Resetting Rate Limits in Laravel: Using the clear Method
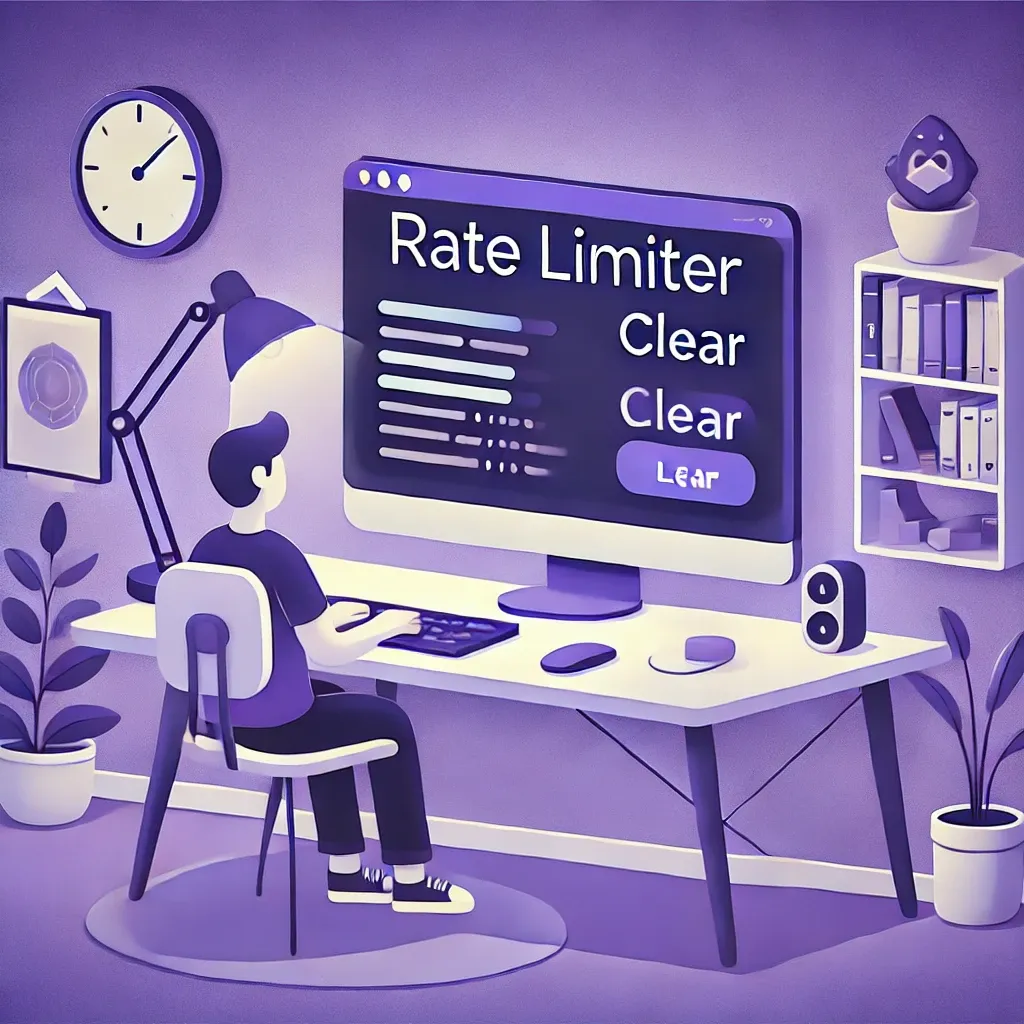
Learn to dynamically reset rate limits when certain conditions are met in your Laravel application. Let's explore how to use the clear
method to manage rate limiting flexibly.
Basic Usage
Here's how to clear a rate limit:
use Illuminate\Support\Facades\RateLimiter;
RateLimiter::clear('send-message:' . $userId);
Real-World Example
Here's a practical implementation for a messaging system:
class MessageController extends Controller
{
public function send(Request $request, User $recipient)
{
$key = 'send-message:' . auth()->id();
$limiter = RateLimiter::attempt(
$key,
$perMinute = 5,
function() use ($request, $recipient) {
// Send message logic here
return Message::create([
'sender_id' => auth()->id(),
'recipient_id' => $recipient->id,
'content' => $request->content
]);
}
);
if (!$limiter) {
return response()->json([
'error' => 'Too many messages sent. Please wait.'
], 429);
}
return response()->json(['status' => 'Message sent']);
}
public function markAsRead(Message $message)
{
$this->authorize('read', $message);
$message->markAsRead();
// Reset rate limit for sender when recipient reads the message
RateLimiter::clear('send-message:' . $message->sender_id);
return response()->json([
'status' => 'Message marked as read',
'can_reply' => true
]);
}
public function clearUserLimits(User $user)
{
// Clear various rate limits for the user
RateLimiter::clear('send-message:' . $user->id);
RateLimiter::clear('create-group:' . $user->id);
RateLimiter::clear('upload-media:' . $user->id);
return back()->with('success', 'Rate limits cleared');
}
}
The clear
method provides a way to programmatically reset rate limits when certain conditions are met, making your rate limiting system more dynamic and user-friendly.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!