Refreshing Your Data: Laravel's fresh Method for Collections
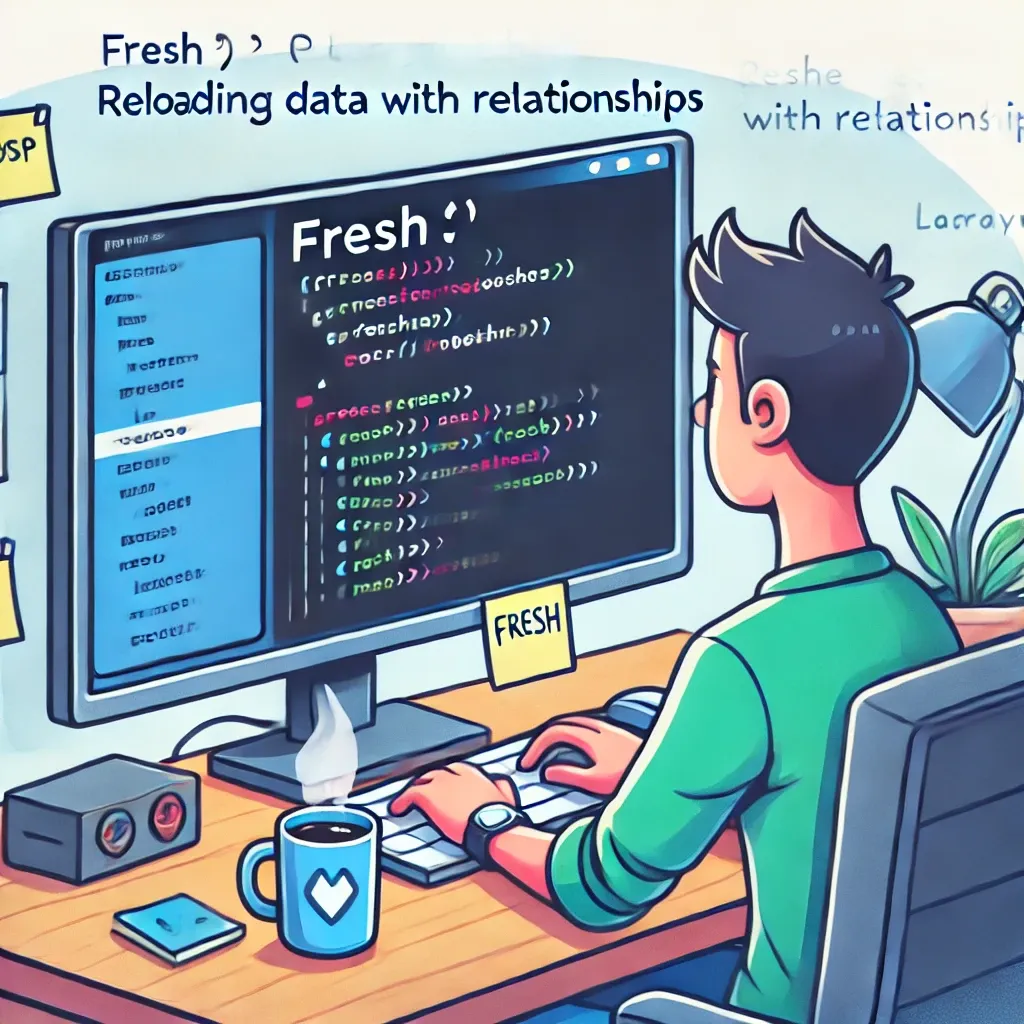
In the world of web development, data can change rapidly. Sometimes, the data you've retrieved from your database might become stale even while your application is processing it. Laravel provides an elegant solution to this problem with the fresh
method for collections. Let's dive into how this method can keep your data up-to-date and your application running smoothly.
Understanding the fresh Method
The fresh
method allows you to retrieve a new instance of each model in a collection from the database. This is particularly useful when you need to ensure you're working with the most current data, especially after time-consuming operations.
Basic Usage
Here's a simple example of how to use fresh
:
$users = User::all();
// Some time passes or operations occur...
$freshUsers = $users->fresh();
Now, $freshUsers
contains updated instances of all the users, reflecting any changes that might have occurred in the database since the initial query.
Refreshing with Relationships
One of the most powerful features of fresh
is its ability to load (or reload) relationships:
$users = User::all();
$freshUsersWithPosts = $users->fresh('posts');
This not only refreshes the user data but also loads (or reloads) the 'posts' relationship for each user.
Practical Applications
1. Ensuring Data Integrity in Long-Running Processes
Imagine you're processing a large number of orders:
$orders = Order::where('status', 'pending')->get();
foreach ($orders as $order) {
// Time-consuming process...
processOrder($order);
// Refresh the order to get the latest status
$freshOrder = $order->fresh();
if ($freshOrder->status !== 'pending') {
// Handle the case where the order status has changed
continue;
}
$freshOrder->status = 'processed';
$freshOrder->save();
}
2. Updating User Interfaces
In a web application, you might need to show updated information after a user action:
public function completeTask($taskId)
{
$task = Task::findOrFail($taskId);
$task->complete();
// Refresh the user to get updated task counts
$user = auth()->user()->fresh('tasks');
return response()->json([
'message' => 'Task completed successfully',
'completedTasksCount' => $user->tasks->where('completed', true)->count(),
'pendingTasksCount' => $user->tasks->where('completed', false)->count(),
]);
}
3. Ensuring Consistent Data in Background Jobs
When working with queued jobs, you might need to ensure you have the latest data:
class ProcessUserData implements ShouldQueue
{
public function handle()
{
$users = User::all();
// Some time might pass before the job is actually processed...
$freshUsers = $users->fresh('profile', 'settings');
foreach ($freshUsers as $user) {
// Process user data with the latest information
processUserData($user);
}
}
}
Real-World Scenario: E-commerce Inventory Management
Imagine you're building an e-commerce platform where inventory changes rapidly. You might use fresh
in a product reservation system:
public function reserveProducts(array $productIds, $quantity)
{
$products = Product::findMany($productIds);
// Simulate some time passing (e.g., user filling out shipping info)
sleep(5);
$freshProducts = $products->fresh();
foreach ($freshProducts as $product) {
if ($product->stock < $quantity) {
throw new InsufficientStockException("Not enough stock for product: {$product->name}");
}
$product->stock -= $quantity;
$product->save();
}
// Proceed with order creation...
}
In this example, we ensure we're working with the most up-to-date stock information before finalizing the reservation, preventing overselling.
The fresh
method in Laravel provides a powerful way to ensure your application is always working with the most current data. By allowing you to easily refresh model instances and their relationships, it helps maintain data integrity and consistency across your application, especially in scenarios involving long-running processes or rapidly changing data.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!