Quick Command Options in Laravel: Mastering Shortcuts
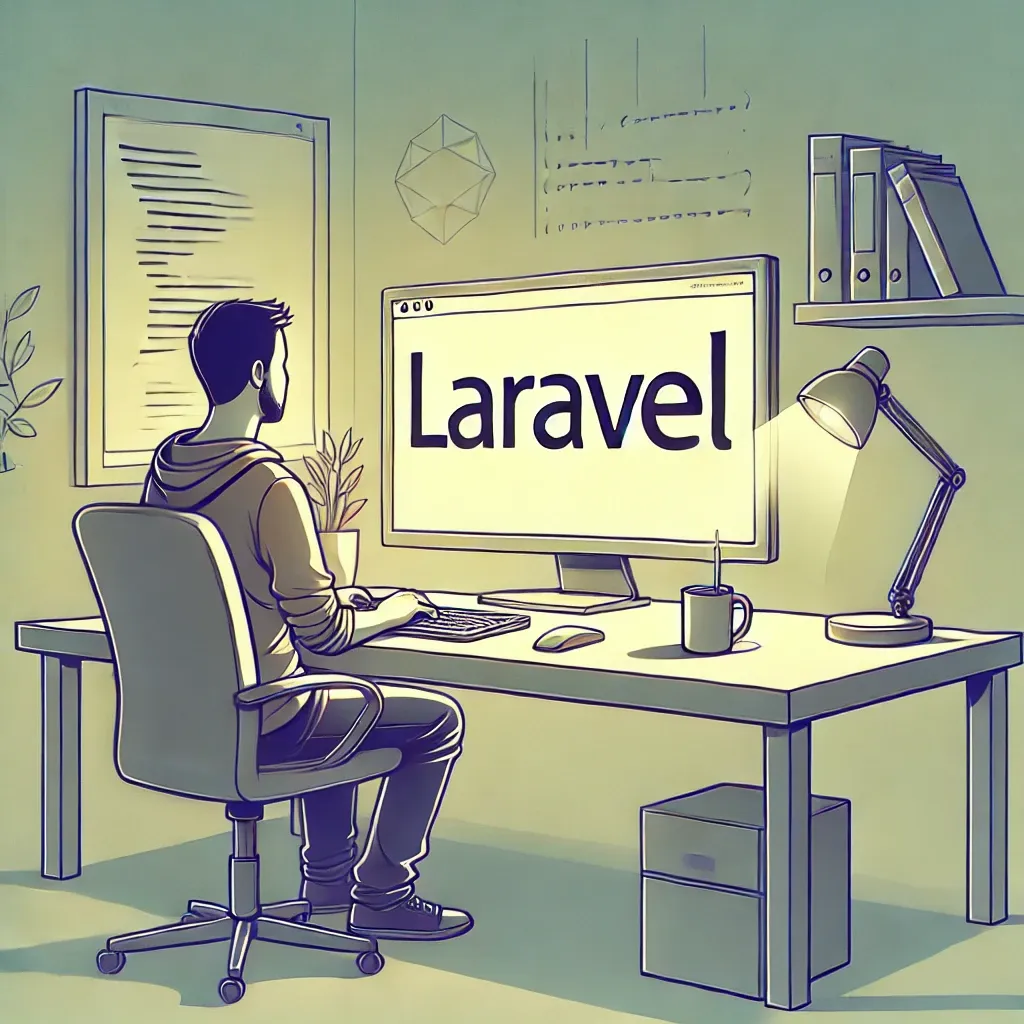
Want to make your Artisan commands more user-friendly? Laravel's option shortcuts let you create intuitive, quick-to-type command options! Let's explore this handy feature.
Defining Shortcuts
Here's how to add a shortcut to your option:
protected $signature = 'mail:send {user} {--Q|queue}';
The syntax is simple:
- Use the
|
character as a delimiter - Put the shortcut before the full option name
- Single letter shortcuts work best
Using Shortcuts
The command can now be used in two ways:
# Full option
php artisan mail:send 1 --queue=default
# With shortcut
php artisan mail:send 1 -Qdefault
Real-World Example
Here's a practical deployment command with shortcuts:
class DeployApplication extends Command
{
protected $signature = 'deploy
{env? : Environment to deploy to}
{--F|force : Skip confirmation}
{--B|backup : Create backup before deploying}
{--C|config= : Custom config file path}';
public function handle()
{
$environment = $this->argument('env') ?? 'production';
if (!$this->option('force')) {
if (!$this->confirm("Deploy to {$environment}?")) {
return;
}
}
if ($this->option('backup')) {
$this->info('Creating backup...');
}
$configPath = $this->option('config');
$this->info("Deploying to {$environment}...");
// Deployment logic here
}
}
Usage examples:
# Full options
php artisan deploy staging --force --backup --config=custom.php
# With shortcuts
php artisan deploy staging -F -B -Ccustom.php
Shortcuts make your commands faster to type while keeping them intuitive and user-friendly.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!