Optimizing Long-Running Processes with LazyCollection's takeUntilTimeout()
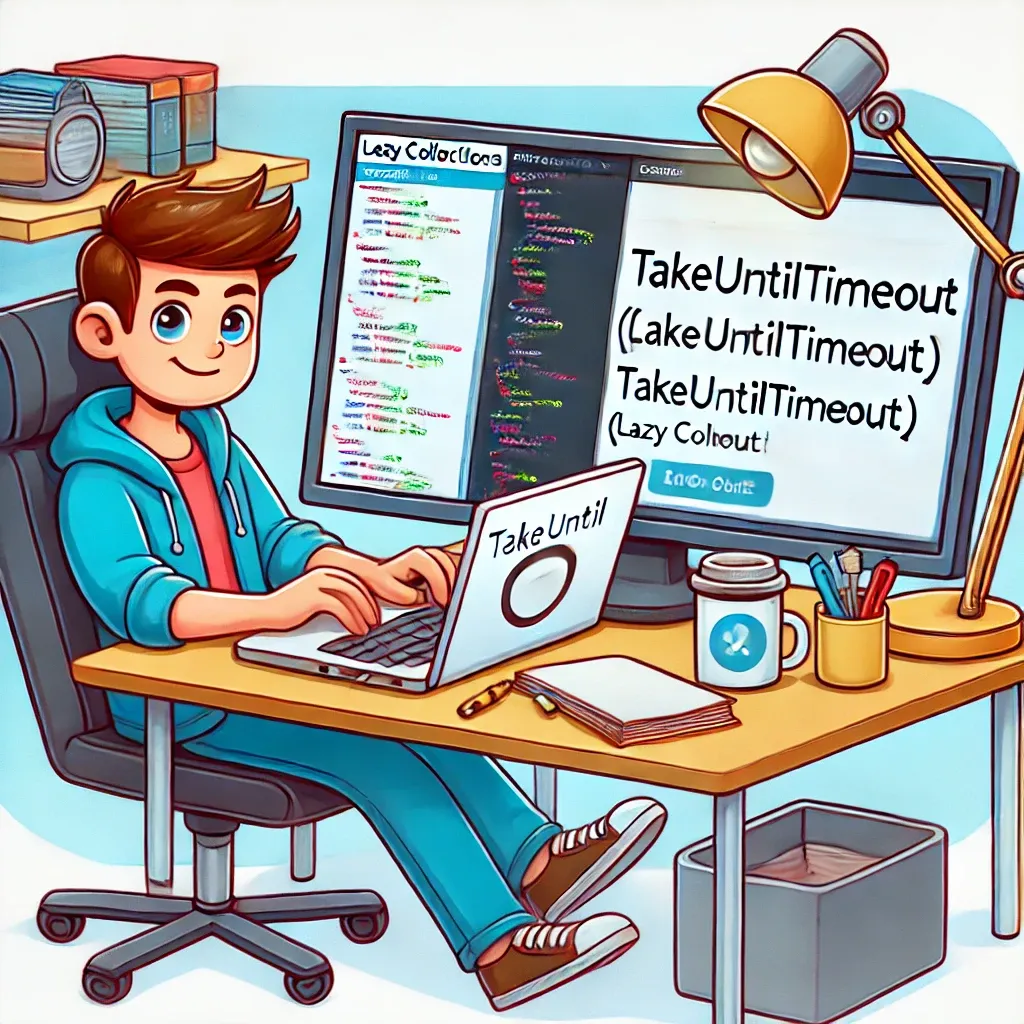
Laravel's LazyCollection provides powerful tools for working with large datasets efficiently. One particularly useful method is takeUntilTimeout()
, which allows you to process items from a lazy collection until a specified timeout is reached. This feature is invaluable when dealing with time-sensitive operations or when you need to ensure your processes don't run indefinitely.
Understanding takeUntilTimeout()
The takeUntilTimeout()
method continues to take items from a lazy collection until a specified timestamp is reached. This allows you to process as many items as possible within a given time frame.
Basic Usage
Here's a simple example of how to use takeUntilTimeout()
:
use Illuminate\Support\LazyCollection;
$result = LazyCollection::make(function () {
while (true) {
yield process_item();
}
})
->takeUntilTimeout(now()->addMinutes(5))
->all();
In this example, the collection will continue to yield items from process_item()
for up to 5 minutes.
Practical Application: Processing Large Datasets
Let's consider a more practical scenario where you need to process a large number of records from a database, but you want to ensure the operation doesn't exceed a certain time limit:
use Illuminate\Support\LazyCollection;
use Illuminate\Support\Facades\DB;
$processed = DB::table('large_table')
->orderBy('id')
->lazy()
->takeUntilTimeout(now()->addMinutes(10))
->map(function ($item) {
// Perform some time-consuming operation
return process_item($item);
})
->filter()
->count();
echo "Processed {$processed} items in 10 minutes or less.";
This script will process as many items as it can in 10 minutes, then stop.
Combining with chunk() for Efficient Batch Processing
For even more efficient processing, especially when dealing with database records, you can combine takeUntilTimeout()
with chunk()
:
$processed = LazyCollection::make(function () {
$lastId = 0;
while (true) {
$chunk = DB::table('large_table')
->where('id', '>', $lastId)
->orderBy('id')
->take(1000)
->get();
if ($chunk->isEmpty()) {
break;
}
foreach ($chunk as $item) {
yield $item;
}
$lastId = $chunk->last()->id;
}
})
->takeUntilTimeout(now()->addMinutes(15))
->map(function ($item) {
return process_item($item);
})
->filter()
->count();
echo "Processed {$processed} items in 15 minutes or less.";
This approach allows for efficient database querying while still maintaining the time limit.
Error Handling and Logging
When using takeUntilTimeout()
, it's important to implement proper error handling and logging:
use Illuminate\Support\Facades\Log;
$processed = LazyCollection::make(function () {
while (true) {
try {
yield process_item();
} catch (\Exception $e) {
Log::error("Error processing item: " . $e->getMessage());
continue;
}
}
})
->takeUntilTimeout(now()->addMinutes(5))
->count();
Log::info("Processed {$processed} items before timeout.");
This ensures that any errors during processing are logged, and you have a record of how many items were successfully processed.
Testing with takeUntilTimeout()
When writing tests for code that uses takeUntilTimeout()
, you can use Laravel's time manipulation helpers:
use Illuminate\Support\Facades\Date;
public function testProcessingWithTimeout()
{
Date::setTestNow(now());
$result = LazyCollection::make(function () {
while (true) {
Date::setTestNow(Date::getTestNow()->addSeconds(30));
yield 'item';
}
})
->takeUntilTimeout(now()->addMinutes(5))
->count();
$this->assertEquals(10, $result);
}
This test simulates a process where each item takes 30 seconds to process, and verifies that the collection stops after 5 minutes (10 items).
Laravel's takeUntilTimeout()
method for LazyCollections provides a powerful way to manage long-running processes and ensure they complete within specified time constraints. By leveraging this feature, you can build more robust and efficient applications that can handle large datasets without the risk of processes running indefinitely.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!