Precise Collection Filtering with Laravel's whereNotInStrict
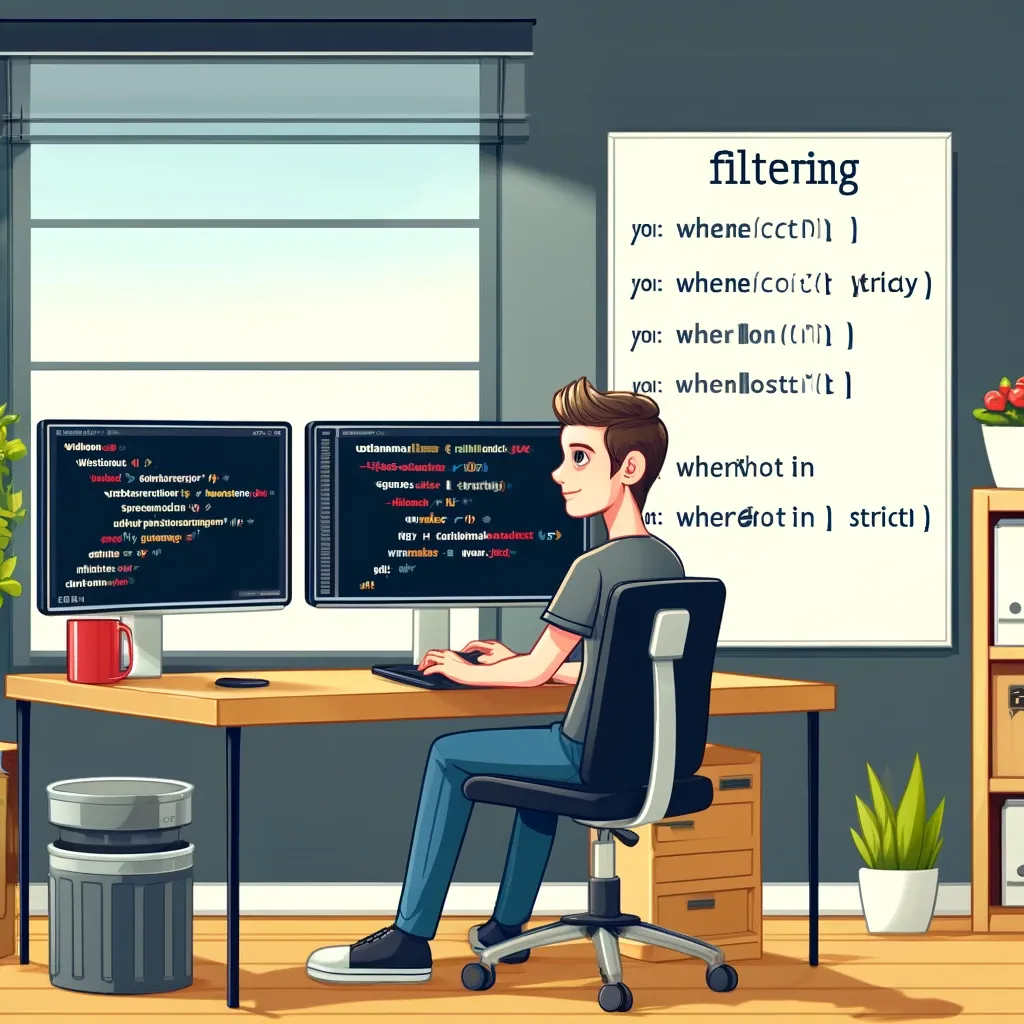
Need precise filtering in your collections? Laravel's whereNotInStrict method provides strict comparison filtering, ensuring exact type and value matches when excluding items.
Basic Usage
Filter collections with strict type checking:
$collection = collect([
['id' => 1, 'value' => '100'],
['id' => 2, 'value' => 100],
['id' => 3, 'value' => '200'],
['id' => 4, 'value' => 200]
]);
// Regular filtering (loose comparison)
$loose = $collection->whereNotIn('value', [100]);
// Result: Only items with value '200' and 200
// Strict filtering (type-sensitive)
$strict = $collection->whereNotInStrict('value', [100]);
// Result: Items with value '100', '200', and 200
// (keeps string '100' because 100 !== '100')
Real-World Example
Here's how you might use it in a product filtering system:
class ProductFilter
{
public function filterByExactStock($products, array $excludedStockLevels)
{
// Strict filtering ensures exact stock level matches
return $products->whereNotInStrict('stock_level', $excludedStockLevels);
}
public function filterByPreciseStatus($products, array $statuses)
{
return $products
->whereNotInStrict('status', $statuses)
->values();
}
public function applyFilters($products)
{
return $products
// Exclude specific numeric codes (type-sensitive)
->whereNotInStrict('product_code', ['001', '002'])
// Exclude specific string statuses (type-sensitive)
->whereNotInStrict('status', ['active', 'pending'])
// Re-index array
->values();
}
}
// Usage
$products = collect([
['product_code' => '001', 'status' => 'active'],
['product_code' => 1, 'status' => 'active'], // Different type
['product_code' => '002', 'status' => 'pending'],
['product_code' => 2, 'status' => 'inactive']
]);
$filter = new ProductFilter();
$filtered = $filter->applyFilters($products);
// Keeps items where product_code is numeric
// and status is not exactly 'active' or 'pending'
The whereNotInStrict method ensures type-sensitive filtering when you need precise control over your collection contents.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!