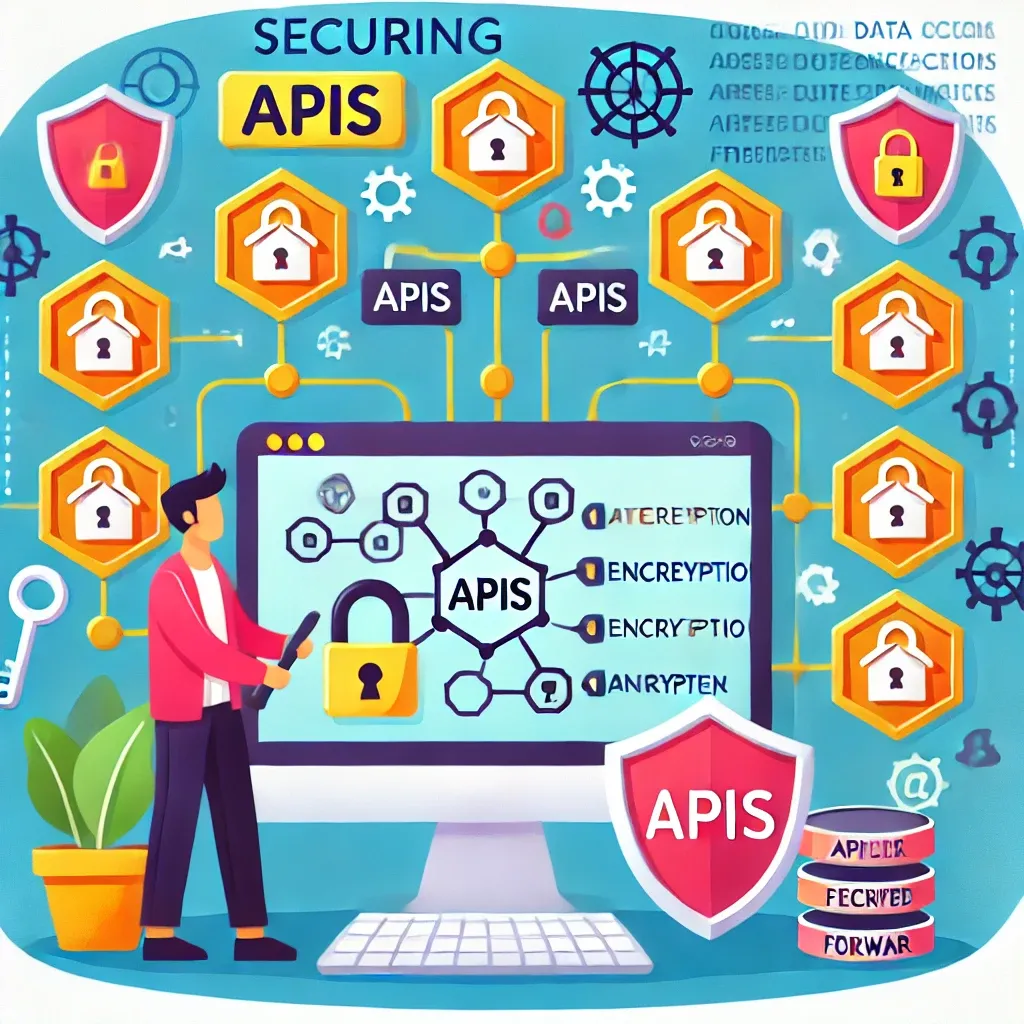
Building APIs that deliver consistent and well-structured responses is crucial for modern web development. Laravel provides a robust way to transform your Eloquent models into JSON responses through Resource Collections. This feature helps you maintain a clean and maintainable codebase, while ensuring that your API responses are consistent and easy to understand.
Understanding Resource Collections
Resource Collections in Laravel allow you to transform a collection of Eloquent models into a structured JSON response. This is particularly useful for APIs, where you need to standardize the format of your responses and include additional metadata or relationships. By using Resource Collections, you can keep your transformation logic separate from your models and controllers, leading to a more modular and organized codebase.
Basic Usage
Here’s a basic example of how to use Resource Collections:
- Creating a Resource Collection:
php artisan make:resource UserResource
- Defining the Resource:
use Illuminate\Http\Resources\Json\JsonResource;
class UserResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'name' => $this->name,
'email' => $this->email,
'created_at' => $this->created_at->toDateTimeString(),
'updated_at' => $this->updated_at->toDateTimeString(),
];
}
}
- Using the Resource in a Controller:
use App\Http\Resources\UserResource;
class UserController extends Controller
{
public function index()
{
$users = User::all();
return UserResource::collection($users);
}
}
In this example, the UserResource
class transforms the User
model into a standardized JSON response. The index
method in the UserController
returns a collection of users using the UserResource
.
Real-Life Example
Consider a scenario where you have a blogging platform and you need to transform a collection of posts into a JSON response. Here’s how you can implement this:
- Creating a Post Resource:
php artisan make:resource PostResource
- Defining the Post Resource:
use Illuminate\Http\Resources\Json\JsonResource;
class PostResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->content,
'author' => new UserResource($this->whenLoaded('author')),
'created_at' => $this->created_at->toDateTimeString(),
'updated_at' => $this->updated_at->toDateTimeString(),
];
}
}
- Using the Post Resource in a Controller:
use App\Http\Resources\PostResource;
class PostController extends Controller
{
public function index()
{
$posts = Post::with('author')->get();
return PostResource::collection($posts);
}
}
In this example, the PostResource
includes the author of the post as a nested resource. The index
method in the PostController
returns a collection of posts, each transformed by the PostResource
. This ensures that the API response includes all necessary information in a consistent format.
Pro Tip
You can customize your resource collections to include additional metadata or relationships as needed. For example, you can add pagination information or include related models conditionally:
use Illuminate\Http\Resources\Json\ResourceCollection;
class UserCollection extends ResourceCollection
{
public function toArray($request)
{
return [
'data' => $this->collection,
'meta' => [
'total_users' => $this->collection->count(),
],
];
}
}
In this example, the UserCollection
includes additional metadata about the total number of users.
Conclusion
Laravel's Resource Collections provide a powerful and flexible way to transform your Eloquent models into consistent and well-structured JSON responses. By leveraging this feature, you can ensure that your API responses are clean, maintainable, and easy to work with.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!