Optimizing View Data Loading with Laravel View Creators
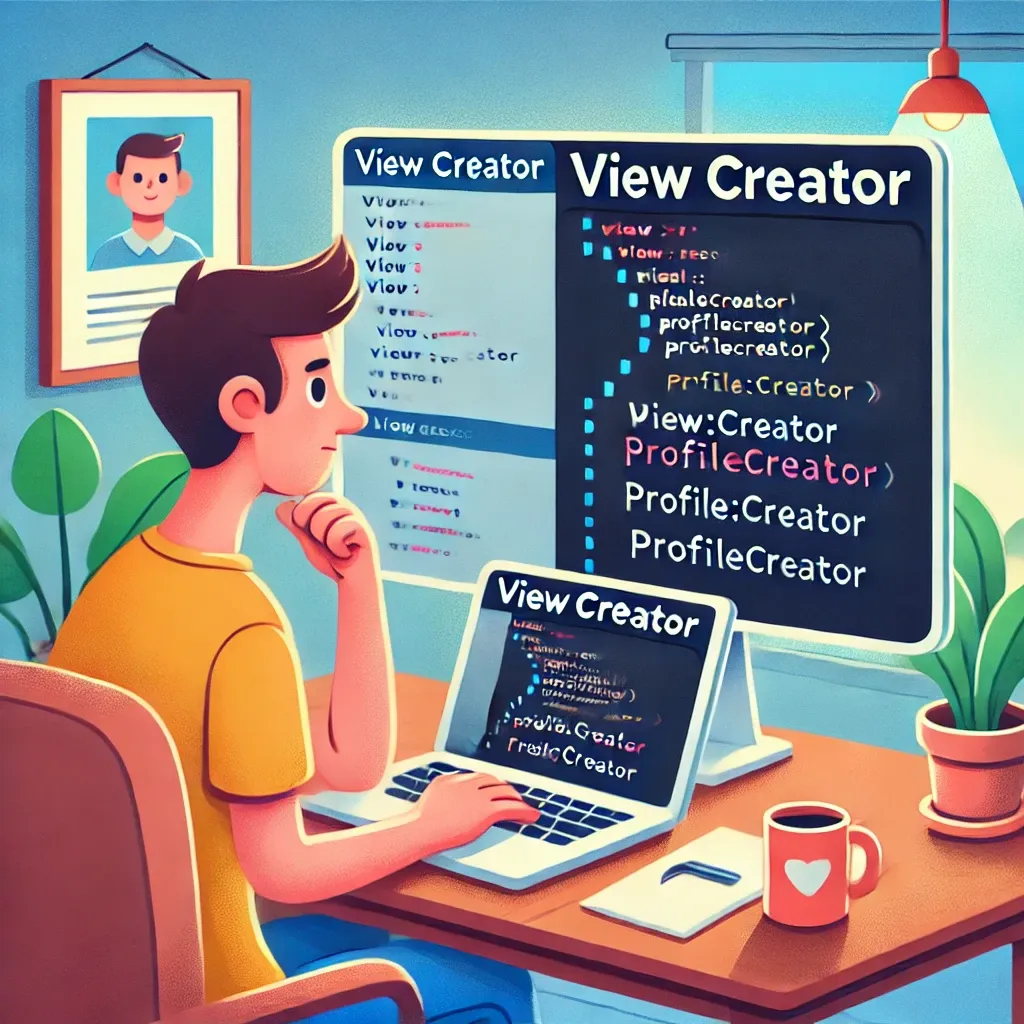
While Laravel's View Composers are a powerful tool for injecting data into views, sometimes you need to prepare data even earlier in the view rendering process. This is where View Creators come into play. Let's explore how View Creators can help you optimize your Laravel application's view rendering process.
Understanding View Creators
View Creators are very similar to View Composers, but with one key difference: they are executed immediately after the view is instantiated, rather than just before the view is rendered. This can be particularly useful for setting up initial view data or for performance optimization in certain scenarios.
Basic Usage of View Creators
To register a view creator, you use the creator
method provided by the View
facade. Typically, you'd do this in a service provider:
use Illuminate\Support\Facades\View;
use App\View\Creators\ProfileCreator;
public function boot()
{
View::creator('profile', ProfileCreator::class);
}
Now, let's create the ProfileCreator
class:
namespace App\View\Creators;
use App\Repositories\UserRepository;
use Illuminate\View\View;
class ProfileCreator
{
protected $users;
public function __construct(UserRepository $users)
{
$this->users = $users;
}
public function create(View $view)
{
$view->with('userCount', $this->users->count());
}
}
The create
method will be called as soon as the 'profile' view is instantiated, allowing you to set up initial data for the view.
When to Use View Creators
View Creators are particularly useful in scenarios where:
- You need to set up data that other view composers might depend on.
- You want to optimize performance by loading data as early as possible in the view lifecycle.
- You're working with views that are conditionally rendered and want to ensure data is ready regardless of the rendering condition.
Real-World Example: Dynamic Navigation Menu
Let's consider a more complex example where we want to create a dynamic navigation menu that depends on the user's role:
// app/View/Creators/NavigationCreator.php
namespace App\View\Creators;
use Illuminate\View\View;
use App\Services\NavigationService;
use Illuminate\Support\Facades\Auth;
class NavigationCreator
{
protected $navigationService;
public function __construct(NavigationService $navigationService)
{
$this->navigationService = $navigationService;
}
public function create(View $view)
{
$user = Auth::user();
$menuItems = $this->navigationService->getMenuItems($user);
$view->with('navigationMenu', $menuItems);
}
}
// app/Providers/AppServiceProvider.php
public function boot()
{
View::creator('layouts.app', NavigationCreator::class);
}
// resources/views/layouts/app.blade.php
<nav>
<ul>
@foreach($navigationMenu as $item)
<li><a href="{{ $item['url'] }}">{{ $item['label'] }}</a></li>
@endforeach
</ul>
</nav>
In this example, the NavigationCreator
prepares the navigation menu items based on the authenticated user's role. By using a View Creator, we ensure that this data is ready as soon as the main layout view is instantiated, potentially before other parts of the view start rendering.
View Creators vs. View Composers
While View Creators and View Composers serve similar purposes, their timing in the view lifecycle differs:
- View Creators run immediately after the view is instantiated.
- View Composers run just before the view is rendered.
This subtle difference can be leveraged for performance optimization or to set up data that other parts of your view logic might depend on.
View Creators in Laravel provide a powerful way to prepare data at the earliest possible point in the view rendering process. By leveraging View Creators, you can optimize data loading, ensure critical view data is always available, and create more efficient, responsive Laravel applications. Whether you're building complex, data-heavy interfaces or simply looking to streamline your view logic, View Creators offer a valuable tool in your Laravel development toolkit.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!