Mastering Request Flow Control with Laravel Middleware
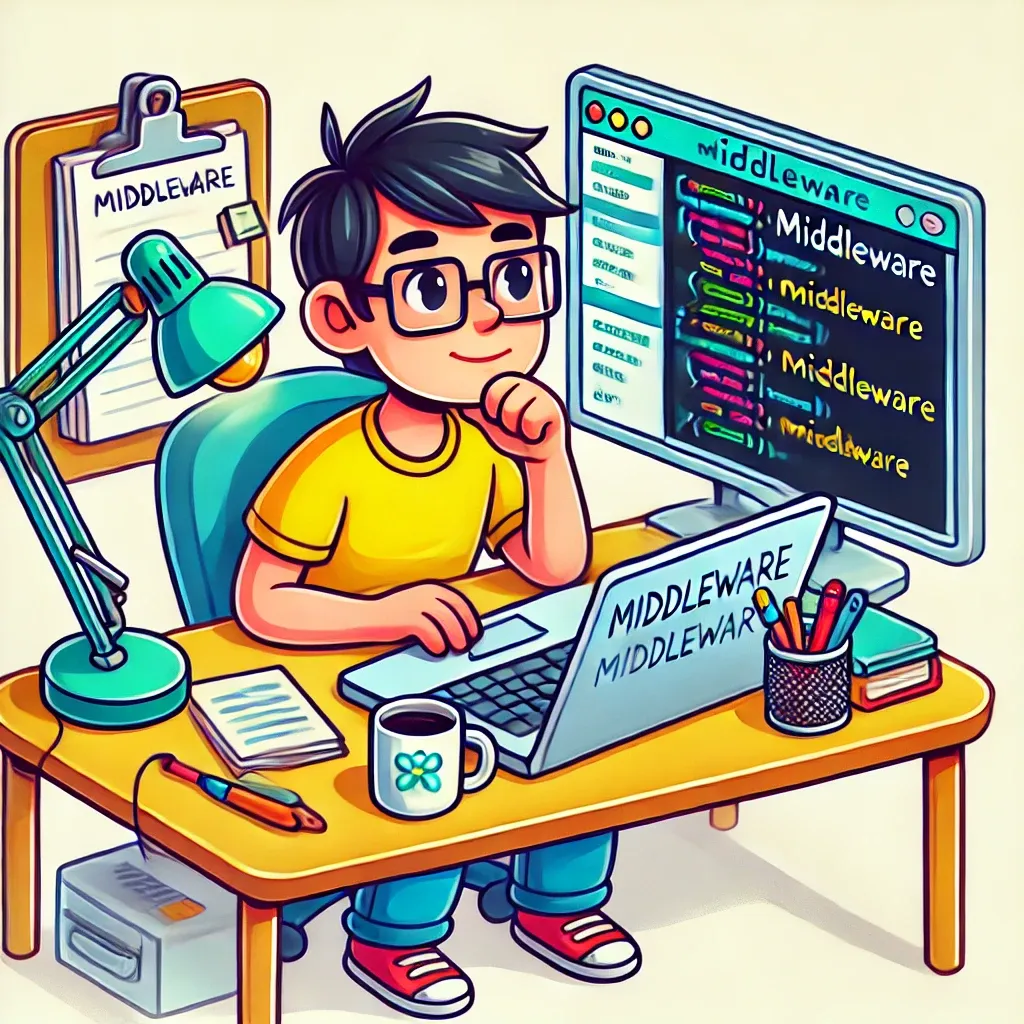
Laravel's middleware provides a powerful mechanism for filtering HTTP requests entering your application. Let's explore how to leverage middleware effectively in your Laravel projects.
Creating Middleware
Create a new middleware using the Artisan command:
php artisan make:middleware EnsureTokenIsValid
This generates a new middleware class:
namespace App\Http\Middleware;
use Closure;
class EnsureTokenIsValid
{
public function handle($request, Closure $next)
{
if ($request->input('token') !== 'my-secret-token') {
return response('Invalid Token', 403);
}
return $next($request);
}
}
Registering and Applying Middleware
Register route-specific middleware in app/Http/Kernel.php
:
protected $routeMiddleware = [
// ...
'token' => \App\Http\Middleware\EnsureTokenIsValid::class,
];
Apply middleware to routes:
Route::get('profile', function () {
//
})->middleware('token');
Middleware Parameters
Pass parameters to your middleware:
Route::put('post/{id}', function ($id) {
//
})->middleware('role:editor');
In your middleware:
public function handle($request, Closure $next, $role)
{
if (! $request->user()->hasRole($role)) {
return response('Unauthorized', 401);
}
return $next($request);
}
Before & After Middleware
Perform actions before or after the request is handled:
public function handle($request, Closure $next)
{
// Before the request is handled
$response = $next($request);
// After the request is handled
return $response;
}
Real-World Example: CORS Middleware
public function handle($request, Closure $next)
{
return $next($request)
->header('Access-Control-Allow-Origin', '*')
->header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS');
}
Laravel's middleware offers a clean, organized approach to filtering HTTP requests and responses. By leveraging middleware, you can create more secure and feature-rich applications while keeping your code modular and reusable.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!