Mastering Redis Transactions in Laravel: Atomic Operations Made Easy
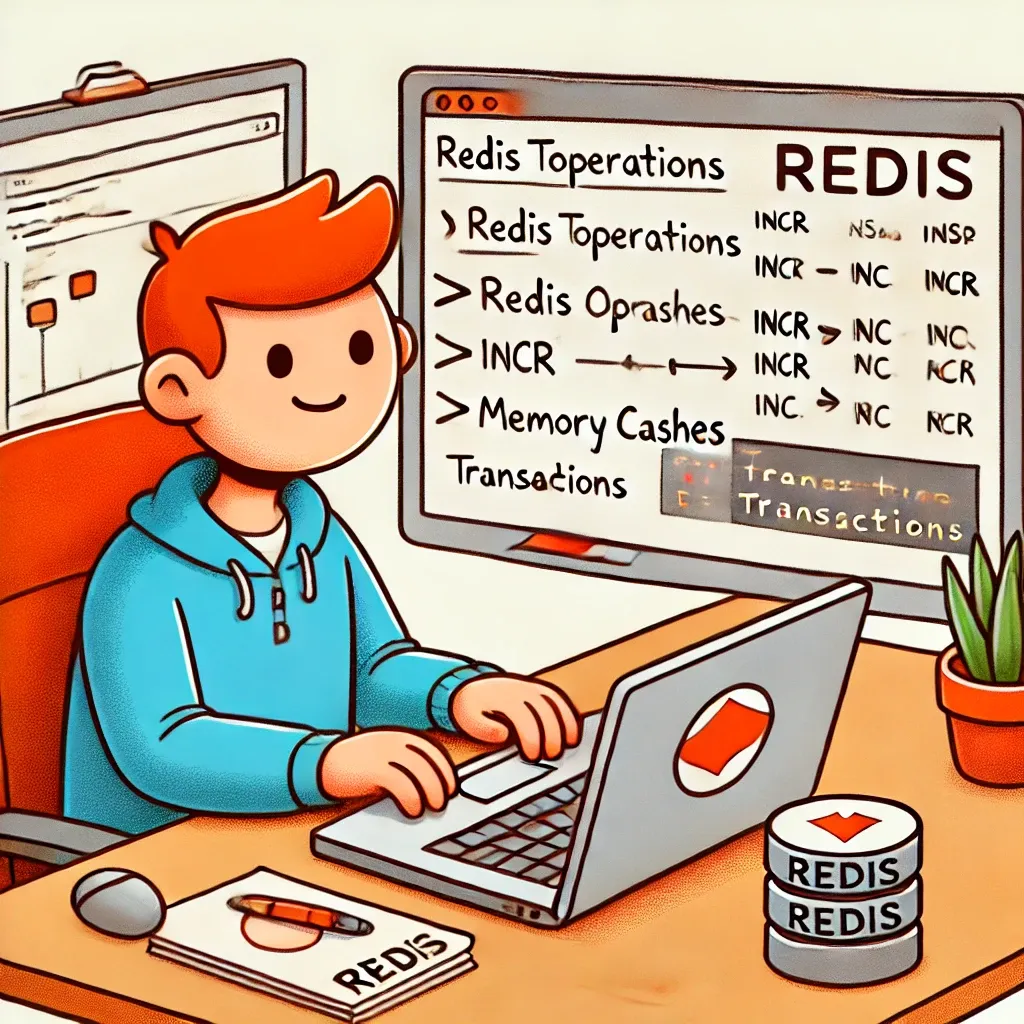
When working with Redis in Laravel, ensuring data consistency across multiple operations is crucial. Enter Redis transactions - a powerful feature that allows you to execute multiple commands as a single, atomic operation. Let's dive into how Laravel makes this process smooth and efficient.
Understanding Redis Transactions
In Redis, a transaction is a sequence of commands that are executed as a single, indivisible operation. Laravel provides a convenient wrapper around Redis' native MULTI
and EXEC
commands through the transaction
method of the Redis
facade.
The Laravel Way: Using the transaction Method
Here's how you can use the transaction
method in Laravel:
use Illuminate\Support\Facades\Redis;
Redis::transaction(function ($redis) {
$redis->incr('user_visits', 1);
$redis->incr('total_visits', 1);
});
In this example, we're incrementing two different counters atomically. Either both operations succeed, or neither does.
Key Points to Remember
- Atomic Execution: All commands within the closure are executed as a single, atomic operation.
- No Retrieving Values: You can't retrieve values from Redis within the transaction closure.
- Deferred Execution: The transaction isn't executed until the entire closure has finished.
A Practical Example: User Registration Counter
Let's say we want to track both new user registrations and total user count atomically:
use Illuminate\Support\Facades\Redis;
class UserController extends Controller
{
public function register(Request $request)
{
// User registration logic here...
Redis::transaction(function ($redis) {
$redis->incr('new_user_registrations_today');
$redis->incr('total_users');
});
return response()->json(['message' => 'User registered successfully']);
}
}
In this example, we ensure that both our daily new user counter and our total user counter are updated together. If any part of this transaction fails, neither counter will be incremented, maintaining data consistency.
By leveraging Redis transactions in Laravel, you can ensure data consistency and integrity in your applications, especially when dealing with complex, multi-step operations that need to succeed or fail as a unit.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!