Mastering Real-Time Validation in Laravel Livewire
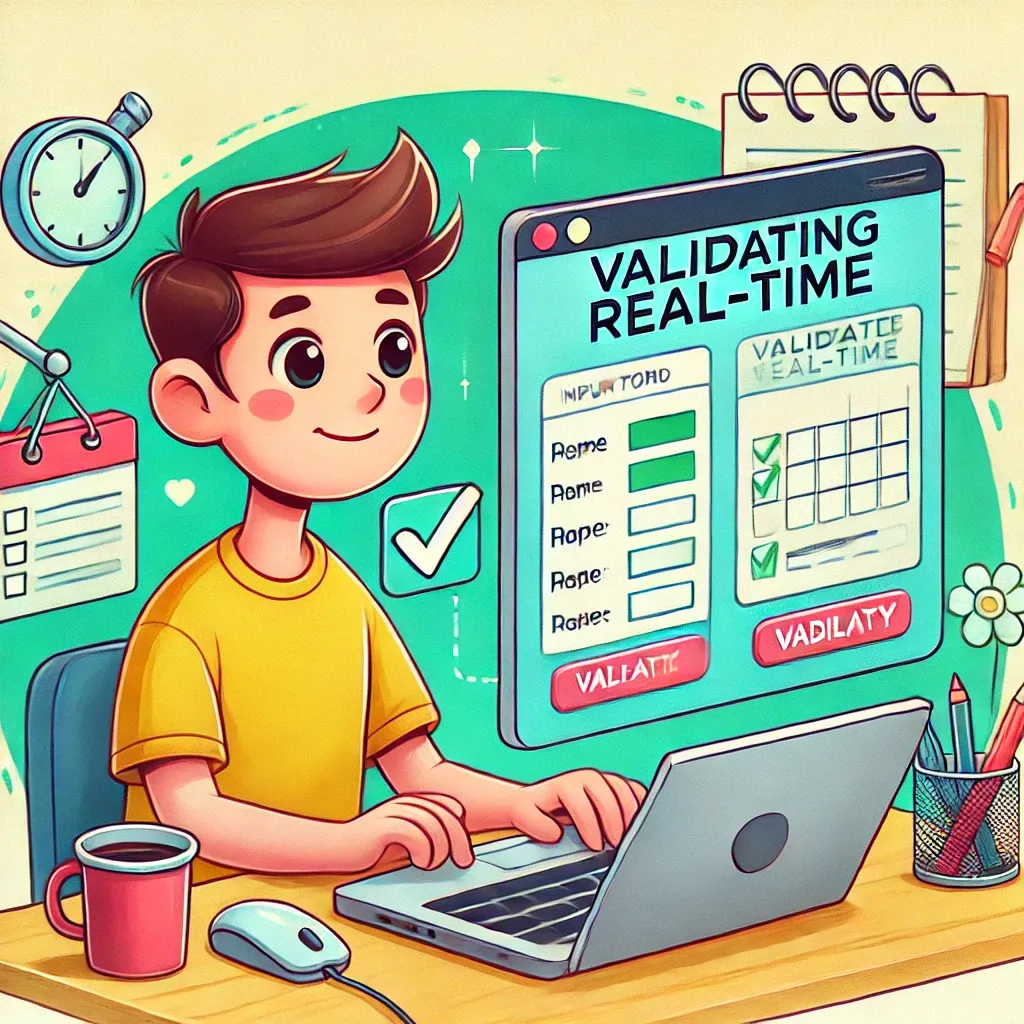
As web developers, we're always striving to create more responsive and user-friendly interfaces. With Laravel Livewire, we can take our form validations to the next level by providing instant feedback to users. In this post, we'll explore how to implement real-time validation in your Livewire components.
Understanding Real-Time Validation in Livewire
Real-time validation in Livewire allows us to validate form inputs as the user types, providing immediate feedback without the need for a full page reload or complex JavaScript.
Implementing Real-Time Validation
Let's walk through the process of setting up real-time validation in a Livewire component:
- Create a Livewire Component:
// app/Http/Livewire/CreatePost.php
use Livewire\Component;
class CreatePost extends Component
{
public $title = '';
public $content = '';
protected $rules = [
'title' => 'required|min:5',
'content' => 'required|min:20'
];
public function updated($propertyName)
{
$this->validateOnly($propertyName);
}
public function save()
{
$validatedData = $this->validate();
// Save the post...
}
public function render()
{
return view('livewire.create-post');
}
}
- Create the Blade View:
<!-- resources/views/livewire/create-post.blade.php -->
<form wire:submit.prevent="save">
<div>
<input wire:model.live.debounce.500ms="title" type="text" placeholder="Post Title">
@error('title') <span class="error">{{ $message }}</span> @enderror
</div>
<div>
<textarea wire:model.live.debounce.500ms="content" placeholder="Post Content"></textarea>
@error('content') <span class="error">{{ $message }}</span> @enderror
</div>
<button type="submit">Create Post</button>
</form>
Key Components of Real-Time Validation
- wire:model.live: This directive tells Livewire to update the property in real-time as the user types.
- debounce: Adding
debounce.500ms
prevents the validation from firing on every keystroke, improving performance. - $rules property: Defines the validation rules for your component's properties.
- updated method: This method is called whenever a property is updated, allowing you to run validation on just that property.
- @error directive: Displays validation errors in real-time as they occur.
Benefits of Real-Time Validation
- Improved User Experience: Users get immediate feedback on their input.
- Reduced Server Load: By validating in real-time, you can prevent invalid form submissions before they reach the server.
- Faster Development: Livewire handles the complexities of AJAX and JavaScript, allowing you to focus on your application logic.
Advanced Techniques
- Custom Validation Messages:
protected $messages = [
'title.required' => 'Your post needs a catchy title!',
'content.min' => 'Let\'s make the content a bit longer, shall we?'
];
- Conditional Validation:
public function updated($propertyName)
{
if($propertyName === 'title') {
$this->validateOnly($propertyName, ['title' => 'required|min:5|unique:posts']);
} else {
$this->validateOnly($propertyName);
}
}
- Complex Validation Logic:
For more complex validations, you can use custom validation rules or even call external services within your updated
method.
Performance Considerations
While real-time validation greatly enhances user experience, it's important to consider performance, especially for complex forms or slow connections. Here are some tips:
- Always use debounce on text inputs to limit the number of validation calls.
- For very complex validations, consider using
wire:model.lazy
instead ofwire:model.live
. - Optimize your backend queries in the validation process to ensure quick responses.
Conclusion
Real-time validation with Laravel Livewire offers a powerful way to enhance your forms and provide immediate feedback to users. By leveraging Livewire's features and following best practices, you can create responsive, user-friendly forms that validate data efficiently and improve overall user experience. Happy coding!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!