Optimizing Performance with Laravel's Benchmark Utility
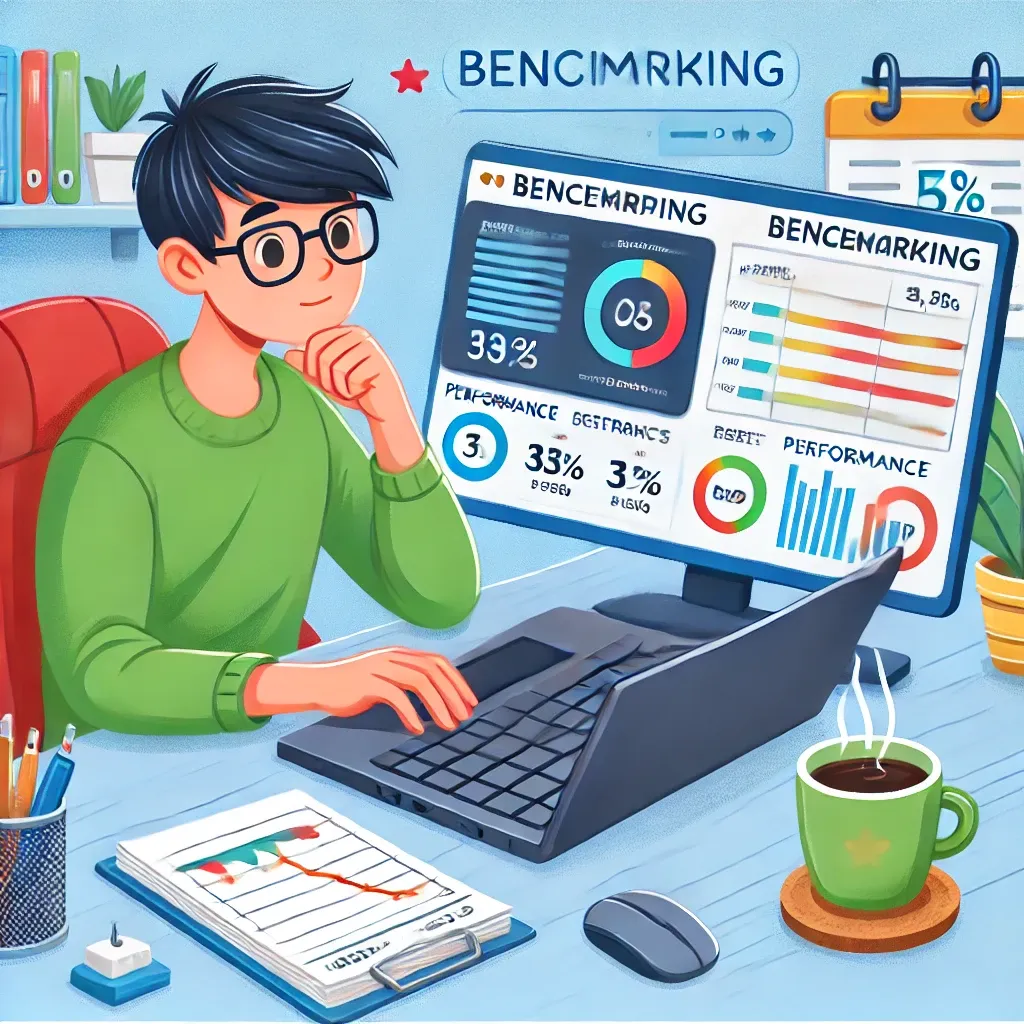
In the world of web development, performance is key. Laravel provides a powerful Benchmark utility that allows developers to measure and compare the execution time of different code snippets. This tool is invaluable for identifying bottlenecks and optimizing your application's performance. Let's dive into how you can leverage the Benchmark utility in your Laravel projects.
Basic Usage
The simplest way to use the Benchmark utility is with the dd
method, which measures and displays the execution time:
use App\Models\User;
use Illuminate\Support\Benchmark;
Benchmark::dd(fn () => User::find(1));
This will output the execution time in milliseconds, for example: 0.1 ms
Comparing Multiple Scenarios
You can compare the performance of different code snippets easily:
Benchmark::dd([
'Scenario 1' => fn () => User::count(),
'Scenario 2' => fn () => User::all()->count(),
]);
This might output something like:
Scenario 1: 0.5 ms
Scenario 2: 20.0 ms
This is particularly useful when you're deciding between different approaches to solve a problem.
Iterations for Accuracy
For very quick operations, a single execution might not give you an accurate picture. You can specify the number of iterations to run:
Benchmark::dd(fn () => User::count(), iterations: 10); // 0.5 ms
This runs the operation 10 times and returns the average execution time.
Capturing Results
Sometimes you need both the result of an operation and its execution time. The value
method returns a tuple with both:
[$count, $duration] = Benchmark::value(fn () => User::count());
echo "Found $count users in $duration milliseconds";
Real-World Example: Optimizing a Dashboard Query
Let's say you're building a dashboard and need to fetch some user statistics. You have two methods and want to know which is faster:
use App\Models\User;
use Illuminate\Support\Benchmark;
$results = Benchmark::dd([
'Method 1' => fn () => User::whereHas('posts', '>', 5)->count(),
'Method 2' => fn () => User::withCount('posts')->having('posts_count', '>', 5)->count()
]);
// Output might look like:
// Method 1: 15.2 ms
// Method 2: 8.7 ms
This clearly shows that Method 2 is faster, helping you make an informed decision about which query to use in your dashboard.
Laravel's Benchmark utility is a powerful tool for developers looking to optimize their applications. By providing easy-to-use methods for timing and comparing code execution, it enables data-driven decision-making in performance optimization. Whether you're fine-tuning database queries, optimizing algorithms, or just curious about the performance implications of different code approaches, the Benchmark utility is an invaluable asset in your Laravel toolkit.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!