Mastering Number Manipulation in Laravel with the Number Helper
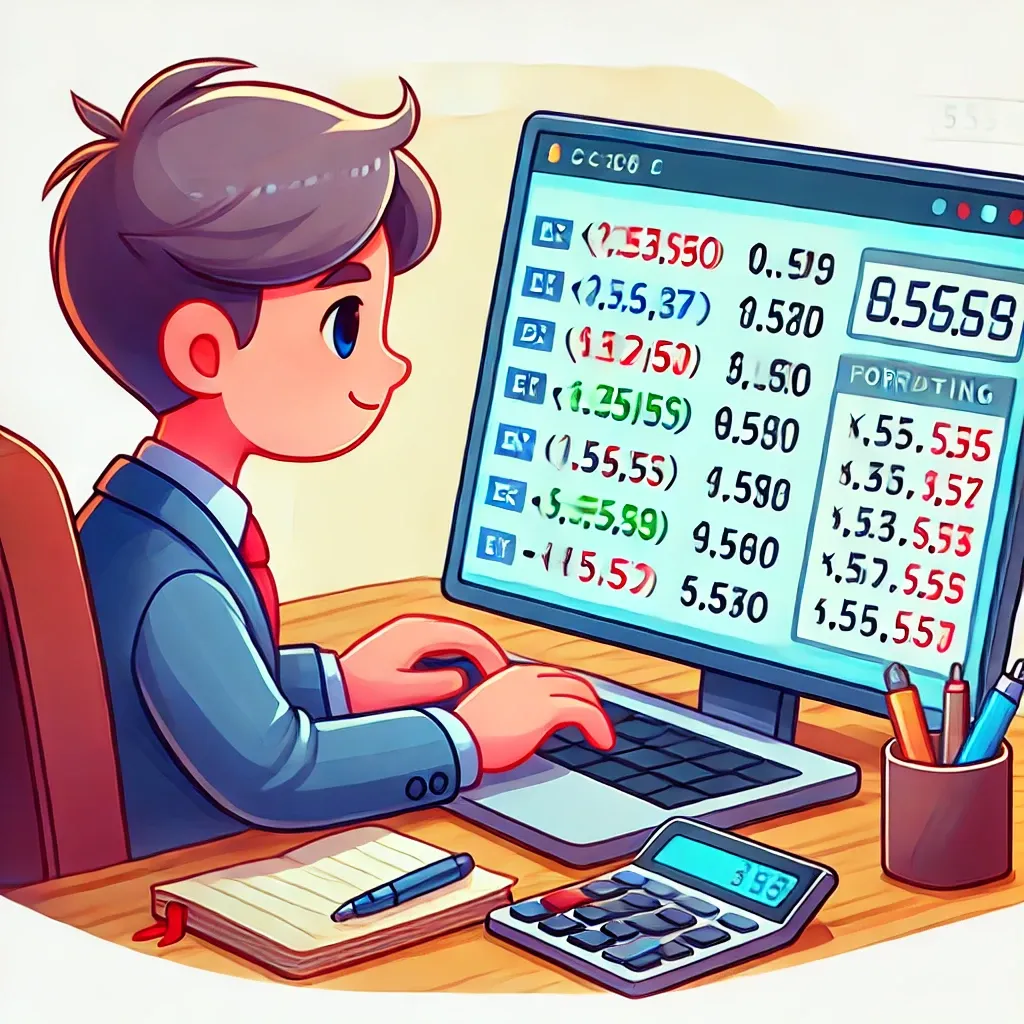
Laravel's Number helper, introduced in Laravel 9, provides a powerful and expressive way to format and manipulate numbers in your applications. Whether you're building financial tools, data-heavy applications, or simply need to present numbers in a user-friendly format, the Number helper has got you covered. Let's dive into how you can leverage this feature in your Laravel projects.
Basic Number Formatting
The format
method allows you to format numbers with precision and locale awareness:
use Illuminate\Support\Number;
$formatted = Number::format(1234567.89, decimal: 2, locale: 'en');
// Output: 1,234,567.89
You can easily adjust the decimal places and locale to suit your needs:
$formatted = Number::format(1234567.89, decimal: 2, locale: 'de');
// Output: 1.234.567,89
Percentage Formatting
For percentage values, the percentage
method is incredibly handy:
$percentage = Number::percentage(0.755, precision: 1);
// Output: 75.5%
This method automatically multiplies the value by 100 and appends the percentage symbol.
Currency Formatting
The currency
method is perfect for displaying monetary values:
$currency = Number::currency(1234.56, in: 'USD', locale: 'en_US');
// Output: $1,234.56
$currency = Number::currency(1234.56, in: 'EUR', locale: 'de_DE');
// Output: 1.234,56 €
This method respects the locale's currency formatting rules, including symbol placement and digit grouping.
Ordinal Numbers
For ordinal numbers (1st, 2nd, 3rd, etc.), use the ordinal
method:
$ordinal = Number::ordinal(23);
// Output: 23rd
File Sizes
When dealing with file sizes, the fileSize
method comes in handy:
$size = Number::fileSize(1024 * 1024);
// Output: 1.00 MB
Compact Number Formatting
For large numbers, you might want to use a more compact representation:
$compact = Number::compactCurrency(1234567, 'USD');
// Output: $1.23M
Advanced Usage: Custom Formatting
You can combine these methods with PHP's native number formatting for more complex scenarios:
$value = 1234567.89;
$formatted = Number::format($value, decimal: 2, locale: 'en');
$padded = str_pad($formatted, 15, ' ', STR_PAD_LEFT);
echo "Total: {$padded}";
// Output: Total: 1,234,567.89
Real-World Example: Financial Report
Here's a more comprehensive example of using the Number helper in a financial context:
use Illuminate\Support\Number;
class FinancialReport
{
public function generate($data, $locale = 'en_US')
{
return [
'total_revenue' => Number::currency($data['revenue'], in: 'USD', locale: $locale),
'expenses' => Number::currency($data['expenses'], in: 'USD', locale: $locale),
'profit_margin' => Number::percentage($data['revenue'] > 0 ? $data['profit'] / $data['revenue'] : 0, precision: 2),
'average_transaction' => Number::currency($data['average_transaction'], in: 'USD', locale: $locale),
'customer_growth' => Number::percentage($data['customer_growth'], precision: 1),
];
}
}
$report = new FinancialReport();
$data = [
'revenue' => 1234567.89,
'expenses' => 987654.32,
'profit' => 246913.57,
'average_transaction' => 123.45,
'customer_growth' => 0.0534,
];
$result = $report->generate($data);
This example demonstrates how the Number helper can be used to create a clean, formatted financial report with proper currency and percentage formatting.
Laravel's Number helper provides a robust and easy-to-use solution for number formatting and manipulation in your applications. By leveraging these methods, you can ensure consistent, locale-aware number presentation throughout your project, enhancing both the user experience and the maintainability of your code.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!