Securing File Uploads: Mastering File Type Validation in Laravel
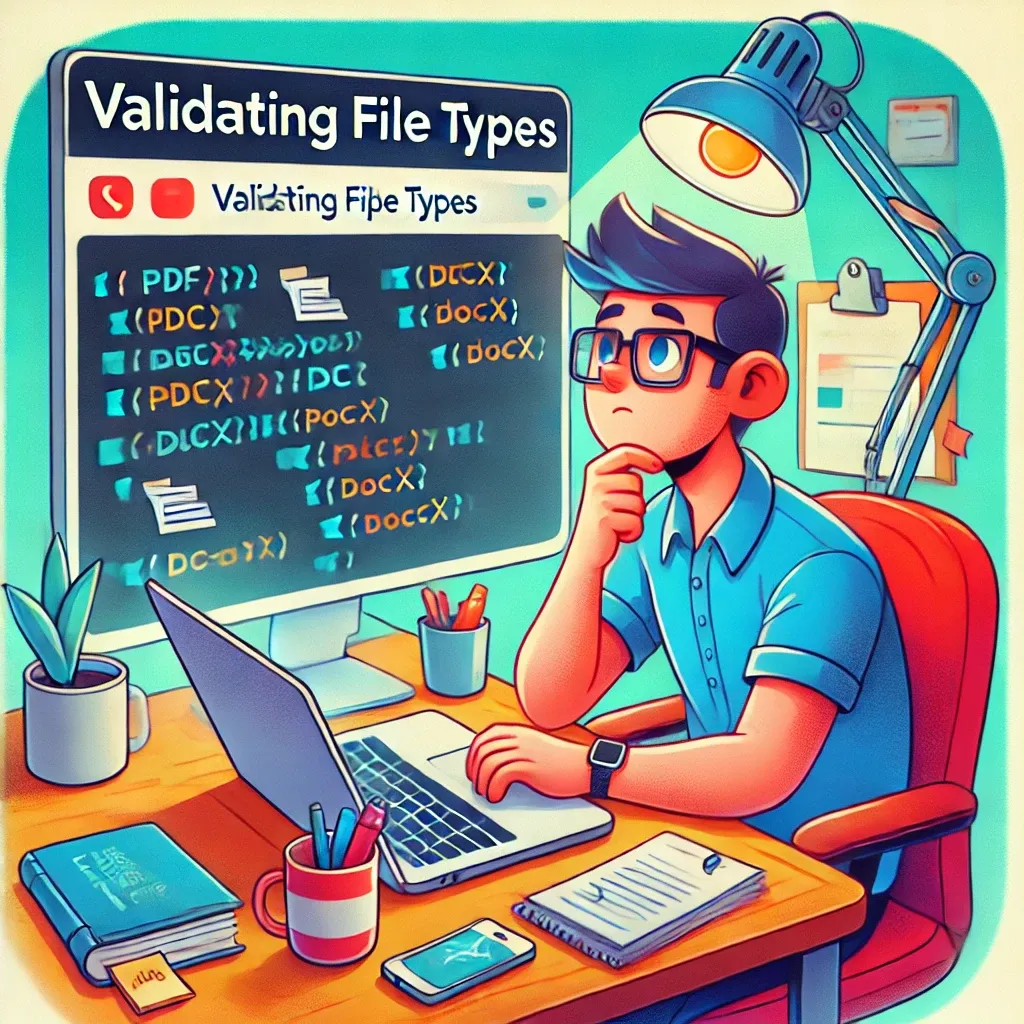
In web applications, file uploads are a common feature, but they can also be a significant security risk if not handled properly. Laravel provides robust tools for validating file uploads, including the ability to verify file types. Let's explore how to implement secure file type validation in your Laravel applications.
Understanding File Type Validation
Laravel offers two primary methods for validating file types:
- The
mimes
rule - The
mimetypes
rule
Both of these rules help ensure that uploaded files match expected types, but they work slightly differently.
Using the 'mimes' Rule
The mimes
rule validates files against a list of extension names:
$request->validate([
'document' => 'required|file|mimes:pdf,docx,txt|max:10240',
]);
In this example:
- The file must be present (
required
) - It must be a file upload (
file
) - It must have an extension of pdf, docx, or txt
- It must not exceed 10MB in size (10240 KB)
Using the 'mimetypes' Rule
The mimetypes
rule checks the file's MIME type:
$request->validate([
'image' => 'required|file|mimetypes:image/jpeg,image/png|max:5120',
]);
This rule ensures:
- The file is present
- It's a file upload
- It has a MIME type of either image/jpeg or image/png
- It doesn't exceed 5MB in size
Combining with Other Validation Rules
You can combine these rules with other Laravel validation rules for comprehensive file validation:
$request->validate([
'avatar' => [
'required',
'file',
'mimes:jpg,png',
'dimensions:min_width=100,min_height=100',
'max:2048',
],
]);
This example ensures the avatar is a jpg or png image, has minimum dimensions, and doesn't exceed 2MB.
Real-World Example: Document Management System
Let's consider a more complex scenario for a document management system:
public function store(Request $request)
{
$request->validate([
'document' => [
'required',
'file',
'mimes:pdf,doc,docx,txt,xls,xlsx',
'max:20480', // 20MB max
],
'document_type' => 'required|in:contract,report,presentation',
'description' => 'required|string|max:500',
]);
if ($request->document_type === 'presentation' && !in_array($request->file('document')->extension(), ['ppt', 'pptx'])) {
return back()->withErrors(['document' => 'Presentations must be PowerPoint files.']);
}
// Process and store the document
$path = $request->file('document')->store('documents');
Document::create([
'path' => $path,
'type' => $request->document_type,
'description' => $request->description,
'user_id' => auth()->id(),
]);
return redirect()->route('documents.index')->with('success', 'Document uploaded successfully.');
}
This example demonstrates:
- Basic file type and size validation
- Additional contextual validation based on document type
- Proper file storage and database recording
Validating file types is a crucial aspect of building secure Laravel applications that handle file uploads. By leveraging Laravel's built-in validation rules and combining them with custom logic, you can create robust and secure file handling systems. Always remember to validate on the server-side and never trust client-side validation alone. With these practices in place, you can confidently manage file uploads in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!