Mastering Laravel Pagination: Customizing Link Display with onEachSide()
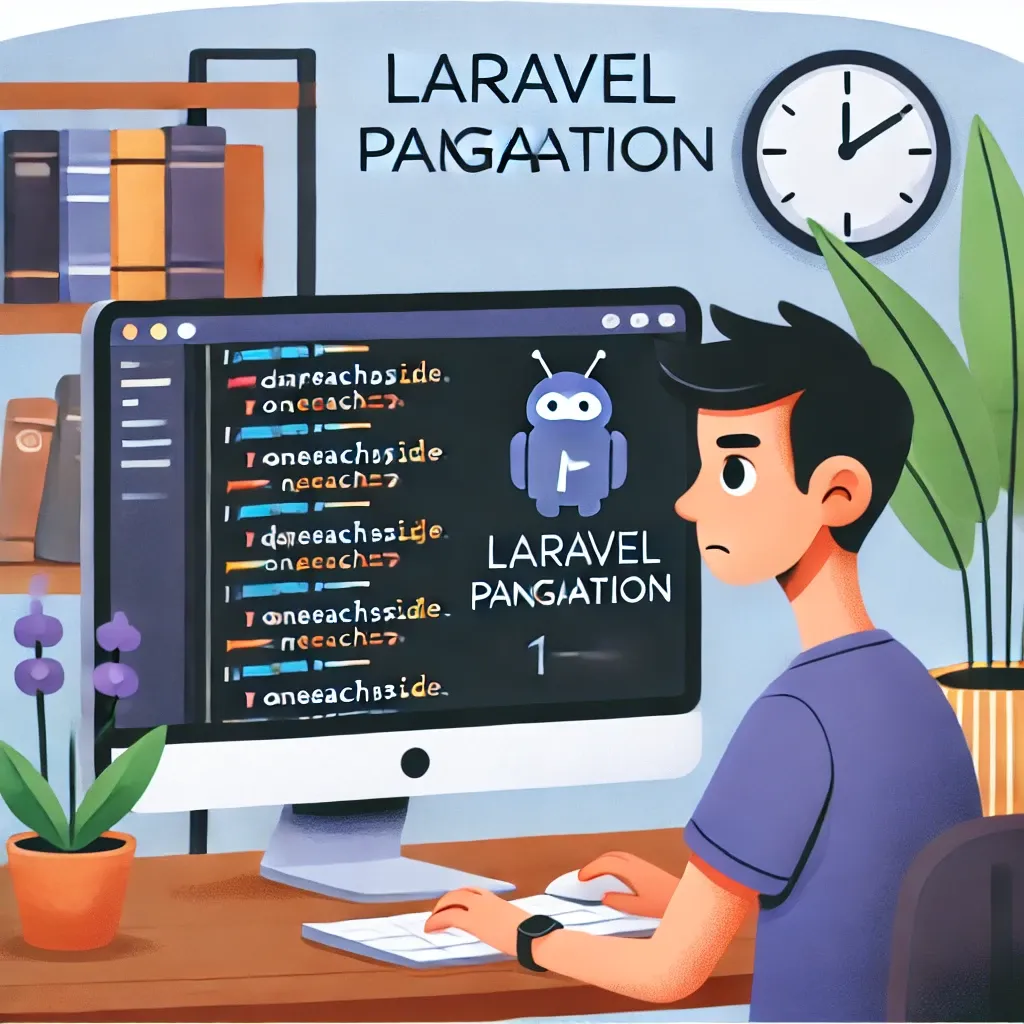
As Laravel developers, we often work with large datasets that require pagination. Laravel's built-in pagination system is powerful and flexible, but did you know you can fine-tune the number of links displayed? Let's dive into the onEachSide()
method and see how it can enhance your pagination experience.
Understanding Laravel's Default Pagination
By default, when you use Laravel's pagination links, you'll see something like this:
1 2 3 4 5 ... 10
This display shows the current page, two pages on each side, and then skips to the last page. But what if you want more (or fewer) links?
Enter the onEachSide() Method
Laravel provides a simple yet powerful method called onEachSide()
that allows you to control how many additional links are displayed on each side of the current page. Here's how you use it:
{{ $users->onEachSide(5)->links() }}
In this example, we're telling Laravel to display 5 links on each side of the current page. So if you're on page 6 of a 20-page result set, you might see something like this:
1 2 3 4 5 6 7 8 9 10 11 ... 20
Practical Example
Let's see how this might look in a real Laravel controller and view:
// UsersController.php
public function index()
{
$users = User::paginate(15);
return view('users.index', compact('users'));
}
// users/index.blade.php
<div class="users-list">
@foreach ($users as $user)
<div class="user-item">{{ $user->name }}</div>
@endforeach
</div>
<div class="pagination-links">
{{ $users->onEachSide(5)->links() }}
</div>
In this example, we're displaying 15 users per page and showing 5 links on each side of the current page in our pagination.
Remember, the key to great pagination is finding the right balance between providing enough context and not overwhelming the user. Experiment with different values for onEachSide()
to find what works best for your specific use case.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!