Precise Validation Testing with Laravel's assertOnlyJsonValidationErrors Method
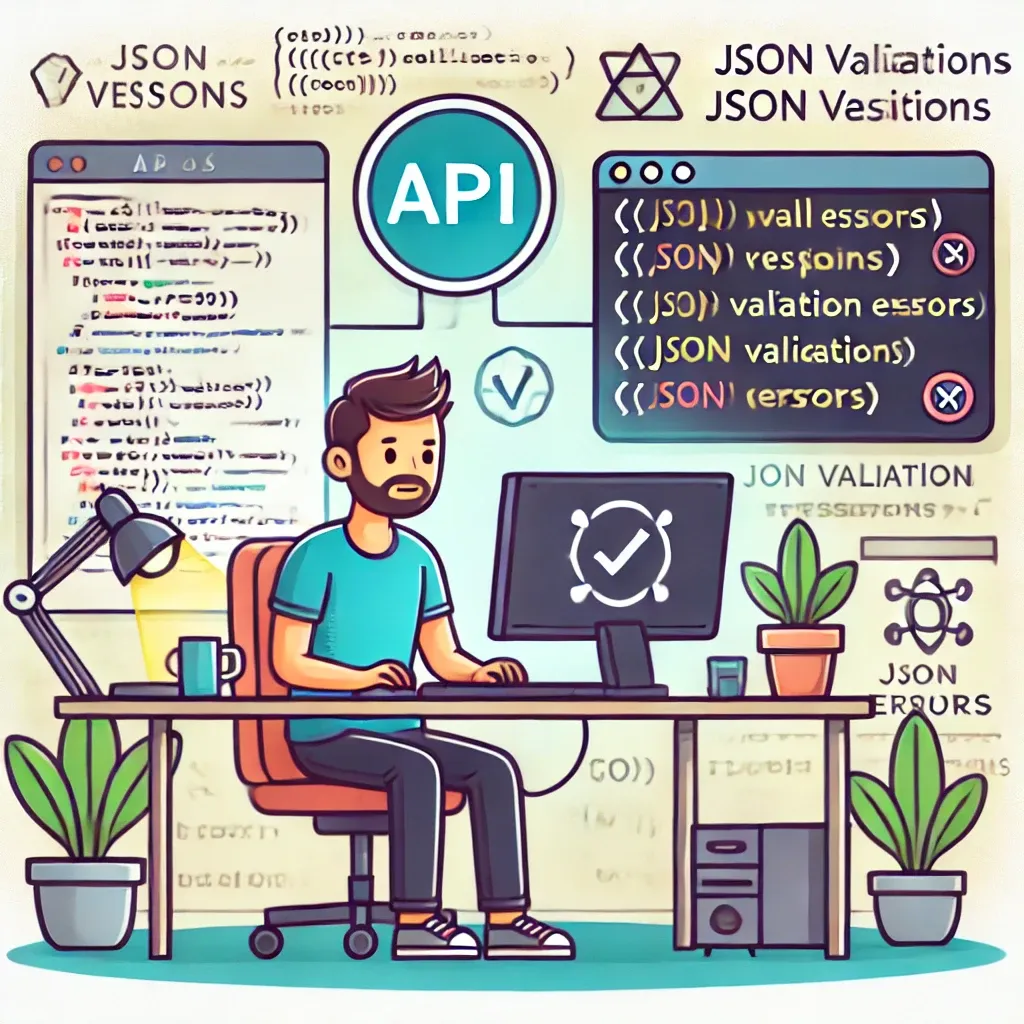
Testing validation errors in Laravel can be surprisingly tricky. The new assertOnlyJsonValidationErrors method provides a more precise way to verify exactly which validation errors occurred.
When testing API endpoints or form submissions, it's often crucial to verify not just that specific validation errors are present, but also that no unexpected errors occurred. Until now, achieving this level of precision required multiple assertions and wasn't always straightforward.
Let's look at the existing approach:
$this->post('/user')
->assertJsonValidationErrors(['email'])
->assertJsonMissingValidationErrors(['name']);
This code checks that an 'email' validation error is present and that a 'name' validation error is not present. However, it has a significant limitation: it won't fail if unexpected validation errors occur, such as a 'phone' error. This makes it difficult to ensure your validation logic is working exactly as expected.
The New Solution
Laravel now provides a more precise method for validation testing:
$this->post('/user')->assertOnlyJsonValidationErrors(['email']);
This single assertion verifies three important things:
- The 'email' validation error is present
- No 'name' validation error is present
- No other unexpected validation errors occurred
Real-World Example
Let's see how this improves testing in a practical scenario:
class UserControllerTest extends TestCase
{
/** @test */
public function email_is_required_for_registration()
{
// Old approach - incomplete verification
$this->postJson('/api/register', [
'name' => 'John Doe',
'password' => 'password',
'password_confirmation' => 'password'
])
->assertStatus(422)
->assertJsonValidationErrors(['email']);
// New approach - complete verification
$this->postJson('/api/register', [
'name' => 'John Doe',
'password' => 'password',
'password_confirmation' => 'password'
])
->assertStatus(422)
->assertOnlyJsonValidationErrors(['email']);
}
/** @test */
public function multiple_fields_can_fail_validation()
{
$this->postJson('/api/register', [
'name' => '',
'password' => 'short'
])
->assertStatus(422)
->assertOnlyJsonValidationErrors([
'name',
'email',
'password'
]);
}
}
This method helps catch subtle validation bugs that might otherwise go unnoticed, such as validation rules that trigger unexpectedly or don't trigger when they should.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!