Monitoring Cache Operations in Laravel: Events Guide
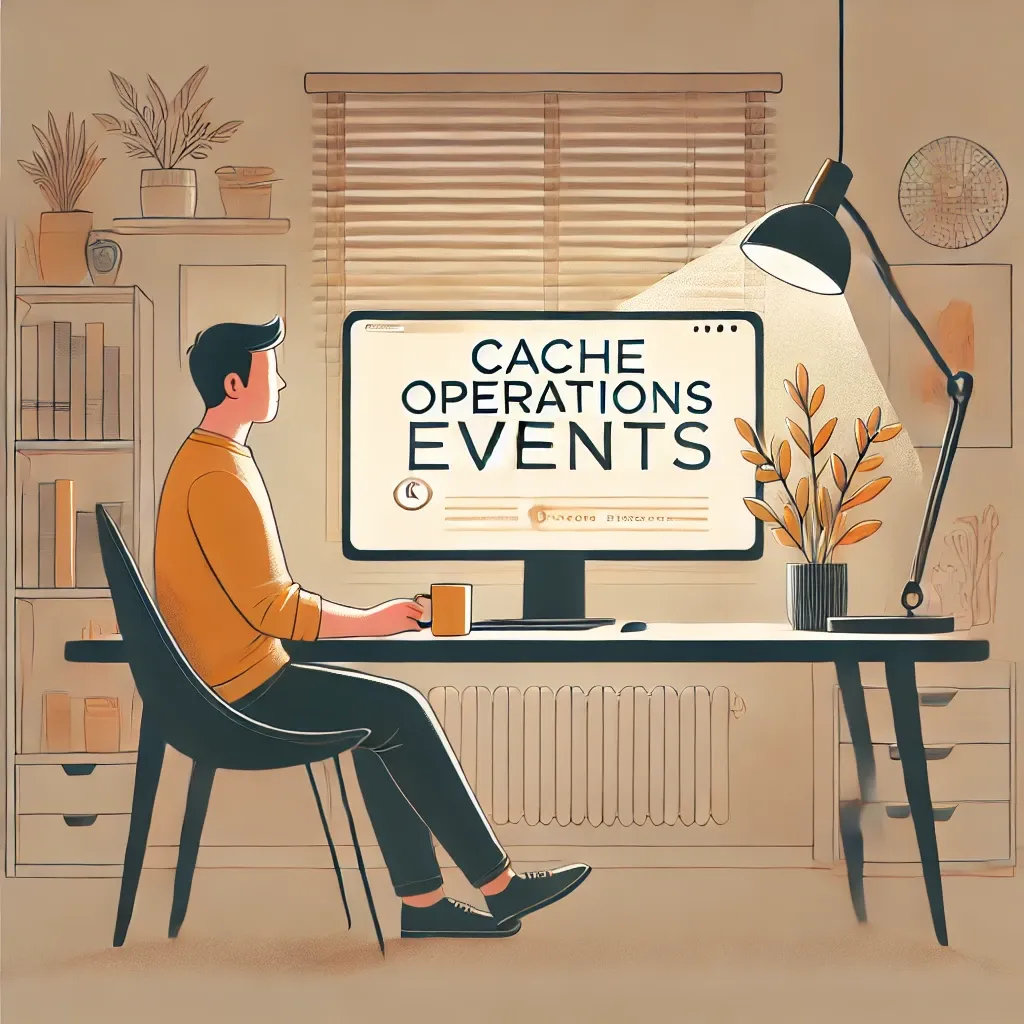
Want to track what's happening with your cache? Laravel's cache events let you monitor every operation! Let's explore how to leverage these powerful events.
Available Cache Events
Laravel dispatches events for key cache operations:
use Illuminate\Cache\Events\{
CacheHit,
CacheMissed,
KeyForgotten,
KeyWritten
};
Simple Implementation
Here's how to listen for cache events:
class CacheEventSubscriber
{
public function handleCacheHit(CacheHit $event)
{
Log::info("Cache hit: {$event->key}");
}
public function handleCacheMiss(CacheMissed $event)
{
Log::info("Cache miss: {$event->key}");
}
}
Real-World Example
Here's a practical implementation for monitoring cache performance:
class CacheMonitoringService
{
public function subscribe($events)
{
$events->listen(CacheHit::class, function ($event) {
$this->recordMetric('hits', [
'key' => $event->key,
'tags' => $event->tags,
'response_time' => microtime(true) - LARAVEL_START
]);
});
$events->listen(CacheMissed::class, function ($event) {
$this->recordMetric('misses', [
'key' => $event->key,
'tags' => $event->tags
]);
});
$events->listen(KeyWritten::class, function ($event) {
$this->recordMetric('writes', [
'key' => $event->key,
'tags' => $event->tags,
'ttl' => $event->seconds ?? 'forever'
]);
});
}
private function recordMetric(string $type, array $data)
{
// Store metrics in your monitoring system
// Example: StatsD, Prometheus, etc.
}
}
// In a service provider:
public function boot()
{
if (config('cache.monitoring.enabled')) {
(new CacheMonitoringService)->subscribe($this->app['events']);
}
}
Disabling Events
For better performance, you can disable cache events in config/cache.php
:
'redis' => [
'driver' => 'redis',
'events' => false,
// other config...
],
Cache events provide valuable insights into your application's caching behavior, perfect for monitoring and debugging.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!