Mastering Job Lifecycle with Laravel Job Events
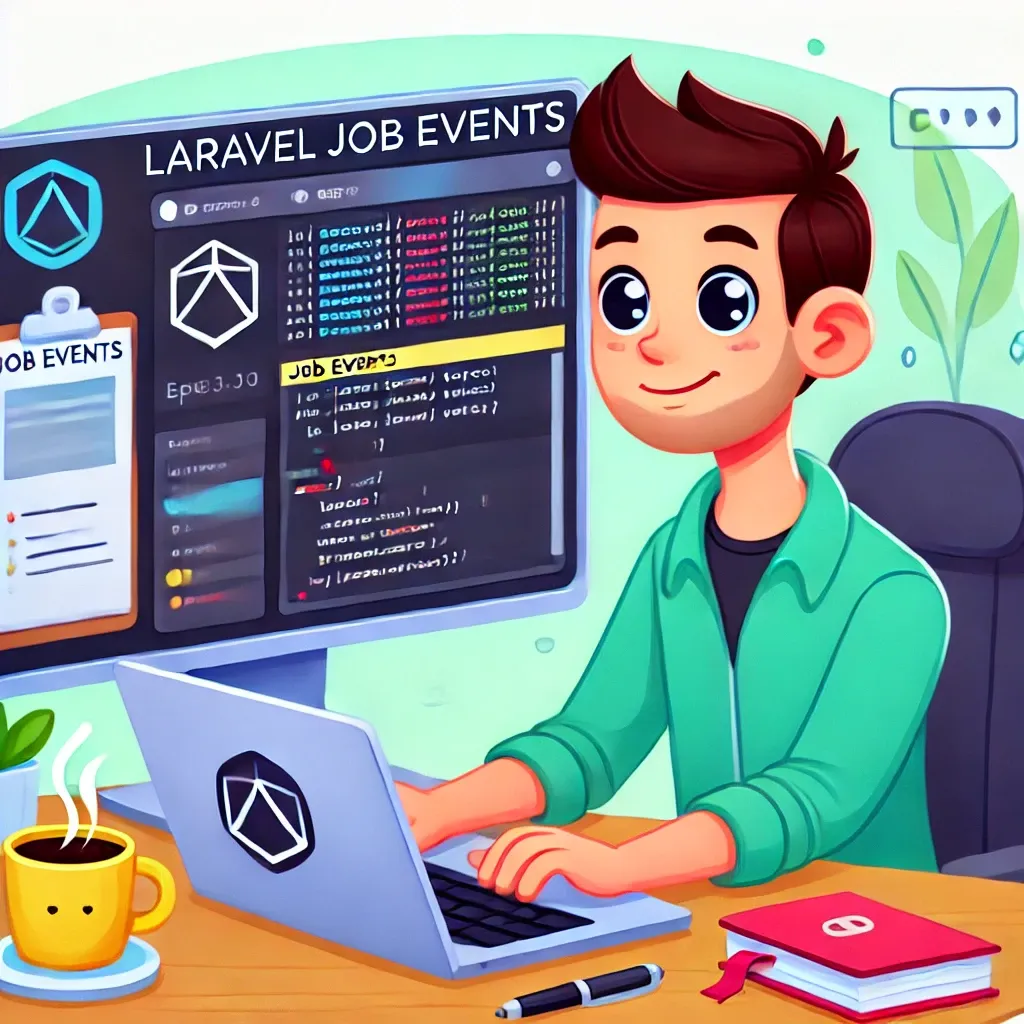
Laravel's queue system becomes even more powerful when combined with Job Events, allowing you to monitor and react to various stages of a job's lifecycle. Let's explore how to leverage these events for advanced job handling and monitoring.
Listening for Job Events
To start listening for job events, register listeners in your EventServiceProvider
:
// In app/Providers/EventServiceProvider.php
protected $listen = [
'Illuminate\Queue\Events\JobProcessed' => [
'App\Listeners\LogSuccessfulJob',
],
'Illuminate\Queue\Events\JobFailed' => [
'App\Listeners\NotifyTeamOfFailedJob',
],
];
Implementing Event Listeners
Here's an example of a listener for successful jobs:
// In app/Listeners/LogSuccessfulJob.php
namespace App\Listeners;
use Illuminate\Queue\Events\JobProcessed;
use Illuminate\Support\Facades\Log;
class LogSuccessfulJob
{
public function handle(JobProcessed $event)
{
Log::info('Job processed successfully', [
'job' => get_class($event->job->resolveName()),
'payload' => $event->job->payload(),
]);
}
}
And for failed jobs:
// In app/Listeners/NotifyTeamOfFailedJob.php
namespace App\Listeners;
use Illuminate\Queue\Events\JobFailed;
use Illuminate\Support\Facades\Notification;
use App\Notifications\JobFailedNotification;
class NotifyTeamOfFailedJob
{
public function handle(JobFailed $event)
{
Notification::route('slack', 'your-slack-webhook-url')
->notify(new JobFailedNotification($event->job, $event->exception));
}
}
Custom Notifications
Create a simple notification for failed jobs:
// In app/Notifications/JobFailedNotification.php
namespace App\Notifications;
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\SlackMessage;
class JobFailedNotification extends Notification
{
protected $job;
protected $exception;
public function __construct($job, $exception)
{
$this->job = $job;
$this->exception = $exception;
}
public function toSlack($notifiable)
{
return (new SlackMessage)
->error()
->content('A job failed in the application.')
->attachment(function ($attachment) {
$attachment
->title(get_class($this->job->resolveName()))
->content($this->exception->getMessage());
});
}
}
Job-Specific Events
For more granular control, dispatch custom events within your jobs:
namespace App\Jobs;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use App\Events\OrderShipmentProcessed;
class ProcessOrderShipment implements ShouldQueue
{
use Dispatchable;
protected $order;
public function __construct($order)
{
$this->order = $order;
}
public function handle()
{
// Process shipment...
event(new OrderShipmentProcessed($this->order));
}
}
You can then listen for these custom events in your EventServiceProvider
.
By leveraging Laravel's Job Events, you can create a robust monitoring and handling system for your queued jobs. This helps in debugging, maintaining your application, and allows for more complex workflows in your job processing pipeline.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!