Sharing Data with All Views in Laravel: The Global View Data Guide
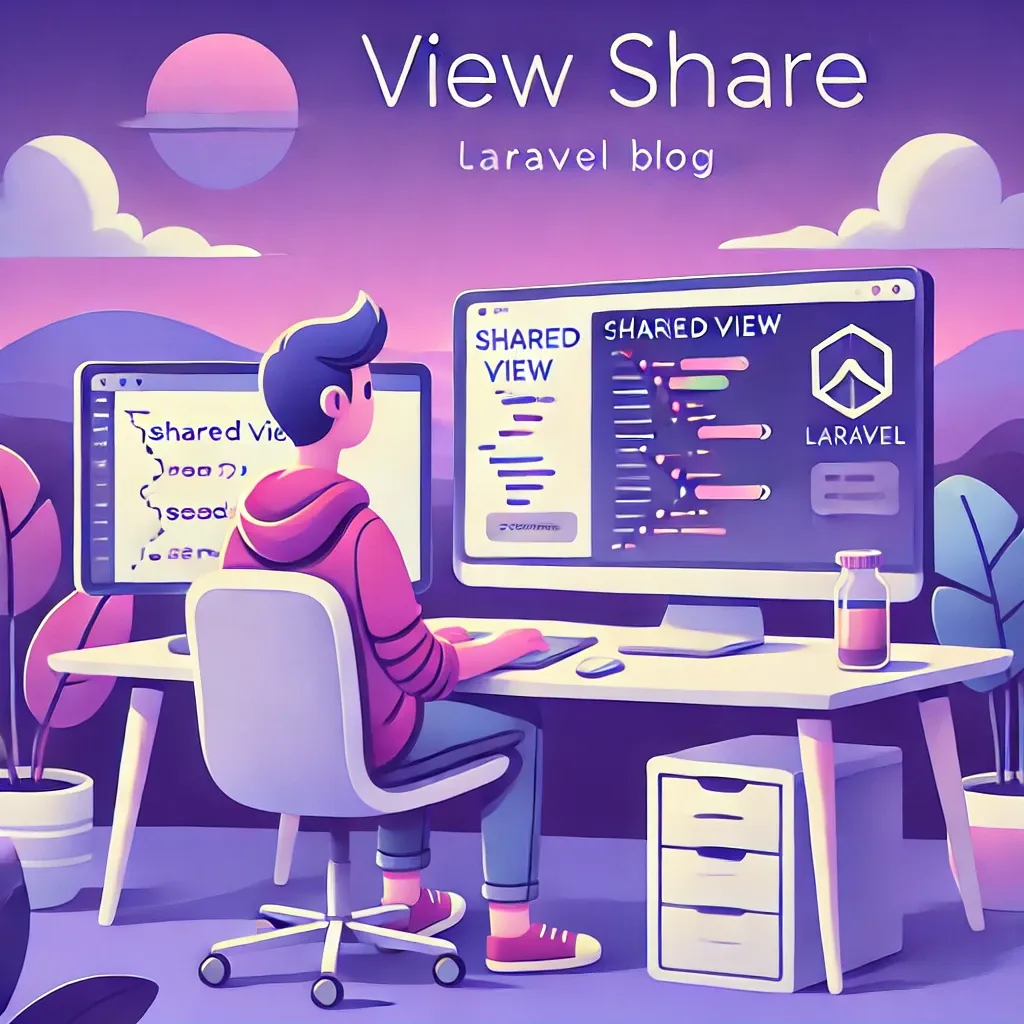
Need to make certain data available across all your views? Laravel's View::share
method makes this a breeze! Let's explore how to effectively share global view data.
Basic View Sharing
Here's how to share data with all views:
use Illuminate\Support\Facades\View;
class AppServiceProvider extends ServiceProvider
{
public function boot(): void
{
View::share('key', 'value');
}
}
Real-World Example
Here's a practical implementation for a SaaS application:
use Illuminate\Support\Facades\View;
use App\Services\SystemSettingsService;
use App\Services\NavigationService;
class ViewServiceProvider extends ServiceProvider
{
public function boot(): void
{
View::share([
'app_name' => config('app.name'),
'support_email' => 'help@example.com',
'current_year' => now()->year
]);
// Share system settings
if (!app()->runningInConsole()) {
$settings = app(SystemSettingsService::class)->getGlobalSettings();
View::share([
'maintenance_mode' => $settings->maintenance_mode,
'system_notice' => $settings->current_notice,
'footer_links' => $settings->footer_navigation
]);
}
// Share user-specific data
View::composer('*', function ($view) {
if ($user = auth()->user()) {
$view->with([
'unread_notifications' => $user->unreadNotifications->count(),
'account_type' => $user->subscription?->type,
'company_name' => $user->company?->name
]);
}
});
}
}
View sharing is perfect for data that needs to be available everywhere in your application, like system settings, user preferences, or global configuration values.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!