Prevent Stray HTTP Requests in Laravel Tests: Using preventStrayRequests
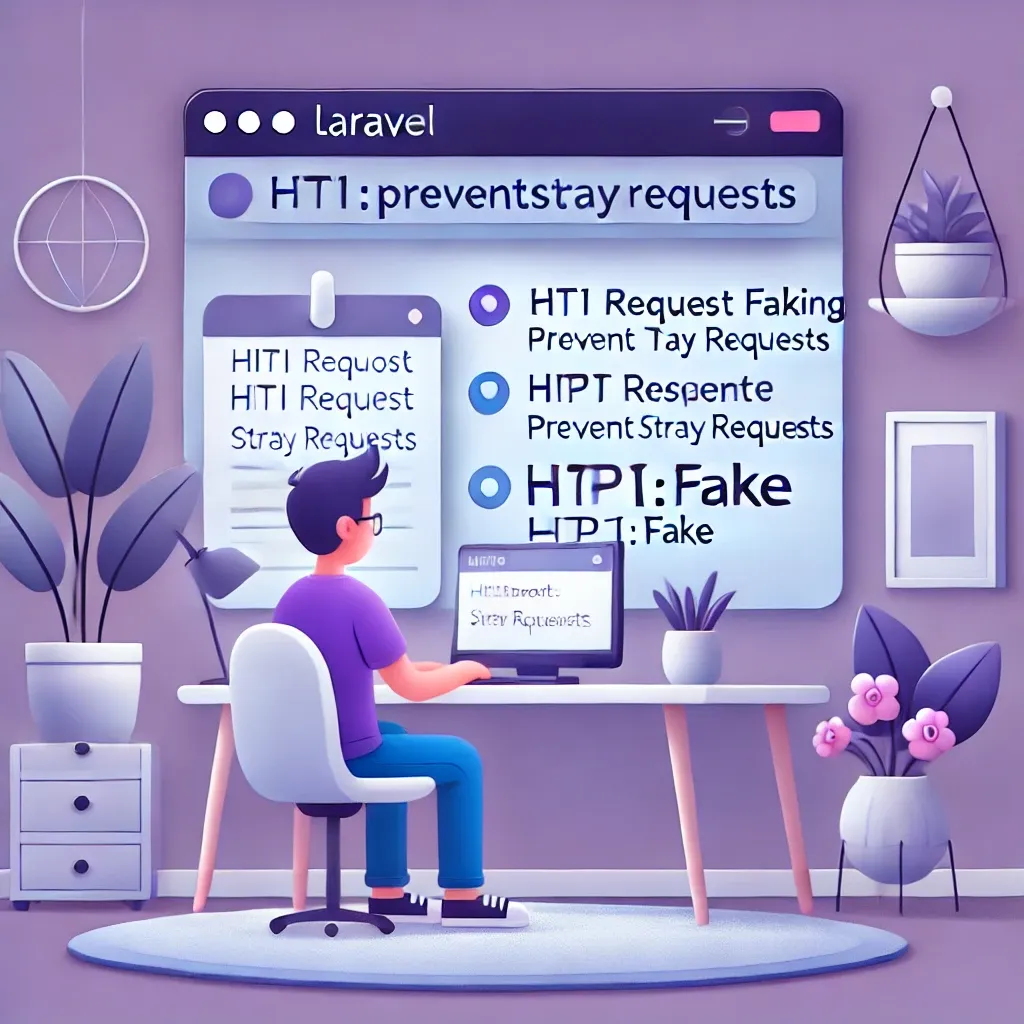
Ever wanted to ensure that all HTTP requests in your Laravel tests are properly faked using the preventStrayRequests method? This powerful testing feature helps catch unintended external requests and maintain reliable tests.
Basic Usage
Here's how to prevent unwanted HTTP requests in your tests:
use Illuminate\Support\Facades\Http;
Http::preventStrayRequests();
Http::fake([
'github.com/*' => Http::response('ok')
]);
Real-World Example
Here's how to implement comprehensive HTTP request testing:
class GitHubServiceTest extends TestCase
{
protected function setUp(): void
{
parent::setUp();
// Prevent any unfaked requests
Http::preventStrayRequests();
// Set up common fake responses
Http::fake([
'api.github.com/repos/*' => Http::response([
'id' => 1,
'name' => 'laravel',
'stars' => 1000
]),
'api.github.com/user/starred/*' => Http::sequence()
->push(['starred' => true], 200)
->push(['starred' => false], 200),
// Catch-all pattern should be last
'*' => Http::response('Unexpected request', 500)
]);
}
public function test_fetches_repository_information()
{
$service = new GitHubService();
$repo = $service->getRepositoryInfo('laravel/framework');
$this->assertEquals('laravel', $repo['name']);
// This would throw an exception because the URL isn't faked
// $service->getPackagistStats('laravel/framework');
}
public function test_checks_star_status()
{
$service = new GitHubService();
$this->assertTrue($service->hasUserStarred('laravel/framework'));
$this->assertFalse($service->hasUserStarred('laravel/framework'));
}
}
Using preventStrayRequests
ensures your tests catch any unintended external HTTP requests, making your test suite more reliable and predictable.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!