Mastering Task Scheduling in Laravel: Automating Your Application
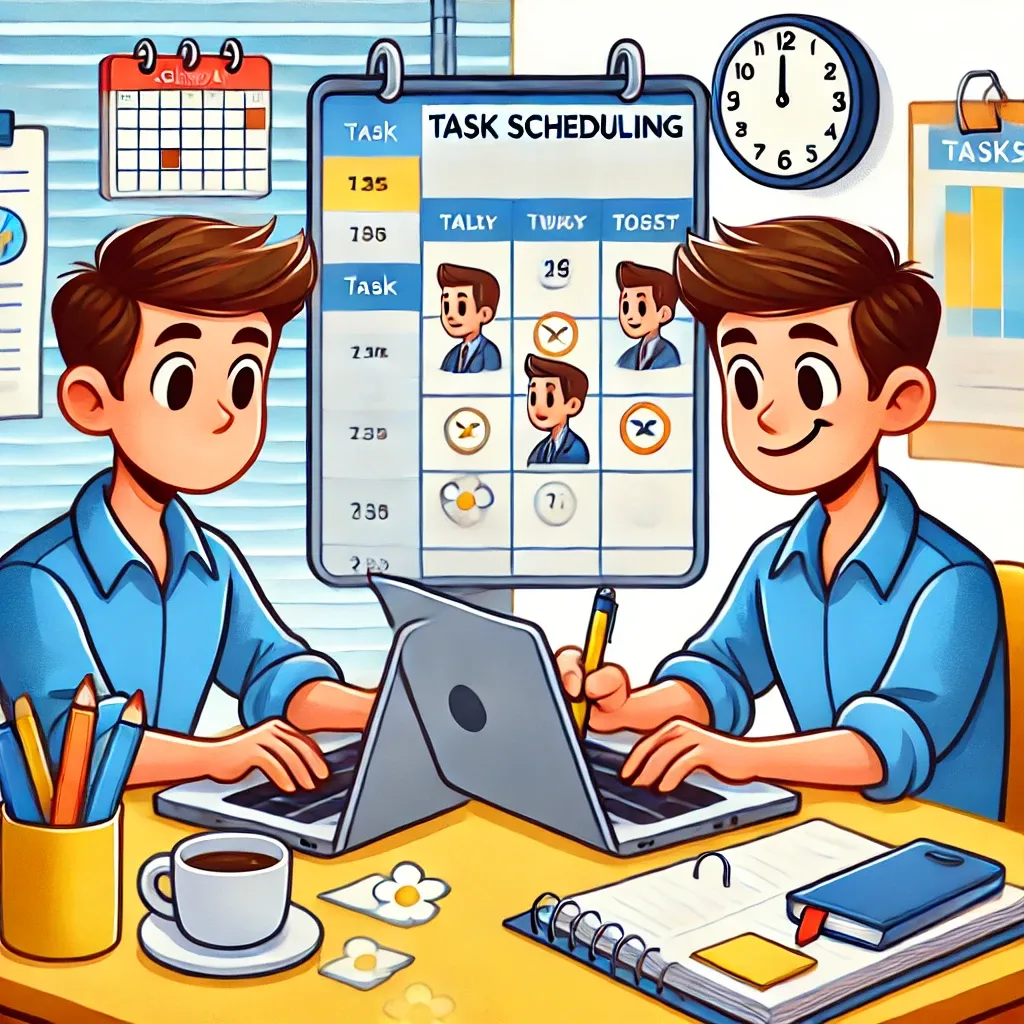
Laravel's Task Scheduling feature provides a powerful and expressive way to manage cron jobs within your application. Let's dive into how you can leverage this feature to automate recurring tasks efficiently.
Basic Task Scheduling
To get started with task scheduling, you'll be working in the app/Console/Kernel.php
file. Here's a basic example:
protected function schedule(Schedule $schedule)
{
$schedule->command('emails:send-weekly-digest')
->weekly()
->mondays()
->at('8:00')
->timezone('America/New_York');
}
This schedule runs the emails:send-weekly-digest
Artisan command every Monday at 8:00 AM, New York time.
Frequency Options
Laravel offers a variety of scheduling frequencies:
$schedule->command('hourly:update')->hourly();
$schedule->command('daily:cleanup')->daily();
$schedule->command('weekly:report')->weekly();
$schedule->command('monday:task')->weekly()->mondays();
$schedule->command('weekday:job')->weekdays();
$schedule->command('database:backup')->monthly();
You can also use cron expressions for more complex schedules:
$schedule->command('complex:task')->cron('0 */4 * * *'); // Every 4 hours
Handling Task Output
You can manage the output of your scheduled tasks:
$schedule->command('emails:send')
->daily()
->sendOutputTo(storage_path('logs/emails.log'))
->emailOutputTo('admin@example.com');
This saves the task output to a log file and emails it to an administrator.
Preventing Task Overlaps
For long-running tasks, prevent overlaps with:
$schedule->command('long:task')
->daily()
->withoutOverlapping();
Running Tasks on One Server
In a multi-server setup, ensure a task runs on only one server:
$schedule->command('report:generate')
->daily()
->onOneServer();
Background Tasks
Run tasks in the background:
$schedule->command('analytics:process')
->daily()
->runInBackground();
Maintenance Mode and Environments
Control task execution based on application state or environment:
$schedule->command('database:backup')
->daily()
->evenInMaintenanceMode();
$schedule->command('emails:send')
->daily()
->environments(['production']);
Hooks
Use before and after hooks for additional control:
$schedule->command('reports:daily')
->daily()
->before(function () {
// Preparation logic
})
->after(function () {
// Cleanup logic
});
Ping URLs
Notify external services about task completion:
$schedule->command('backup:run')
->daily()
->thenPing('https://beats.envoyer.io/heartbeat/xyz');
By mastering these Task Scheduling features, you can automate a wide range of processes in your Laravel application, from simple daily cleanups to complex, conditionally executed tasks across multiple servers.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!