Mastering Query Debugging in Laravel: Unveiling the Power of dd, dump, and Raw SQL Methods
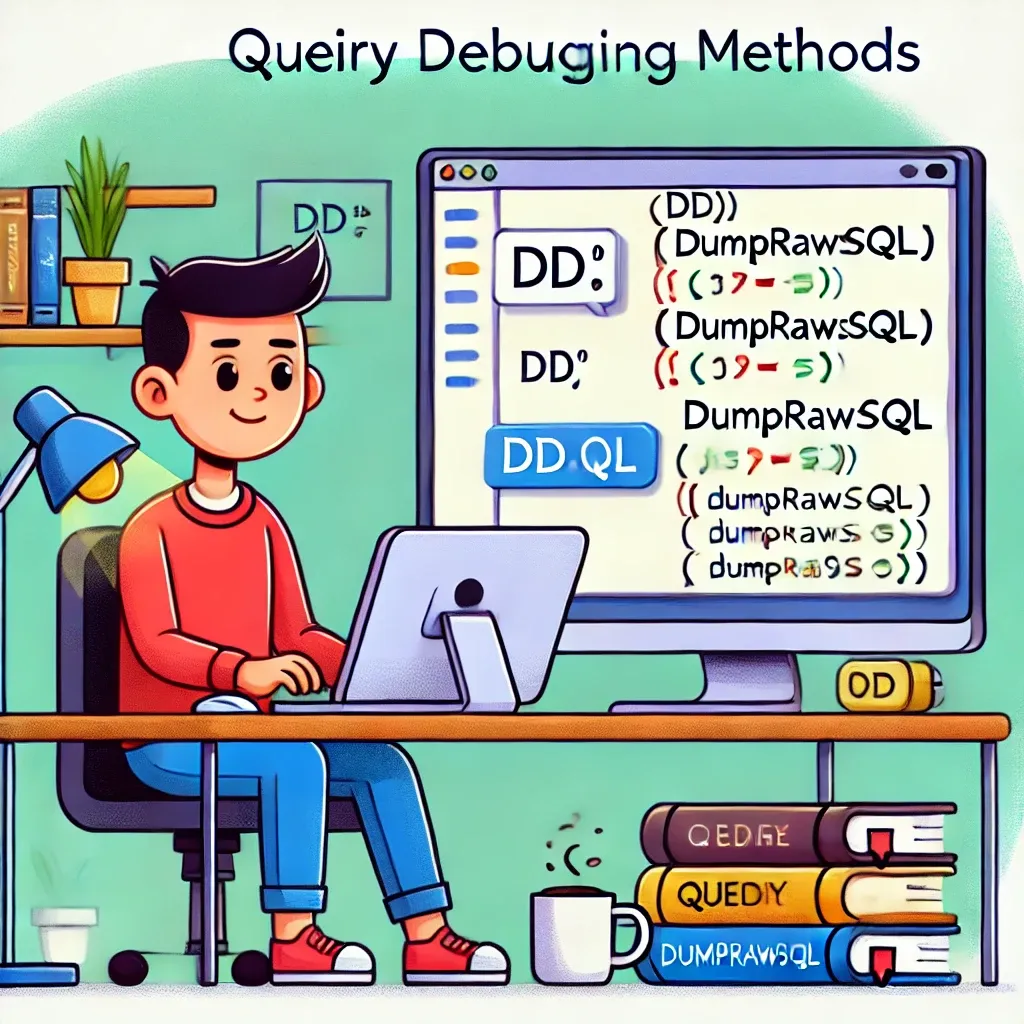
When building complex database queries in Laravel, having powerful debugging tools at your fingertips can be a game-changer. Laravel provides a set of methods that allow you to inspect your queries in detail, making the debugging process smoother and more efficient. Let's dive into these methods and see how they can enhance your development workflow.
The dd and dump Methods
Laravel's query builder offers two primary methods for debugging: dd()
and dump()
.
• dd()
(Dump and Die): This method dumps the debug information and then stops the execution of the request.
• dump()
: This method displays the debug information but allows the request to continue executing.
Here's how you can use these methods:
DB::table('users')->where('votes', '>', 100)->dd();
DB::table('users')->where('votes', '>', 100)->dump();
These methods are incredibly useful when you want to inspect the current state of your query, including the SQL that will be executed and the bindings that will be used.
Raw SQL Debugging with dumpRawSql and ddRawSql
Sometimes, seeing the raw SQL with all parameters properly substituted can provide clearer insights. Laravel offers dumpRawSql()
and ddRawSql()
for this purpose:
DB::table('users')->where('votes', '>', 100)->dumpRawSql();
DB::table('users')->where('votes', '>', 100)->ddRawSql();
These methods show you the exact SQL that will be sent to the database, with all parameter bindings in place. This can be particularly helpful when dealing with complex queries or when you need to optimize your database interactions.
Practical Applications
• Troubleshooting complex queries:
DB::table('orders')
->join('users', 'orders.user_id', '=', 'users.id')
->where('orders.status', 'pending')
->where('users.active', true)
->orderBy('orders.created_at', 'desc')
->ddRawSql();
• Debugging subqueries:
$subquery = DB::table('posts')->select('user_id')->where('created_at', '>', now()->subDays(7));
DB::table('users')
->whereIn('id', $subquery)
->dumpRawSql();
• Inspecting query builder state:
$query = DB::table('products')->where('category', 'electronics');
if ($request->has('min_price')) {
$query->where('price', '>=', $request->min_price);
}
if ($request->has('max_price')) {
$query->where('price', '<=', $request->max_price);
}
$query->dump(); // Check the state of the query before execution
$results = $query->get();
By leveraging these powerful debugging methods, you can gain deeper insights into your Laravel database queries, making it easier to optimize performance and catch potential issues early in the development process. Whether you're building complex reports, optimizing slow queries, or just learning the ins and outs of Laravel's query builder, these tools are invaluable additions to your Laravel debugging toolkit.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!