Securing Your Laravel App Behind Load Balancers: Mastering the TrustProxies Middleware
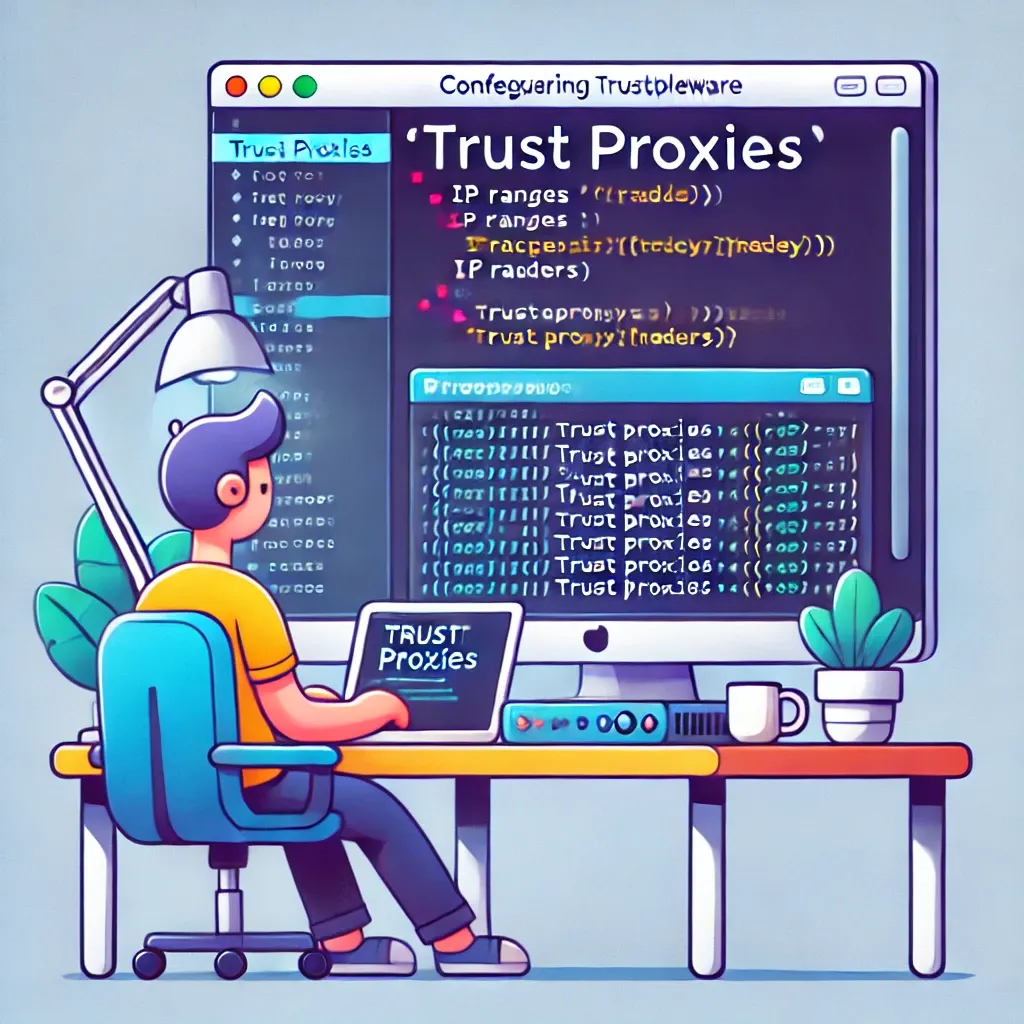
When running Laravel applications behind load balancers or reverse proxies, you might encounter issues with HTTPS detection or incorrect client IP addresses. Laravel's TrustProxies middleware provides a solution to these problems, ensuring your application works correctly in various deployment scenarios.
Understanding the Need for TrustProxies
When your Laravel app sits behind a load balancer or proxy, incoming requests pass through this intermediate layer before reaching your application. This can lead to two main issues:
- HTTPS detection may fail, causing Laravel to generate non-secure URLs.
- The client's IP address may be incorrectly identified as the proxy's IP instead of the actual client.
The TrustProxies middleware allows your application to trust the headers set by your proxy, resolving these issues.
Configuring TrustProxies
To set up TrustProxies, you'll need to modify your application's bootstrap/app.php
file. Here's how you can configure it:
->withMiddleware(function (Middleware $middleware) {
$middleware->trustProxies(at: [
'192.168.1.1',
'10.0.0.0/8',
]);
})
This configuration tells Laravel to trust proxies with the IP addresses 192.168.1.1
and any IP in the 10.0.0.0/8
range.
Trusting All Proxies
In some cases, particularly in cloud environments, you might want to trust all proxies:
->withMiddleware(function (Middleware $middleware) {
$middleware->trustProxies(at: '*');
})
Be cautious with this setting, as it trusts all incoming requests as if they're from a proxy.
Configuring Trusted Headers
You can also specify which headers should be trusted:
use Illuminate\Http\Request;
->withMiddleware(function (Middleware $middleware) {
$middleware->trustProxies(headers: Request::HEADER_X_FORWARDED_FOR |
Request::HEADER_X_FORWARDED_HOST |
Request::HEADER_X_FORWARDED_PORT |
Request::HEADER_X_FORWARDED_PROTO |
Request::HEADER_X_FORWARDED_AWS_ELB
);
})
This configuration trusts the standard forwarded headers as well as those specific to AWS Elastic Load Balancer.
Real-World Scenario: Application Behind AWS ELB
Let's consider a scenario where your Laravel application is deployed behind an AWS Elastic Load Balancer:
use Illuminate\Http\Request;
->withMiddleware(function (Middleware $middleware) {
$middleware->trustProxies(at: '*', headers: Request::HEADER_X_FORWARDED_AWS_ELB);
})
This configuration:
- Trusts all proxies, which is often necessary in cloud environments where proxy IPs can change.
- Specifically trusts the AWS ELB headers for determining the client's protocol, IP, and port.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!