Mastering Multi-Channel Alerts with Laravel's Notification System
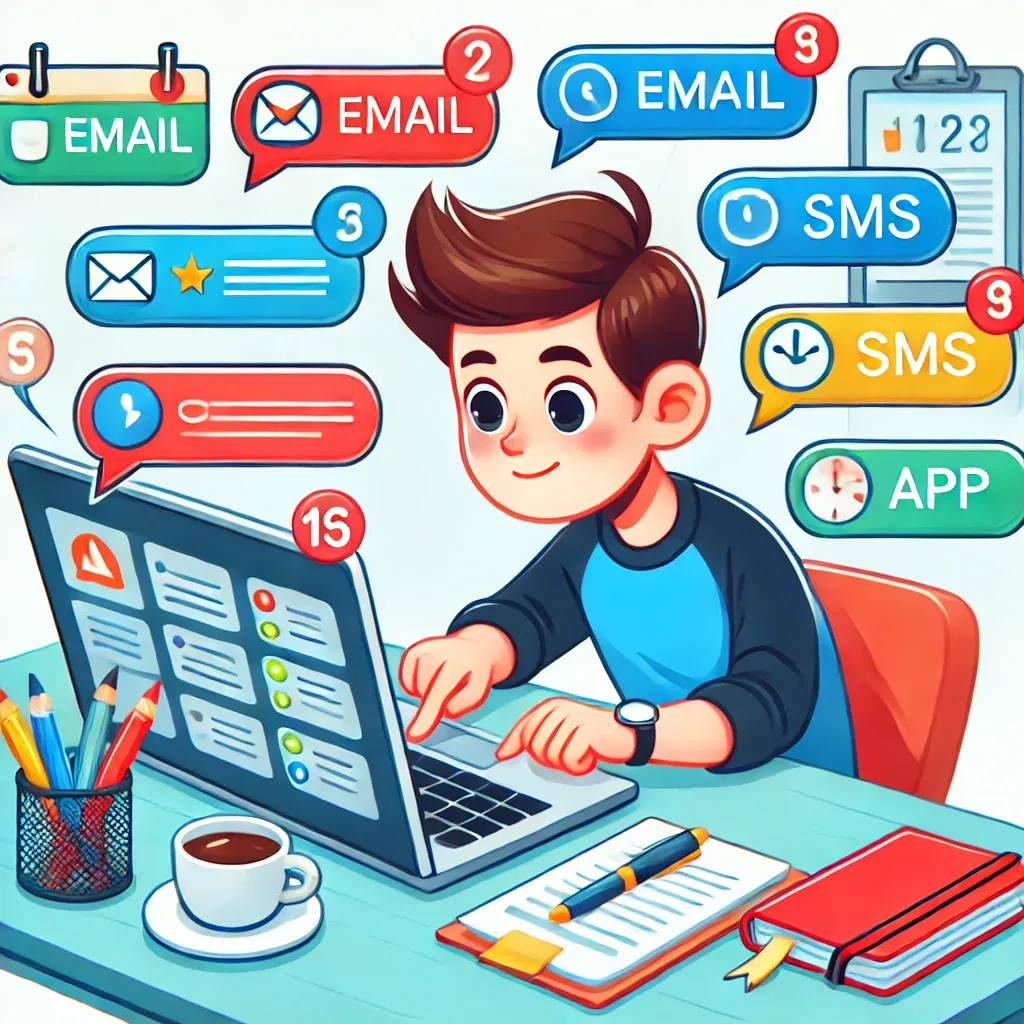
Laravel's notification system provides a powerful and flexible way to send alerts across multiple channels from a single, unified interface. This feature allows developers to easily keep users informed through various mediums such as email, SMS, Slack, and more. Let's dive into how to leverage this system effectively.
Creating a Notification
To create a notification, use the Artisan command:
php artisan make:notification InvoicePaid
This creates a new notification class:
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\Messages\MailMessage;
use Illuminate\Notifications\Messages\SlackMessage;
class InvoicePaid extends Notification
{
public function via($notifiable)
{
return ['mail', 'slack', 'database'];
}
public function toMail($notifiable)
{
return (new MailMessage)
->subject('Invoice Paid')
->markdown('mail.invoice.paid', ['invoice' => $this->invoice]);
}
public function toSlack($notifiable)
{
return (new SlackMessage)
->content('An invoice has been paid!');
}
public function toDatabase($notifiable)
{
return [
'invoice_id' => $this->invoice->id,
'amount' => $this->invoice->amount,
];
}
}
Sending Notifications
To send a notification:
$user->notify(new InvoicePaid($invoice));
Or dispatch it to the queue:
$user->notifyNow(new InvoicePaid($invoice));
Customizing Delivery Channels
You can customize delivery channels based on the notifiable entity:
public function via($notifiable)
{
return $notifiable->prefers_sms ? ['nexmo'] : ['mail', 'database'];
}
Creating Custom Channels
For app-specific notification methods, create a custom channel:
namespace App\Channels;
use Illuminate\Notifications\Notification;
class FirebaseChannel
{
public function send($notifiable, Notification $notification)
{
$message = $notification->toFirebase($notifiable);
// Send notification via Firebase
}
}
Then use it in your notification:
public function via($notifiable)
{
return [FirebaseChannel::class];
}
public function toFirebase($notifiable)
{
return FirebaseMessage::create()
->withTitle('New Message')
->withBody('You have a new message.');
}
On-Demand Notifications
Send notifications to users who are not stored as notifiable entities:
Notification::route('mail', 'taylor@example.com')
->route('nexmo', '5555555555')
->notify(new InvoicePaid($invoice));
Notification Events
Laravel fires events during the notification sending process:
namespace App\Listeners;
use Illuminate\Notifications\Events\NotificationSent;
class LogNotification
{
public function handle(NotificationSent $event)
{
// $event->channel, $event->notifiable, $event->notification, $event->response
}
}
Bulk Notifications
To send notifications to multiple users efficiently:
Notification::send(User::all(), new InvoicePaid($invoice));
Localizing Notifications
For multi-language applications, you can localize your notifications:
$user->notify((new InvoicePaid($invoice))->locale('es'));
Testing Notifications
Laravel provides helpers for testing notifications:
use Illuminate\Support\Facades\Notification;
public function test_invoice_paid_notification_is_sent()
{
Notification::fake();
// Perform order payment...
Notification::assertSentTo(
$user,
InvoicePaid::class,
function ($notification, $channels) use ($order) {
return $notification->invoice->id === $order->id;
}
);
}
Laravel's notification system offers a robust and extensible way to handle multi-channel alerts in your application. By leveraging this feature, you can create a more engaging and informative experience for your users across various communication platforms.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!