Organizing Collections with Laravel's splitIn Method
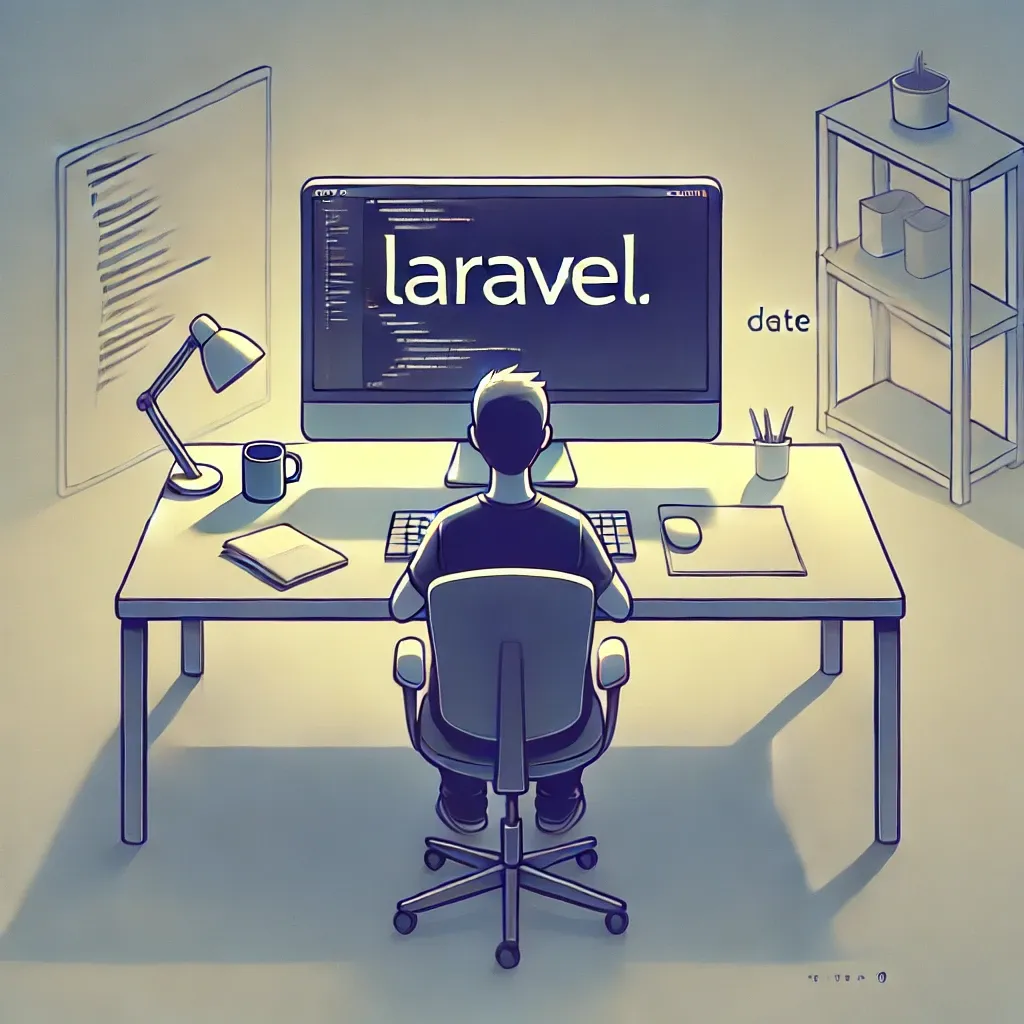
Need to split your collection into equal-sized groups? Laravel's new splitIn
method makes it a breeze! Let's explore how this handy method helps you organize your data.
Basic Usage
Here's how simple it is:
$collection = collect([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]);
$groups = $collection->splitIn(3);
// Result: [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10]]
Real-World Example
Here's how you might use it in a task distribution system:
class TaskDistributor
{
public function distributeToTeams(Collection $tasks, int $teamCount)
{
$distributedTasks = $tasks->splitIn($teamCount);
return $distributedTasks->map(function ($teamTasks, $index) {
return [
'team_number' => $index + 1,
'task_count' => count($teamTasks),
'tasks' => $teamTasks->map(fn($task) => [
'id' => $task->id,
'priority' => $task->priority,
'deadline' => $task->deadline->format('Y-m-d')
])
];
});
}
}
// Usage
$tasks = Task::where('status', 'pending')->get();
$distributor = new TaskDistributor();
$teamAssignments = $distributor->distributeToTeams($tasks, 3);
foreach ($teamAssignments as $assignment) {
$this->info("Team {$assignment['team_number']} received " .
"{$assignment['task_count']} tasks");
}
The splitIn
method ensures that groups are filled evenly, with any remainder going to the last group. It's perfect for distributing workloads, paginating content, or any scenario where you need balanced groups.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!