Securing Routes with Policy-Based Authorization in Laravel
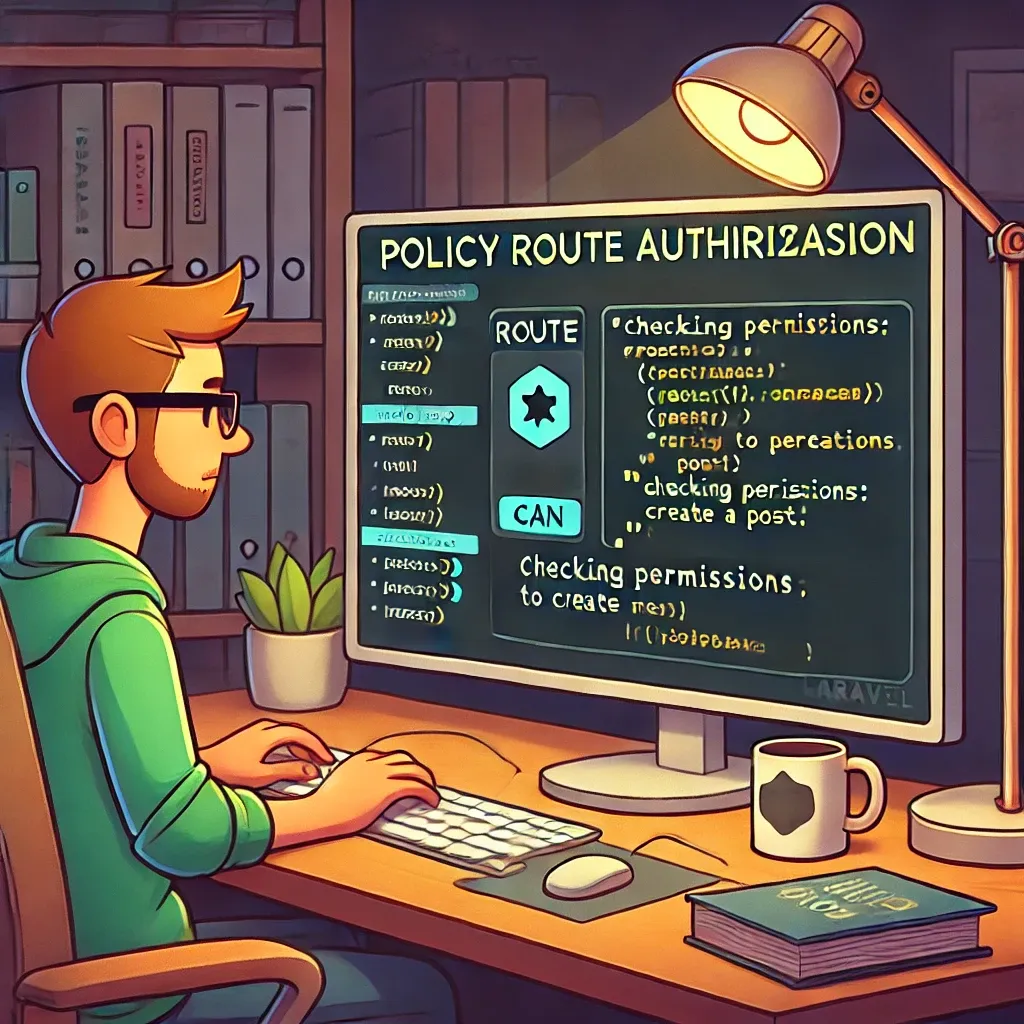
Laravel's policy-based authorization system provides a powerful way to manage access control in your application. When combined with route middleware, it offers a clean and efficient method to secure your routes based on user permissions. Let's explore how to implement policy-based route authorization using the 'can' middleware.
Understanding Policy-Based Route Authorization
Policy-based authorization allows you to define access rules in dedicated policy classes and then apply these rules to your routes. This approach promotes cleaner, more maintainable code by separating authorization logic from your controllers.
Using the 'can' Middleware
Laravel provides the 'can' middleware to easily apply policy-based authorization to your routes. Here's a basic example:
use App\Models\Post;
Route::post('/post', function () {
// Create post...
})->middleware('can:create,App\Models\Post');
In this example, the route is protected by the 'can' middleware, which checks if the authenticated user has permission to create a Post.
Breaking Down the Syntax
The 'can' middleware takes two parameters:
- The name of the policy method to check (e.g., 'create')
- The model class or instance the policy should be applied to
Using Route::can() for Cleaner Syntax
Laravel also offers a can()
method on the Route facade for a more fluent syntax:
use App\Models\Post;
Route::post('/post', function () {
// Create post...
})->can('create', Post::class);
This achieves the same result as the previous example but with a cleaner, more readable syntax.
Authorizing Actions on Specific Model Instances
When you need to authorize actions on a specific model instance, you can pass the instance directly:
Route::put('/post/{post}', function (Post $post) {
// Update the post...
})->can('update', 'post');
Here, Laravel will automatically resolve the 'post' parameter from the route and pass it to the policy.
Handling Authorization Failure
By default, when authorization fails, Laravel will generate a 403 HTTP response. You can customize this behavior in your App\Exceptions\Handler
class.
Real-World Examples
• Protecting a user profile update route:
Route::put('/user/{user}', [UserController::class, 'update'])
->can('update', 'user');
• Securing an admin dashboard:
Route::get('/admin/dashboard', [AdminController::class, 'index'])
->can('viewAdminDashboard', User::class);
• Managing article visibility:
Route::get('/articles/{article}', [ArticleController::class, 'show'])
->can('view', 'article');
Combining with Route Groups
You can apply the 'can' middleware to entire route groups for more efficient route definitions:
Route::middleware('can:manage-posts')->group(function () {
Route::get('/posts/create', [PostController::class, 'create']);
Route::post('/posts', [PostController::class, 'store']);
Route::get('/posts/{post}/edit', [PostController::class, 'edit']);
Route::put('/posts/{post}', [PostController::class, 'update']);
Route::delete('/posts/{post}', [PostController::class, 'destroy']);
});
This applies the 'manage-posts' policy check to all routes within the group.
By leveraging policy-based route authorization, you can create a robust, scalable authorization system for your Laravel application. This approach not only secures your routes but also promotes cleaner, more maintainable code by separating concerns and centralizing your authorization logic.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!