Mastering Rate Limiting in Laravel: Smooth Sailing for Your API and Queue Jobs
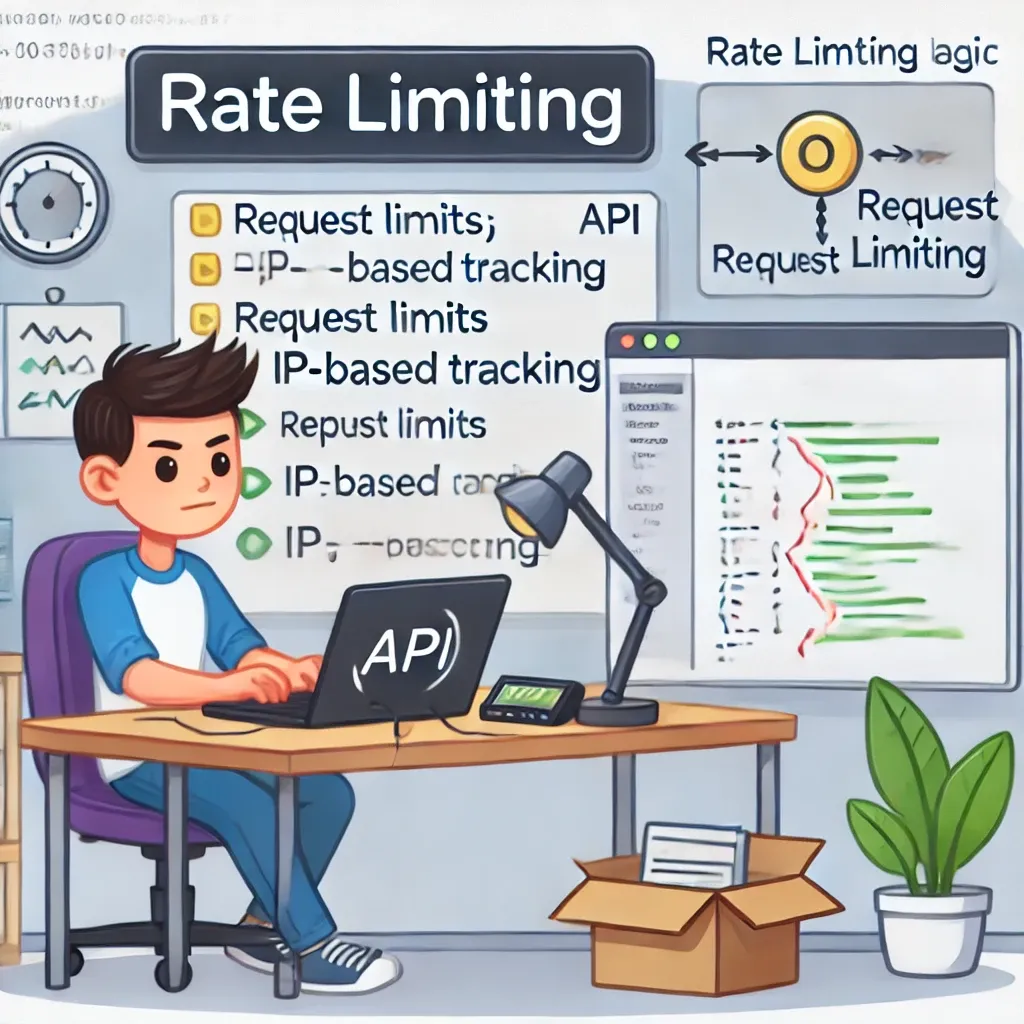
Ever worried about your API getting hammered or your queue jobs overwhelming external services? Laravel's rate limiting features have got your back! Let's dive into how you can use RateLimiter
to keep things under control.
The RateLimiter Facade
Laravel's RateLimiter
facade is your go-to tool for setting up rate limits. It's simple to use but packs a punch.
Setting Up a Rate Limit
Here's how you can set up a rate limit:
use Illuminate\Support\Facades\RateLimiter;
RateLimiter::for(
GlobalRateLimit::HUBSPOT,
fn () => Limit::perSecond(100, 10)
);
What's happening here?
- We're defining a rate limit named
GlobalRateLimit::HUBSPOT
- We're allowing 100 attempts per second
- If the limit is exceeded, we're adding a 10-second delay
Rate Limiting Queue Jobs
But wait, there's more! You can also apply rate limits to your queue jobs:
use Illuminate\Queue\Middleware\RateLimited;
public function middleware(): array
{
return [
new RateLimited(GlobalRateLimit::HUBSPOT)
];
}
This code applies the HUBSPOT
rate limit to a queue job. It's perfect for when you're hitting external APIs and need to stay within their usage limits.
Real-World Example: API Integration
Let's say you're integrating with HubSpot's API. You want to ensure you don't exceed their rate limits:
use Illuminate\Support\Facades\RateLimiter;
use App\Jobs\SyncHubSpotContacts;
class HubSpotController extends Controller
{
public function __construct()
{
RateLimiter::for('hubspot-api', function () {
return Limit::perMinute(100)->by(auth()->id());
});
}
public function syncContacts()
{
SyncHubSpotContacts::dispatch()->middleware(['throttle:hubspot-api']);
return response()->json(['message' => 'Contact sync job queued']);
}
}
class SyncHubSpotContacts implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public function middleware(): array
{
return [new RateLimited('hubspot-api')];
}
public function handle()
{
// Sync logic here
}
}
In this example:
- We set up a rate limit for HubSpot API calls
- We apply this limit to both our controller and our queue job
- This ensures we stay within HubSpot's rate limits, whether we're making direct API calls or processing jobs in the queue
Using Laravel's rate limiting features, you can keep your application running smoothly, respect API limits, and prevent any single user or process from overwhelming your system. It's a must-have for any robust Laravel application!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!