Optimizing API Performance with Conditional Relationship Loading in Laravel
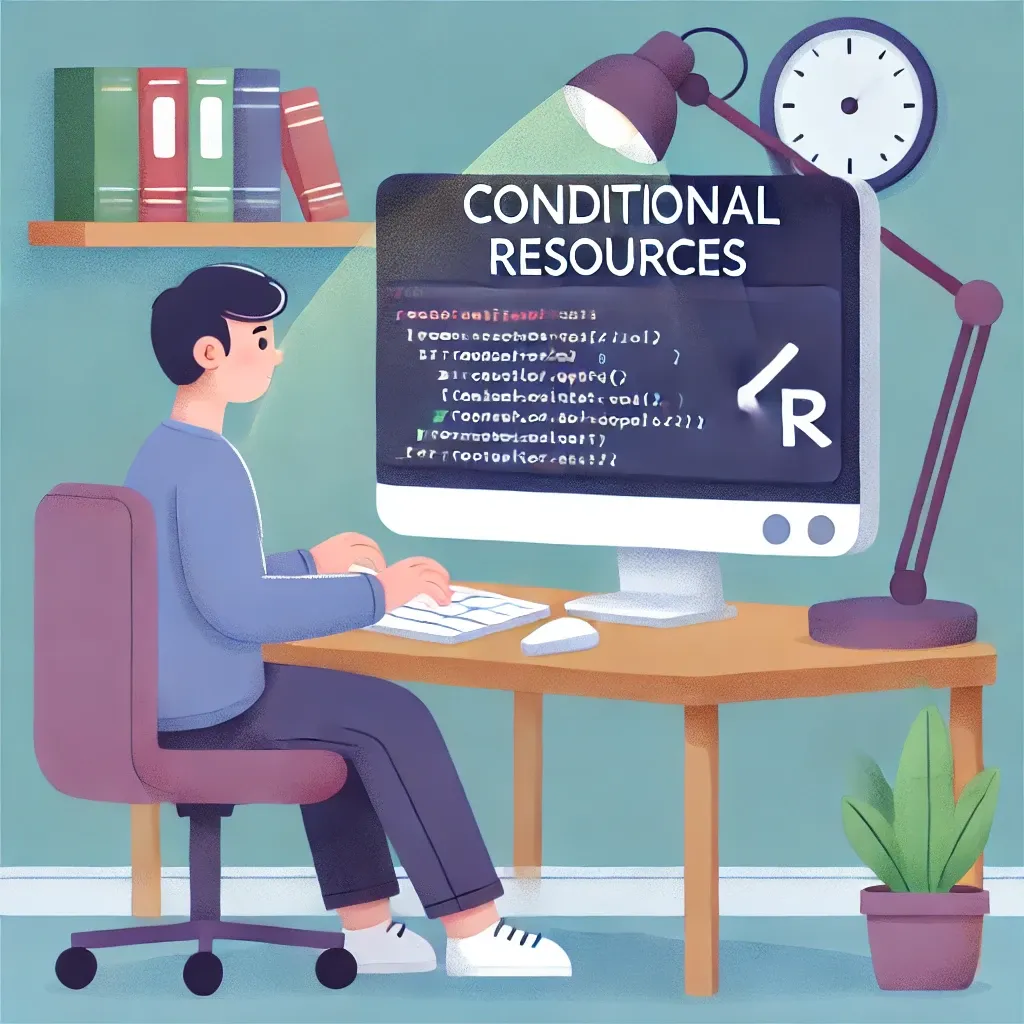
When building APIs with Laravel, it's crucial to consider performance, especially when dealing with relationship data. Laravel's API Resources provide a powerful feature called whenLoaded
that allows you to conditionally include relationships in your API responses. This can significantly reduce unnecessary database queries and improve your API's efficiency.
Understanding the Problem
In many API scenarios, you might have resources that include related data. For example, a user resource might include their posts. However, you don't always want to load and return this related data, especially if it's not needed for every request.
The whenLoaded Method
Laravel's whenLoaded
method in API Resources provides an elegant solution to this problem. It allows you to include a relationship in the resource only if it has been explicitly loaded on the model.
Here's a basic example of how to use whenLoaded
:
<?php
namespace App\Http\Resources;
use Illuminate\Http\Resources\Json\JsonResource;
class UserResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'name' => $this->name,
'email' => $this->email,
'posts' => PostResource::collection($this->whenLoaded('posts')),
'created_at' => $this->created_at,
'updated_at' => $this->updated_at,
];
}
}
In this example, the posts
relationship will only be included in the API response if it has been explicitly loaded on the user model.
Practical Application
Let's consider a scenario where you have an API endpoint that can return users with or without their posts, depending on the client's needs.
In your controller:
public function index(Request $request)
{
$users = User::query();
if ($request->include_posts) {
$users->with('posts');
}
return UserResource::collection($users->get());
}
Now, clients can request users with or without posts:
/api/users
will return users without posts/api/users?include_posts=1
will return users with their posts
This approach allows you to optimize your API's performance by only loading and returning the data that's actually needed.
Advanced Usage
You can also use whenLoaded
with a callback for more complex transformations:
'latest_post' => $this->whenLoaded('posts', function () {
return new PostResource($this->posts->latest()->first());
})
This will include only the latest post if the posts relationship is loaded.
Handling Nested Relationships
whenLoaded
is particularly powerful when dealing with nested relationships. For example:
class PostResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->content,
'author' => new UserResource($this->whenLoaded('author')),
'comments' => CommentResource::collection($this->whenLoaded('comments')),
];
}
}
This allows you to efficiently handle complex, nested data structures without overloading your API responses.
The whenLoaded
method in Laravel's API Resources provides a powerful way to optimize your API's performance and flexibility. By conditionally including relationships, you can create efficient, scalable APIs that only return the data that's actually needed. This leads to faster response times, reduced server load, and a better experience for your API consumers.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!