Optimize your Blade views with Laravel's @once directive
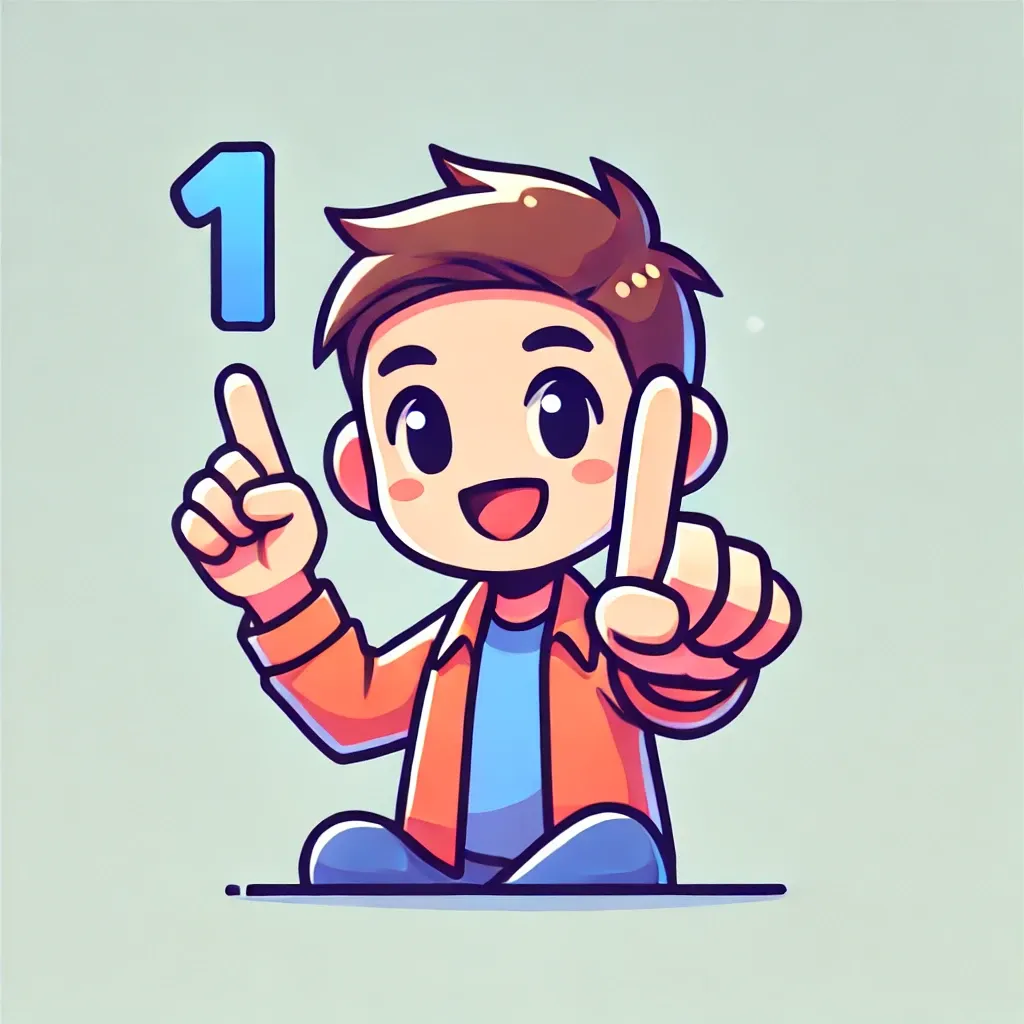
Managing Blade templates efficiently is crucial for maintaining clean and organized views in Laravel applications. One common challenge is ensuring that certain pieces of code, like JavaScript snippets or styles, are only included once in a page, even if the component is rendered multiple times. Laravel's @once
directive provides a simple and elegant solution to this problem.
Understanding @once
The @once
directive allows you to wrap a block of code that should only be included once in the rendered HTML, regardless of how many times the enclosing component or view is included. This is particularly useful for things like including JavaScript or CSS snippets in components that might be rendered multiple times on the same page.
Basic Usage
Here’s a basic example to illustrate how @once
works:
@once
<style>
.unique-style {
color: red;
}
</style>
@endonce
<div class="unique-style">
This text will be red.
</div>
In this example, the style block will only be included once in the rendered HTML, even if this snippet is part of a Blade component that is included multiple times.
Real-Life Example
Consider a scenario where you have a custom Blade component for displaying user profiles, and you want to include a JavaScript snippet that initializes some JavaScript logic only once, no matter how many user profiles are rendered on the page. Here’s how you can implement this using @once
:
- Blade Component:
// resources/views/components/user-profile.blade.php
<div class="user-profile">
<h2>{{ $user->name }}</h2>
<p>{{ $user->email }}</p>
@once
<script>
document.addEventListener('DOMContentLoaded', function () {
console.log('JavaScript initialized for user profiles');
});
</script>
@endonce
</div>
- Using the Component in a View:
<!-- resources/views/users.blade.php -->
@foreach ($users as $user)
<x-user-profile :user="$user" />
@endforeach
In this example, the JavaScript snippet inside the @once
directive will only be included once in the final HTML, regardless of how many times the user-profile
component is rendered in the users.blade.php
view.
Pro Tip
The @once
directive can also be used to conditionally include other types of content that should only appear once. For example, you might use it to include a set of meta tags or an analytics script:
@once
<meta name="description" content="This is a unique page description">
@endonce
This ensures that the meta tag is only included once in the rendered HTML, maintaining the uniqueness required by HTML standards.
Conclusion
The @once
directive in Laravel Blade is a powerful tool for managing your templates more efficiently. By ensuring that certain pieces of code are only included once, you can avoid duplication and maintain cleaner, more organized views.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!