Securing Your Laravel APIs with Built-in Rate Limiting
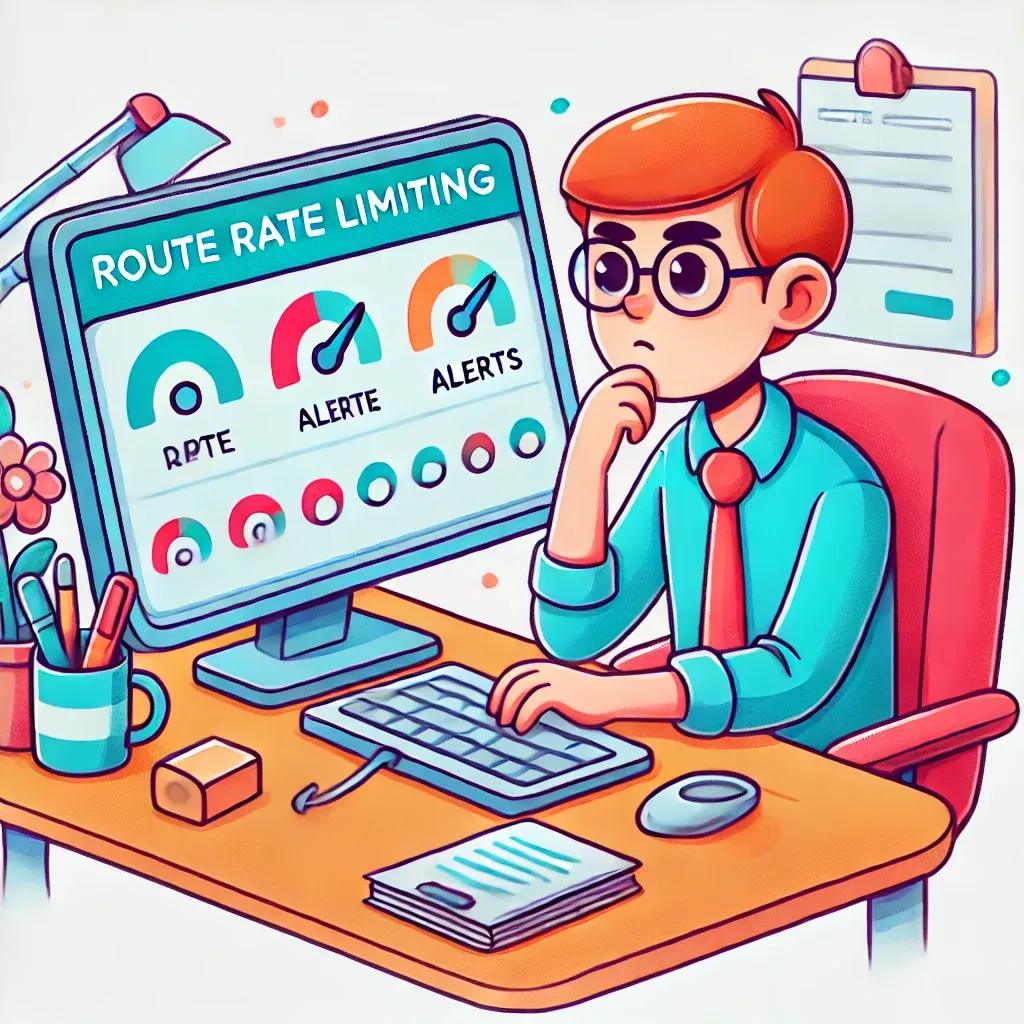
Rate limiting is a crucial aspect of building robust and secure APIs. Laravel provides powerful built-in tools for implementing rate limiting, allowing you to prevent abuse, ensure fair usage, and protect your application's resources. Let's explore how to leverage these features effectively.
Basic Rate Limiting
Laravel's rate limiting is typically applied using middleware. Here's a basic example:
Route::middleware(['auth:api', 'throttle:60,1'])->group(function () {
Route::get('/user', function () {
return auth()->user();
});
});
In this example, authenticated users are limited to 60 requests per minute for the /user
endpoint.
Custom Rate Limiters
For more complex scenarios, you can define custom rate limiters in your AppServiceProvider
or a dedicated service provider:
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
public function boot()
{
RateLimiter::for('api', function (Request $request) {
return Limit::perMinute(60)->by($request->user()?->id ?: $request->ip());
});
}
Then apply it to your routes:
Route::middleware(['throttle:api'])->group(function () {
// API routes
});
Dynamic Rate Limiting
You can create dynamic limits based on user roles or subscription levels:
RateLimiter::for('premium', function (Request $request) {
return $request->user()->isPremium()
? Limit::perMinute(100)
: Limit::perMinute(30);
});
Route::middleware(['auth:api', 'throttle:premium'])->group(function () {
Route::post('/process', [DataController::class, 'process']);
});
This allows premium users more requests per minute compared to regular users.
Global vs. Route-Specific Limits
You can set global limits in your app/Http/Kernel.php
:
protected $middlewareGroups = [
'api' => [
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
];
Or apply specific limits to individual routes:
Route::post('/login', [AuthController::class, 'login'])
->middleware('throttle:5,1'); // 5 attempts per minute
Handling Rate Limit Exceptions
When a rate limit is exceeded, Laravel throws a Illuminate\Http\Exceptions\ThrottleRequestsException
. You can handle this in your app/Exceptions/Handler.php
:
public function render($request, Throwable $exception)
{
if ($exception instanceof ThrottleRequestsException) {
return response()->json([
'error' => 'Too many requests. Please try again later.'
], 429);
}
return parent::render($request, $exception);
}
Advanced Techniques
Sliding Window Rate Limiting
For more precise control, you can implement a sliding window rate limit:
RateLimiter::for('sliding', function (Request $request) {
return Limit::perMinute(60)->by($request->user()?->id ?: $request->ip());
});
Rate Limiting with Redis
For high-traffic applications, you might want to use Redis for rate limiting:
RateLimiter::for('redis', function (Request $request) {
return Limit::perMinute(60)->by($request->user()?->id ?: $request->ip())->response(function () {
return response('Custom rate limit exceeded message', 429);
});
});
Combining Multiple Limits
You can apply multiple rate limits to a single route:
Route::middleware(['throttle:global,throttle:api'])->group(function () {
// Routes that need to satisfy both global and API-specific limits
});
By effectively utilizing Laravel's rate limiting features, you can create more secure, fair, and efficient APIs. This not only protects your application from abuse but also ensures a better experience for all users by maintaining consistent performance and availability.