Optimizing Database Queries: Preventing Lazy Loading in Laravel
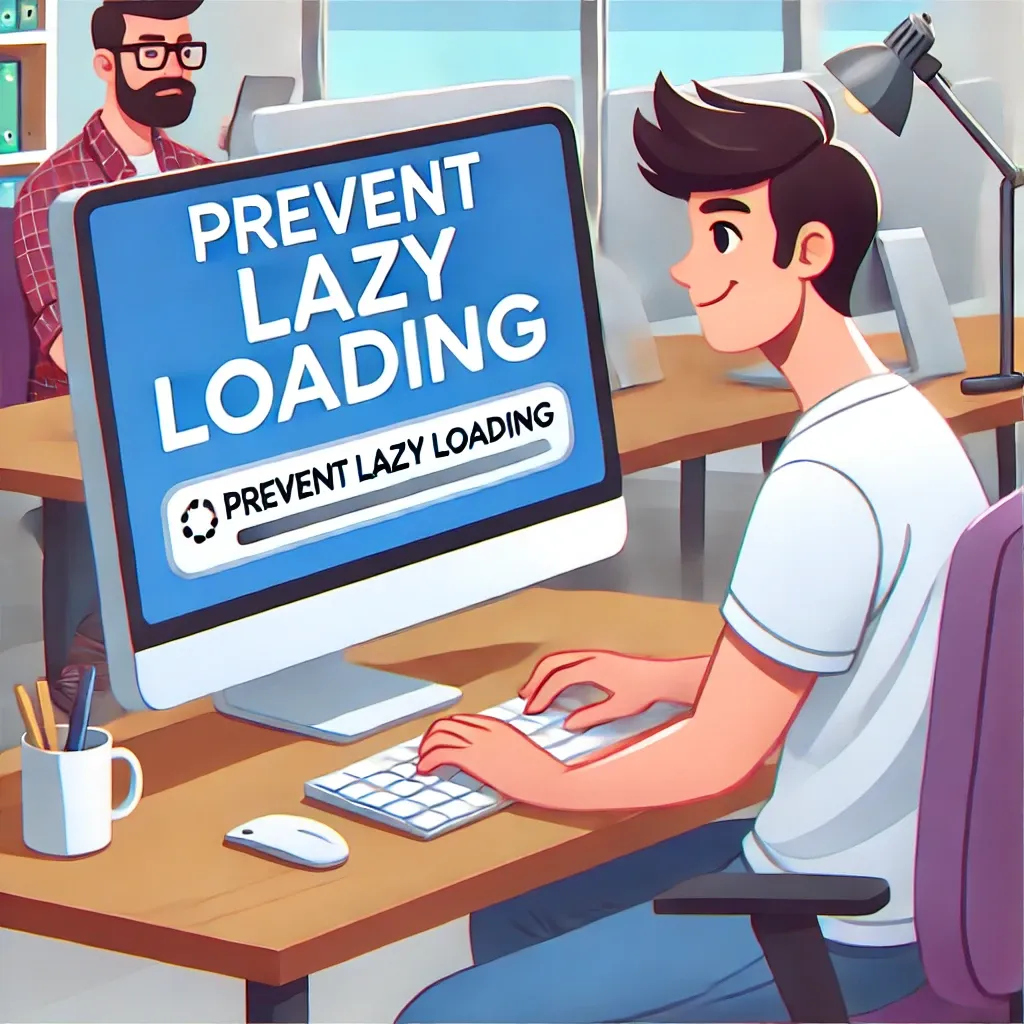
Laravel's Eloquent ORM is known for its ease of use, particularly when it comes to working with relationships. However, this convenience can sometimes lead to performance issues, particularly in the form of the N+1 query problem. Laravel provides a powerful feature to help combat this: the ability to prevent lazy loading. Let's dive into how this can significantly improve your application's performance.
Understanding Lazy Loading
Lazy loading is Eloquent's default behavior when accessing relationships. It means that relationship data is loaded from the database only when you actually access the relationship property. While convenient, this can lead to performance issues, especially when dealing with collections of models.
The N+1 Query Problem
Consider this scenario:
$posts = Post::all();
foreach ($posts as $post) {
echo $post->author->name;
}
This seemingly innocent code will execute N+1 queries: 1 query to fetch all posts, and then N queries (where N is the number of posts) to fetch the author for each post.
Preventing Lazy Loading
Laravel allows you to prevent lazy loading entirely, which can help identify these N+1 query issues during development. Here's how you can enable this feature:
use Illuminate\Database\Eloquent\Model;
Model::preventLazyLoading(!app()->isProduction());
This code, typically placed in your AppServiceProvider
, will disable lazy loading in all environments except production.
Handling Lazy Loading Violations
When lazy loading is prevented and your code attempts to lazy load a relationship, Laravel will throw a Illuminate\Database\LazyLoadingViolationException
. You can customize how these violations are handled:
Model::handleLazyLoadingViolationUsing(function (Model $model, string $relation) {
$class = get_class($model);
logger()->warning("Attempted to lazy load [{$relation}] on model [{$class}].");
});
This approach logs warnings instead of throwing exceptions, which can be useful for identifying issues without breaking the application flow.
Practical Application
Let's look at a real-world scenario where preventing lazy loading can be beneficial:
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', compact('posts'));
}
}
In the corresponding view:
@foreach ($posts as $post)
<h2>{{ $post->title }}</h2>
<p>By {{ $post->author->name }}</p>
@endforeach
With lazy loading prevention enabled, this code would throw an exception (or log a warning, depending on your configuration). This immediately alerts you to the N+1 query issue, which you can then fix by eager loading the relationship:
class PostController extends Controller
{
public function index()
{
$posts = Post::with('author')->get();
return view('posts.index', compact('posts'));
}
}
Real-World Scenario: API Optimization
Imagine you're building an API for a blogging platform. You might have an endpoint to fetch recent posts:
public function recentPosts()
{
$posts = Post::latest()->take(10)->get();
return PostResource::collection($posts);
}
Your PostResource
might look like this:
class PostResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'title' => $this->title,
'author' => $this->author->name,
'comments_count' => $this->comments->count(),
];
}
}
With lazy loading prevention, this would immediately alert you to potential N+1 queries for both the author
relationship and the comments
count. You could then optimize your controller:
public function recentPosts()
{
$posts = Post::with('author')
->withCount('comments')
->latest()
->take(10)
->get();
return PostResource::collection($posts);
}
This optimized version fetches all necessary data in just two queries, regardless of the number of posts.
Preventing lazy loading in Laravel is a powerful tool for identifying and addressing potential performance issues in your application. By forcing you to be explicit about your data requirements, it encourages more efficient database querying patterns and can significantly improve your application's performance.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!