Navigate URI Paths Elegantly with Laravel's New pathSegments() Method
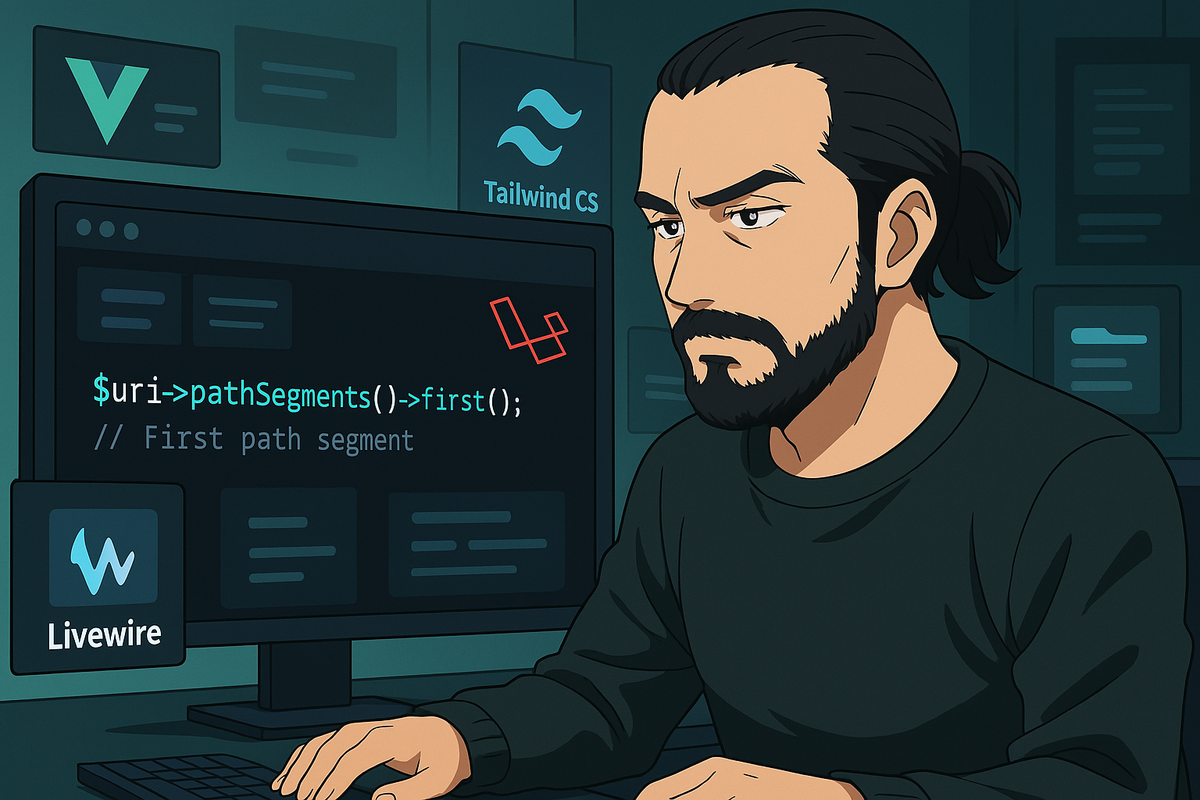
Need to work with individual segments of a URI path in Laravel? The new pathSegments() method offers a cleaner, more fluent approach to accessing URI path components.
When working with URLs in web applications, you often need to extract or manipulate specific parts of the path. Previously, this required splitting the path string and creating a collection manually. Laravel's Uri class now includes a pathSegments() method that streamlines this process by returning the path components as a ready-to-use collection.
Let's see how it works:
use Illuminate\Support\Facades\Uri;
$uri = Uri::of('https://laravel.com/docs/11.x/validation');
// Get all path segments as a collection
$segments = $uri->pathSegments(); // ['docs', '11.x', 'validation']
// Access specific segments using collection methods
$firstSegment = $uri->pathSegments()->first(); // 'docs'
$lastSegment = $uri->pathSegments()->last(); // 'validation'
$secondSegment = $uri->pathSegments()->get(1); // '11.x'
Before and After Comparison
The improvement in readability and convenience is substantial:
// Before: manual string splitting and collection creation
$path = Uri::of('https://laravel.com/docs/11.x/validation')->path();
$segments = collect(explode('/', ltrim($path, '/')));
$firstSegment = $segments->first();
// After: clean, fluent approach
$firstSegment = Uri::of('https://laravel.com/docs/11.x/validation')
->pathSegments()
->first();
Real-World Example
This method is particularly useful when building routing logic, breadcrumbs, or navigation structures:
class DocumentationController extends Controller
{
public function show(Request $request)
{
$uri = Uri::of($request->url());
$segments = $uri->pathSegments();
// Extract version and page from URL
$section = $segments->get(0); // 'docs'
$version = $segments->get(1); // '11.x'
$page = $segments->get(2); // 'validation'
// Build breadcrumbs based on path segments
$breadcrumbs = $segments->map(function ($segment, $index) use ($segments) {
$url = '/'.implode('/', $segments->take($index + 1)->all());
return ['name' => $segment, 'url' => $url];
});
return view('documentation.show', [
'section' => $section,
'version' => $version,
'page' => $page,
'breadcrumbs' => $breadcrumbs,
]);
}
}
By leveraging the new pathSegments() method, you can create cleaner, more maintainable code when working with URL paths in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!