Secure Markdown Processing with Laravel's inlineMarkdown Method
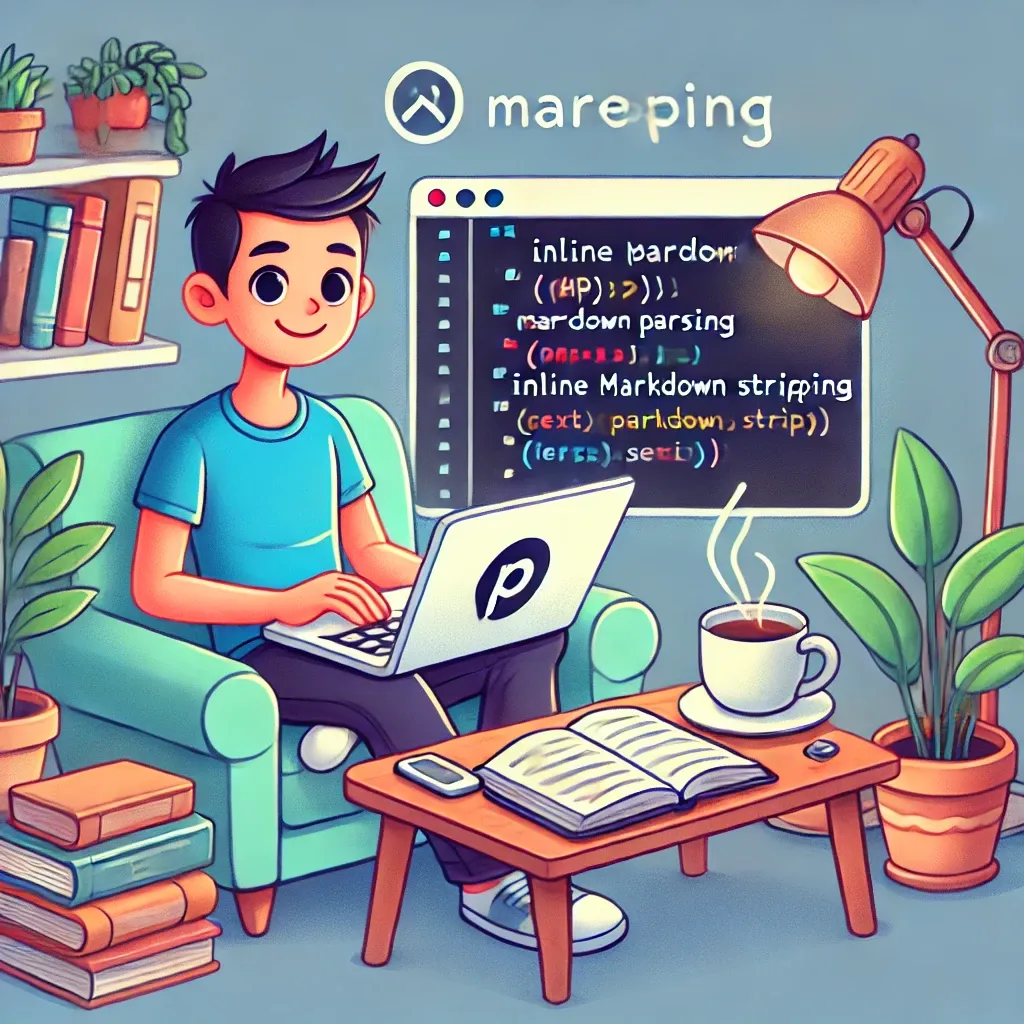
Laravel's string handling includes a powerful Markdown converter with the inlineMarkdown method, offering secure transformation of GitHub-flavored Markdown to inline HTML.
Basic Usage
Convert Markdown to inline HTML:
use Illuminate\Support\Str;
// Basic conversion
$html = Str::inlineMarkdown('**Laravel**');
// Result: <strong>Laravel</strong>
// Secure conversion with options
$html = Str::inlineMarkdown(
'Inject: <script>alert("Hello XSS!");</script>',
[
'html_input' => 'strip',
'allow_unsafe_links' => false,
]
);
// Result: Inject: alert("Hello XSS!");
Real-World Example
Here's how you might use it in a comment processing system:
class CommentProcessor
{
protected $markdownOptions = [
'html_input' => 'strip',
'allow_unsafe_links' => false
];
public function formatComment(string $content)
{
return Str::inlineMarkdown(
$content,
$this->markdownOptions
);
}
public function processUserMention(string $content)
{
// Convert @username to links while keeping other markdown
$processed = preg_replace(
'/@(\w+)/',
'[@$1](/users/$1)',
$content
);
return Str::inlineMarkdown(
$processed,
$this->markdownOptions
);
}
public function formatNotification(string $template, array $vars)
{
$content = strtr($template, $vars);
return Str::inlineMarkdown(
$content,
[
'html_input' => 'escape',
'allow_unsafe_links' => false
]
);
}
}
// Usage
$processor = new CommentProcessor();
$comment = $processor->formatComment('**Important** update!');
$mention = $processor->processUserMention('Hey @john, check this!');
The inlineMarkdown method ensures secure Markdown processing while maintaining clean, inline HTML output.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!