Mastering Job Queue Pacing with Laravel's Sleep Helper
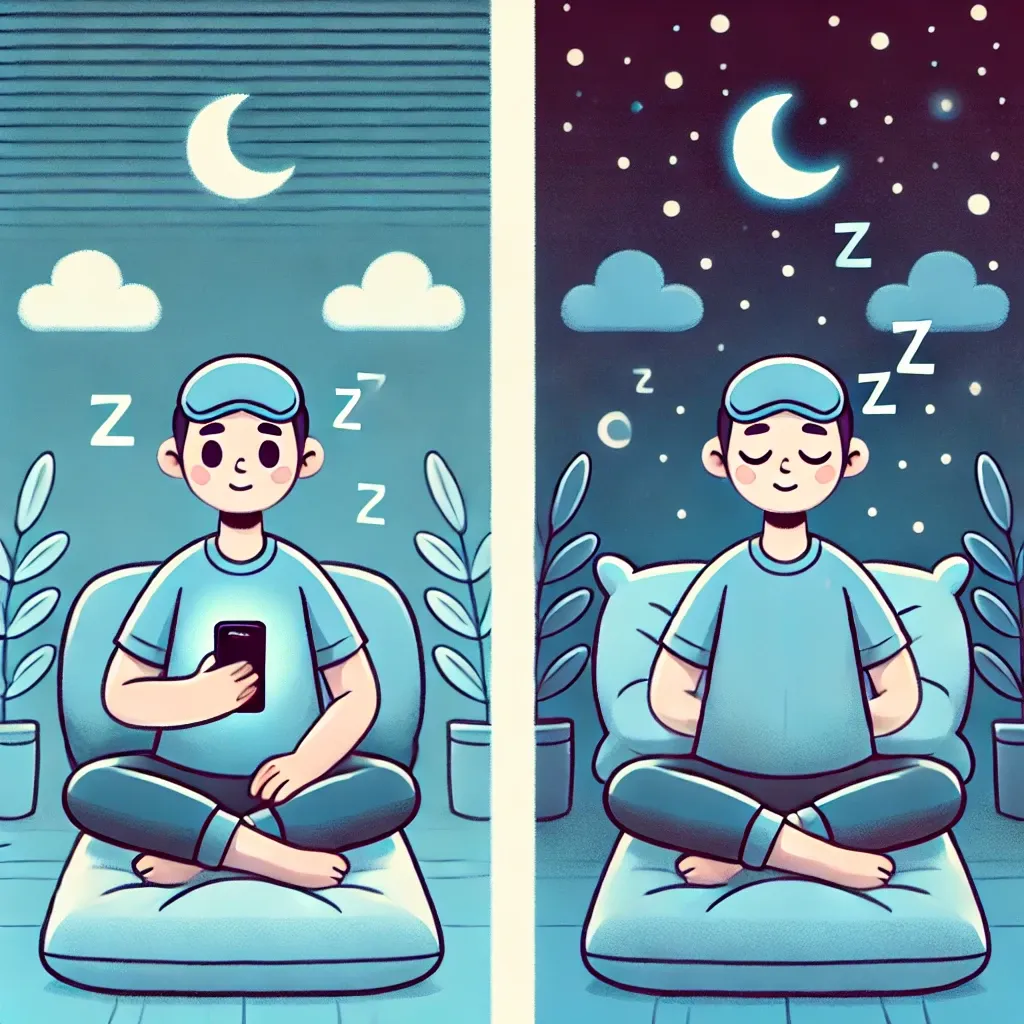
Laravel's Sleep helper provides a clean and expressive way to introduce controlled pauses in your code execution. This feature is particularly useful when working with job queues, especially for tasks that interact with rate-limited APIs or resource-intensive operations.
Understanding the Sleep Helper
The Sleep helper allows you to pause the execution of your code for a specified duration. It's part of Laravel's Illuminate\Support\Facades\Sleep
facade and offers a fluent interface for readability.
Using Sleep in Queue Jobs
Let's look at a practical example of using the Sleep helper in a job that fetches data from an external API:
use Illuminate\Support\Facades\Http;
use Illuminate\Support\Facades\Sleep;
use Illuminate\Contracts\Queue\ShouldQueue;
class FetchExternalApiData implements ShouldQueue
{
public $timeout = 120;
private $apiUrl = 'https://api.example.com/data';
public function handle()
{
$response = Http::get($this->apiUrl);
$this->processData($response->json());
// Check rate limit headers
$remainingRequests = $response->header('X-RateLimit-Remaining');
$resetTime = $response->header('X-RateLimit-Reset');
if ($remainingRequests <= 1) {
$sleepDuration = $resetTime - now()->timestamp;
Sleep::for($sleepDuration)->seconds();
} else {
Sleep::for(0.5)->seconds();
}
}
private function processData($data)
{
// Process the fetched data
}
}
In this example, we're using the Sleep helper to manage our API requests responsibly:
- We make a request to the external API.
- After processing the data, we check the rate limit headers.
- If we're close to the rate limit, we pause execution until the limit resets.
- If we're not close to the limit, we add a small delay to pace our requests.
Benefits of Using Sleep in Queues
- Respect Rate Limits: Easily adhere to API rate limits by dynamically adjusting your request frequency.
- Resource Management: Prevent overwhelming your own server or database by pacing intensive operations.
- Improved Reliability: Reduce the chances of jobs failing due to rate limit errors.
Advanced Usage
You can extend this concept further:
use Illuminate\Support\Facades\Redis;
public function handle()
{
// ... API request and processing ...
$key = 'api_request_count';
$count = Redis::incr($key);
if ($count >= 100) {
Sleep::for(60)->seconds();
Redis::del($key);
} else {
$dynamicSleep = min(1 + ($count / 20), 5);
Sleep::for($dynamicSleep)->seconds();
}
}
This approach uses Redis to keep track of request counts across multiple job executions and dynamically adjusts the sleep duration based on the number of recent requests.
Testing Considerations
When writing tests for jobs that use the Sleep helper, you may want to skip the actual sleep to speed up your tests:
use Illuminate\Support\Facades\Sleep;
public function test_api_fetch_job()
{
Sleep::fake();
// Dispatch and assert job logic
Sleep::assertSlept(2);
}
The Sleep helper in Laravel provides a powerful tool for managing the pacing of your queued jobs. By using it effectively, you can create more robust, efficient, and API-friendly applications.