Securing Sensitive Actions with Password Confirmation in Laravel
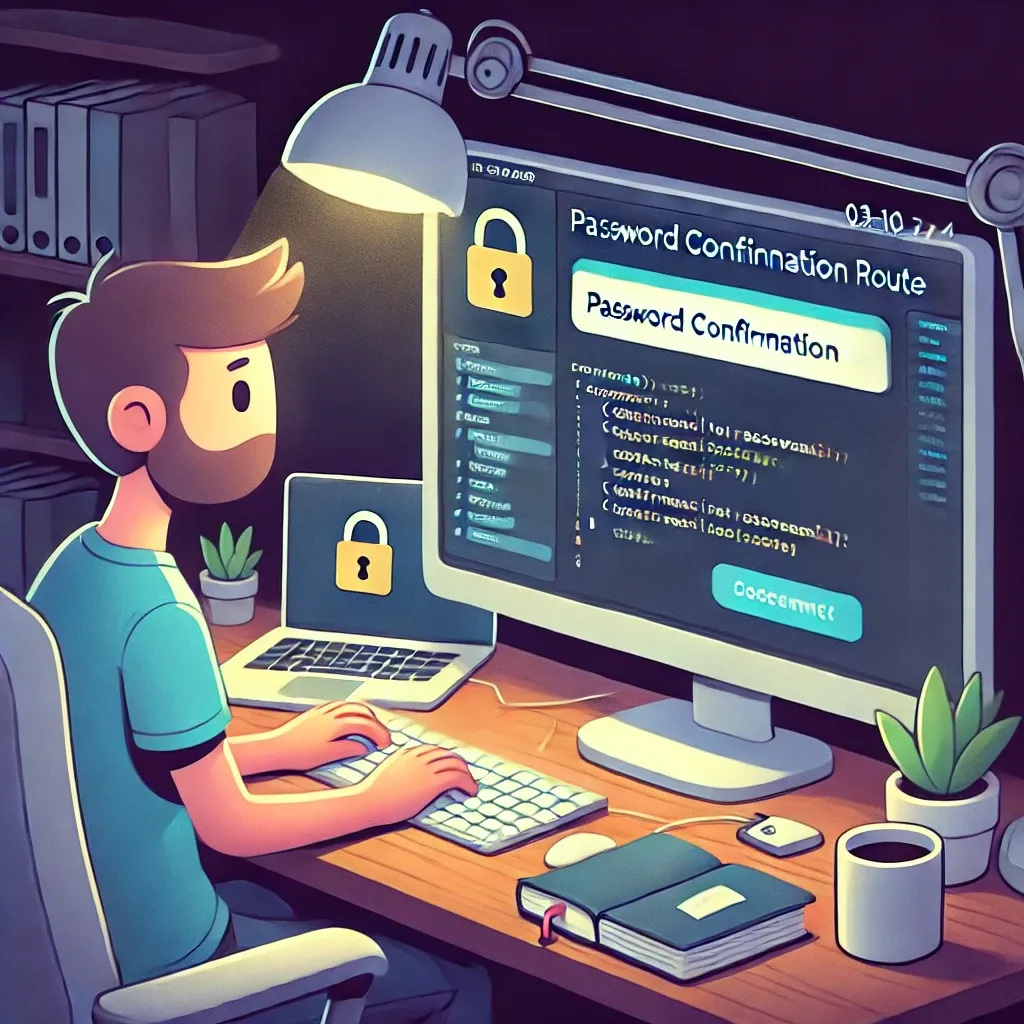
In any web application, certain actions require an extra layer of security. Laravel provides a elegant way to implement this through password confirmation. Let's explore how to set up a password confirmation route to validate user identity before crucial operations.
The Password Confirmation Concept
Password confirmation adds an extra security layer by requiring users to re-enter their password before performing sensitive actions. This ensures that even if a user leaves their account logged in, others can't perform critical operations without knowing the password.
Implementing the Password Confirmation Route
Here's how you can implement a password confirmation route in Laravel:
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Redirect;
Route::post('/confirm-password', function (Request $request) {
if (!Hash::check($request->password, $request->user()->password)) {
return back()->withErrors([
'password' => ['The provided password does not match our records.']
]);
}
$request->session()->passwordConfirmed();
return redirect()->intended();
})->middleware(['auth', 'throttle:6,1']);
Breaking Down the Implementation
• The route uses the POST method, typically triggered by a form submission.
• We check if the provided password matches the authenticated user's password using Hash::check()
.
• If the password is incorrect, we redirect back with an error message.
• If the password is correct, we call $request->session()->passwordConfirmed()
to mark the session as confirmed.
• Finally, we redirect the user to their intended destination.
• The route is protected by the auth
middleware to ensure only authenticated users can access it.
• We also apply throttle:6,1
middleware to prevent brute-force attempts.
Using the Password Confirmation
After setting up this route, you can protect sensitive routes or actions using the password.confirm
middleware:
Route::post('/sensitive-action', function () {
// Perform sensitive action
})->middleware(['auth', 'password.confirm']);
When a user tries to access this route, they'll be redirected to the password confirmation page if they haven't recently confirmed their password.
Real-World Example: Deleting Account
Let's consider a scenario where we want to confirm the user's password before allowing them to delete their account:
Route::delete('/account', function (Request $request) {
$request->user()->delete();
Auth::logout();
return redirect('/')->with('status', 'Your account has been deleted.');
})->middleware(['auth', 'password.confirm']);
In this example:
• The route is protected by both auth
and password.confirm
middleware.
• Users must confirm their password before accessing this route.
• After confirmation, the account is deleted and the user is logged out.
Customizing the Confirmation Window
By default, a successful password confirmation is valid for three hours. You can customize this in your config/auth.php
file:
'password_timeout' => 10800,
This value is in seconds, so 10800 equals three hours.
By implementing password confirmation, you add an important layer of security to your Laravel application. This feature is particularly useful for protecting actions like changing passwords, updating email addresses, or deleting accounts. It provides peace of mind for both you and your users, ensuring that sensitive operations are only performed by the rightful account owner.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!