Precision Data Extraction with Laravel's takeWhile Method
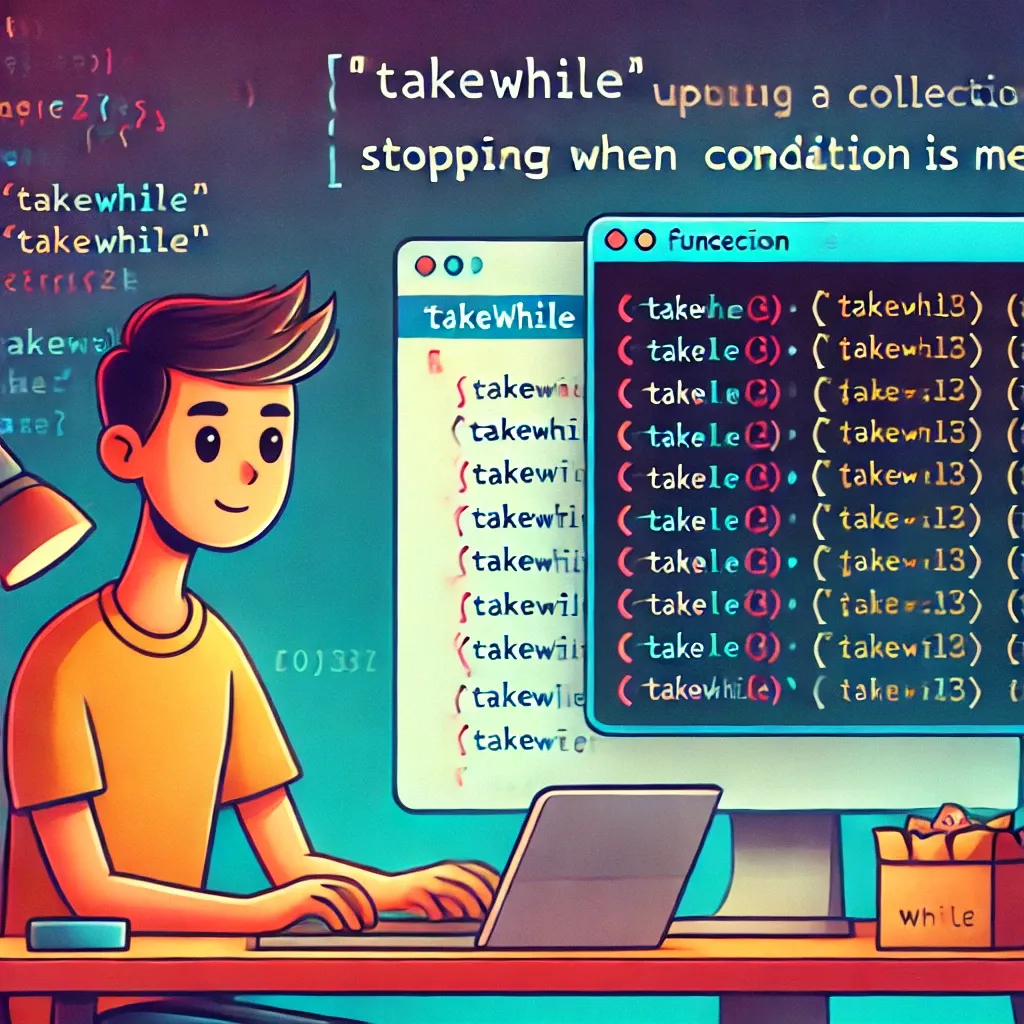
Laravel's Collection class continues to impress with its array of powerful methods for data manipulation. The takeWhile
method stands out as a precise tool for extracting portions of collections based on specific conditions. Let's explore how this method works and how it can enhance your Laravel applications.
Understanding takeWhile
The takeWhile
method allows you to extract items from the beginning of a collection until a given condition evaluates to false. Once an item fails to meet the condition, the method stops and returns the collected items up to that point.
Basic Usage
Here's a simple example to illustrate how takeWhile
works:
$collection = collect([1, 2, 3, 4, 5, 1, 2, 3]);
$subset = $collection->takeWhile(function ($item) {
return $item < 4;
});
// Result: [1, 2, 3]
In this example, takeWhile
takes items less than 4. It stops as soon as it encounters 4, excluding it and all subsequent items from the result.
Practical Applications
The takeWhile
method shines in scenarios where you need to extract a specific segment of data based on a condition. Here are some practical applications:
Processing Time Series Data
Imagine you're analyzing stock prices and want to capture the initial uptrend:
$stockPrices = collect([100, 101, 103, 105, 104, 103, 105, 107]);
$uptrend = $stockPrices->takeWhile(function ($price, $key) use ($stockPrices) {
if ($key === 0) return true;
return $price > $stockPrices[$key - 1];
});
// Result: [100, 101, 103, 105]
Extracting Valid Data
When working with user inputs, you might want to collect valid entries until an invalid one is encountered:
$userInputs = collect(['John', 'Jane', 'Bob', '', 'Alice', 'Charlie']);
$validInputs = $userInputs->takeWhile(function ($input) {
return !empty($input);
});
// Result: ['John', 'Jane', 'Bob']
Pagination Control
In a pagination scenario, you might want to show links until the current page:
$pages = collect(range(1, 10));
$currentPage = 5;
$pageLinks = $pages->takeWhile(function ($page) use ($currentPage) {
return $page <= $currentPage;
});
// Result: [1, 2, 3, 4, 5]
Combining with Other Collection Methods
takeWhile
becomes even more powerful when combined with other collection methods. For instance, you can use it with skipWhile
to extract a specific section of a collection:
$temperatures = collect([15, 16, 18, 21, 23, 25, 24, 22, 20, 18]);
$summerDays = $temperatures->skipWhile(function ($temp) {
return $temp < 20;
})->takeWhile(function ($temp) {
return $temp >= 20;
});
// Result: [21, 23, 25, 24, 22, 20]
This combination allows you to extract a range of items that meet certain criteria, which can be incredibly useful for data analysis or processing specific data segments.
Edge Cases and Considerations
It's important to note that if the condition is never false, takeWhile
will return all items in the collection. Also, if the first item doesn't satisfy the condition, you'll get an empty collection.
$allPositive = collect([1, 2, 3, 4, 5])->takeWhile(fn($n) => $n > 0);
// Result: [1, 2, 3, 4, 5]
$noNegative = collect([1, 2, 3, 4, 5])->takeWhile(fn($n) => $n < 0);
// Result: []
The takeWhile
method in Laravel's Collection class offers a precise and elegant way to extract portions of data based on specific conditions. Whether you're processing time series data, handling user inputs, or implementing custom pagination logic, takeWhile
provides a clean and expressive solution for targeted data extraction in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!