Replace String Prefixes with Laravel's replaceStart Method
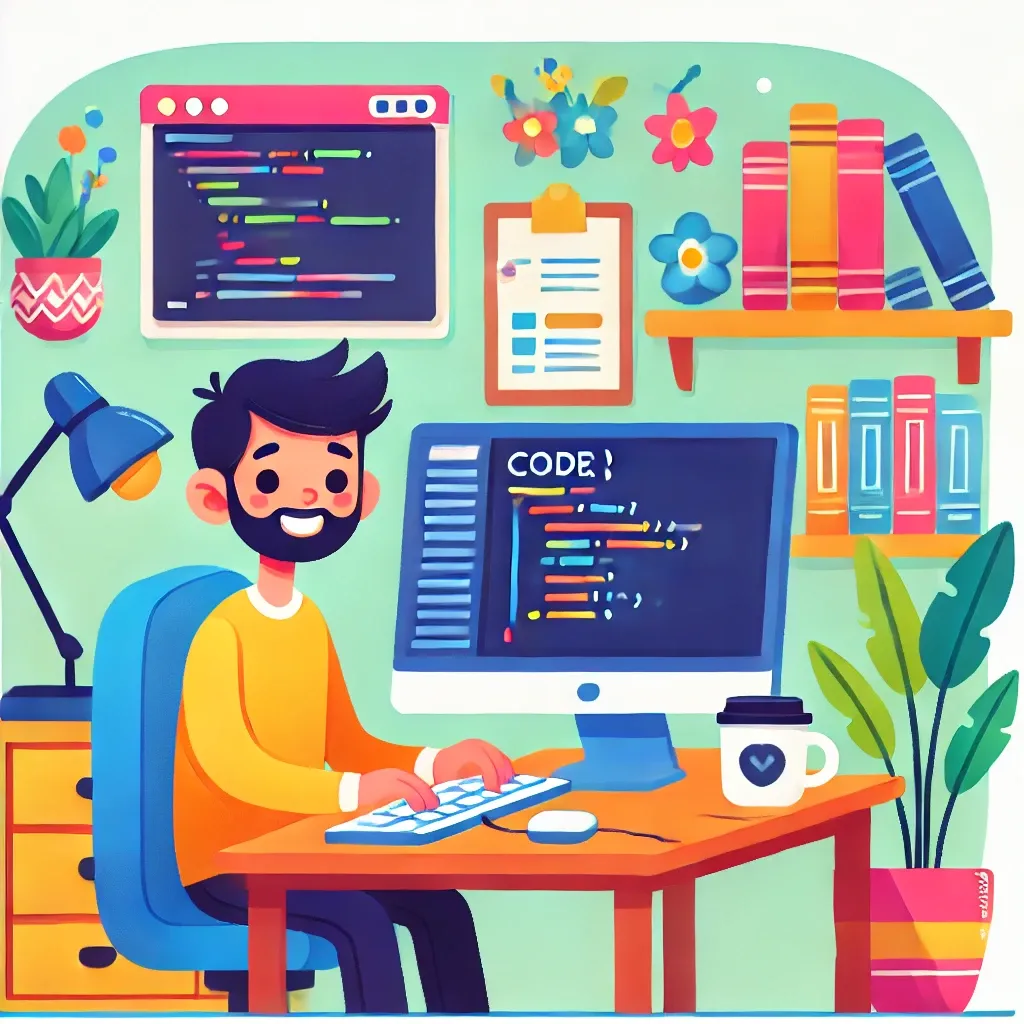
Need to replace text at the beginning of a string? Laravel's Str::replaceStart method offers a precise way to modify strings only when they start with specific content.
Basic Usage
Replace text at the start of a string:
use Illuminate\Support\Str;
// Successful replacement
$replaced = Str::replaceStart('Hello', 'Laravel', 'Hello World');
// Result: 'Laravel World'
// No replacement (doesn't start with 'World')
$replaced = Str::replaceStart('World', 'Laravel', 'Hello World');
// Result: 'Hello World'
Real-World Example
Here's how you might use it in a URL formatter:
class UrlFormatter
{
public function normalizeProtocol(string $url, string $protocol = 'https')
{
// Replace http:// with https://
$url = Str::replaceStart('http://', $protocol . '://', $url);
// Add protocol if missing
if (!str_starts_with($url, $protocol . '://')) {
$url = $protocol . '://' . $url;
}
return $url;
}
public function standardizeDomain(string $url, string $mainDomain)
{
// Replace www with main domain
$url = Str::replaceStart('www.', $mainDomain . '.', $url);
// Replace dev with main domain
$url = Str::replaceStart('dev.', $mainDomain . '.', $url);
return $url;
}
public function cleanupApiEndpoint(string $endpoint)
{
// Remove leading /api if present
return Str::replaceStart('/api', '', $endpoint);
}
}
// Usage
$formatter = new UrlFormatter();
echo $formatter->normalizeProtocol('http://example.com');
// Output: 'https://example.com'
echo $formatter->standardizeDomain('www.example.com', 'app');
// Output: 'app.example.com'
echo $formatter->cleanupApiEndpoint('/api/users');
// Output: '/users'
The replaceStart method provides precise control over string modifications when you need to target content at the beginning of a string.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!