Monitoring Database Connections with Laravel's db:monitor
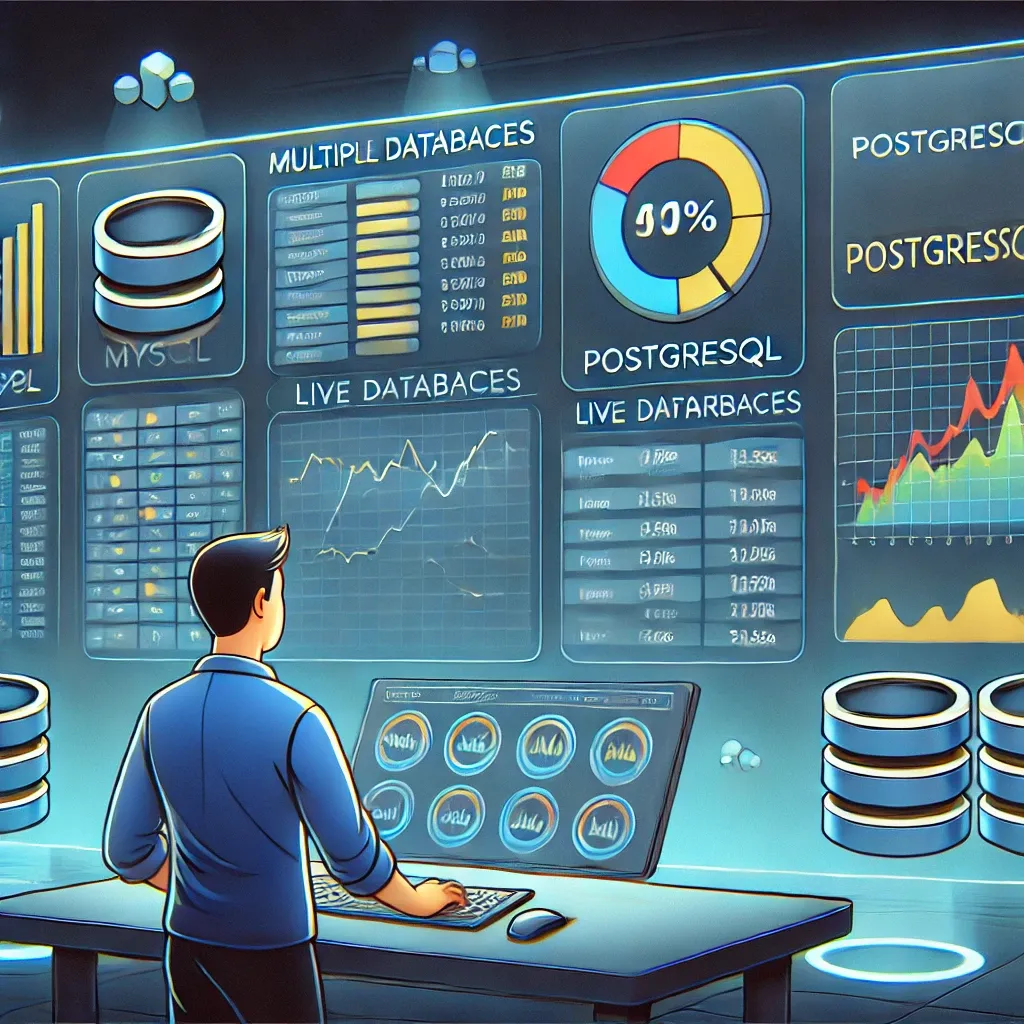
Need to monitor your database connections proactively? Laravel's db:monitor command helps you track open connections and react when they exceed your defined thresholds.
Starting from Laravel 9.24, you can monitor your database connections and receive notifications when they approach critical levels. This feature helps prevent connection pool exhaustion by alerting you before issues arise.
Let's see how it works:
// Schedule monitoring for specific databases with thresholds
php artisan db:monitor --databases=mysql,pgsql --max=100
Real-World Example
Here's how to set up database connection monitoring:
// App\Providers\AppServiceProvider
use App\Notifications\DatabaseApproachingMaxConnections;
use Illuminate\Database\Events\DatabaseBusy;
use Illuminate\Support\Facades\Event;
use Illuminate\Support\Facades\Notification;
public function boot(): void
{
// Listen for DatabaseBusy events
Event::listen(function (DatabaseBusy $event) {
Notification::route('mail', 'dev@example.com')
->notify(new DatabaseApproachingMaxConnections(
$event->connectionName,
$event->connections
));
});
}
// App\Console\Kernel
protected function schedule(Schedule $schedule)
{
// Monitor every minute
$schedule->command('db:monitor --database=mysql --max=100')
->everyMinute();
}
// App\Notifications\DatabaseApproachingMaxConnections
class DatabaseApproachingMaxConnections extends Notification
{
public function toMail($notifiable)
{
return (new MailMessage)
->error()
->subject('Database Connection Alert')
->line("The {$this->connectionName} database has {$this->connections} open connections.")
->line('This exceeds the configured threshold.');
}
}
The db:monitor command provides a simple yet effective way to prevent database connection issues. Think of it as a watchdog for your database connections - it continuously monitors the connection pool and alerts you when you're approaching dangerous territory.
This becomes especially valuable when:
- Running applications with high concurrent users
- Managing multiple database connections
- Debugging connection pool issues
- Ensuring application stability
- Planning for scaling operations
For example, setting a threshold at 80% of your maximum connections gives you time to react before the pool is exhausted.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!