Optimizing Large Dataset Processing with Laravel Lazy Collections
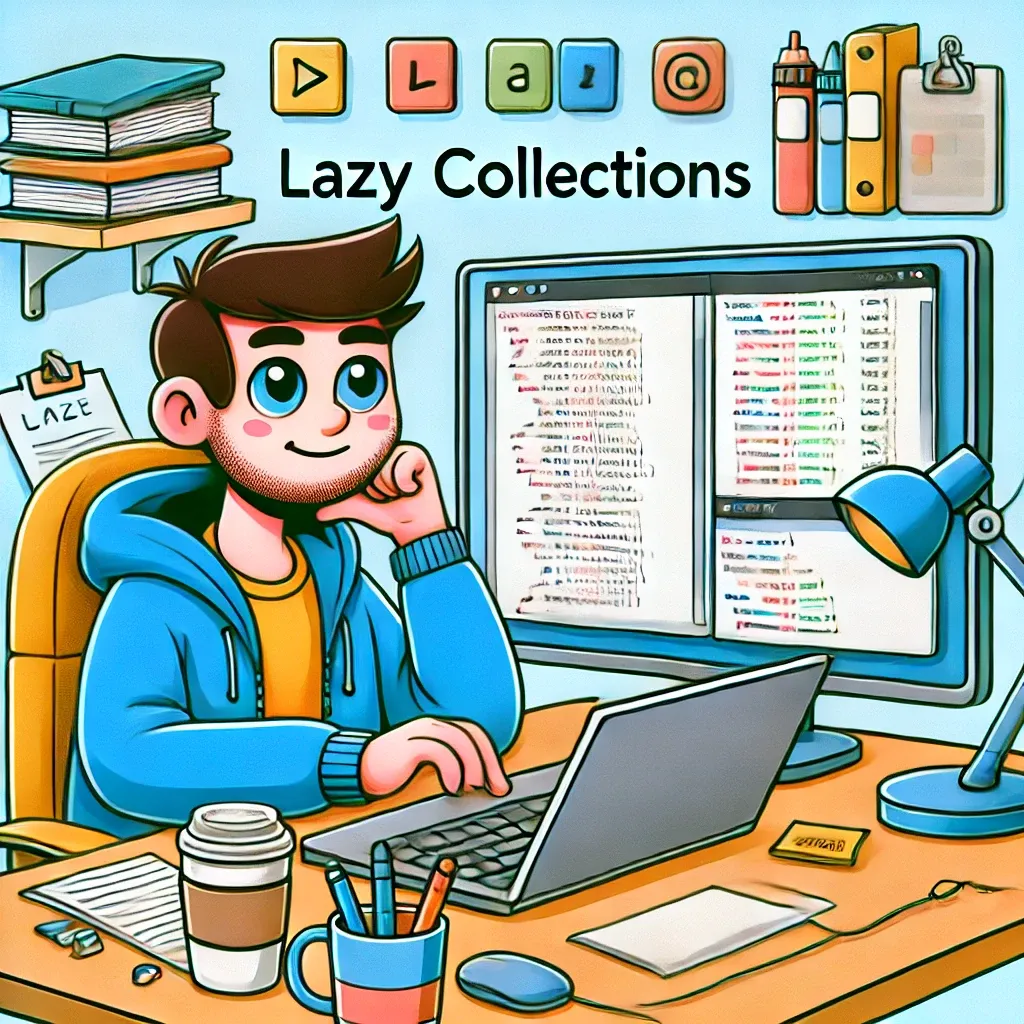
When dealing with large datasets in Laravel, memory usage can quickly become a bottleneck. Laravel's lazy collections offer an elegant solution to this problem, allowing you to work with large amounts of data efficiently. Let's explore how to leverage this powerful feature in your Laravel applications.
Understanding Lazy Collections
Lazy collections, introduced in Laravel 6.0, allow you to work with very large datasets without loading the entire dataset into memory at once. They work by only loading items as they're needed, making them perfect for processing large files or working with big database result sets.
Basic Usage
Here's a simple example of how to create and use a lazy collection:
use Illuminate\Support\LazyCollection;
LazyCollection::make(function () {
$handle = fopen('large-file.csv', 'r');
while (($line = fgets($handle)) !== false) {
yield str_getcsv($line);
}
})->each(function ($row) {
// Process each row
});
This code reads a large CSV file line by line, without loading the entire file into memory.
Working with Database Results
Lazy collections are particularly useful when working with large database result sets:
User::cursor()->each(function ($user) {
// Process each user
});
The cursor()
method returns a lazy collection, allowing you to iterate over large numbers of database records efficiently.
Chunking Results
For even more efficient processing, you can combine lazy collections with chunking:
LazyCollection::make(function () {
for ($i = 0; $i < 1000000; $i++) {
yield $i;
}
})
->chunk(1000)
->each(function ($chunk) {
// Process chunk of 1000 items
DB::table('numbers')->insert($chunk->all());
});
This approach is particularly useful when you need to perform batch operations, like inserting data into the database.
Transforming Data
Lazy collections support many of the same methods as regular collections:
LazyCollection::make(function () {
yield from ['apple', 'banana', 'cherry'];
})
->map(function ($item) {
return strtoupper($item);
})
->each(function ($item) {
echo $item . "\n";
});
Real-World Example: Processing a Large Log File
Here's a more complex example that processes a large log file:
LazyCollection::make(function () {
$handle = fopen('large-log-file.log', 'r');
while (($line = fgets($handle)) !== false) {
yield $line;
}
})
->map(function ($line) {
return json_decode($line, true);
})
->filter(function ($log) {
return $log['level'] === 'error';
})
->chunk(100)
->each(function ($chunk) {
ErrorLog::insert($chunk->all());
});
This script reads a large log file line by line, decodes each line from JSON, filters for error logs, and inserts them into the database in chunks of 100.
Performance Considerations
While lazy collections are memory-efficient, they may be slower for smaller datasets due to the overhead of generating items on-demand. For small to medium-sized datasets, regular collections might be more appropriate.
Lazy collections in Laravel provide a powerful tool for working with large datasets efficiently. By loading data on-demand, they allow you to process vast amounts of information without running into memory limitations. Whether you're dealing with large files, extensive database queries, or any scenario involving big data, lazy collections can significantly improve your application's performance and scalability.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!