Mastering Interactive UIs with Livewire's wire:click.prevent
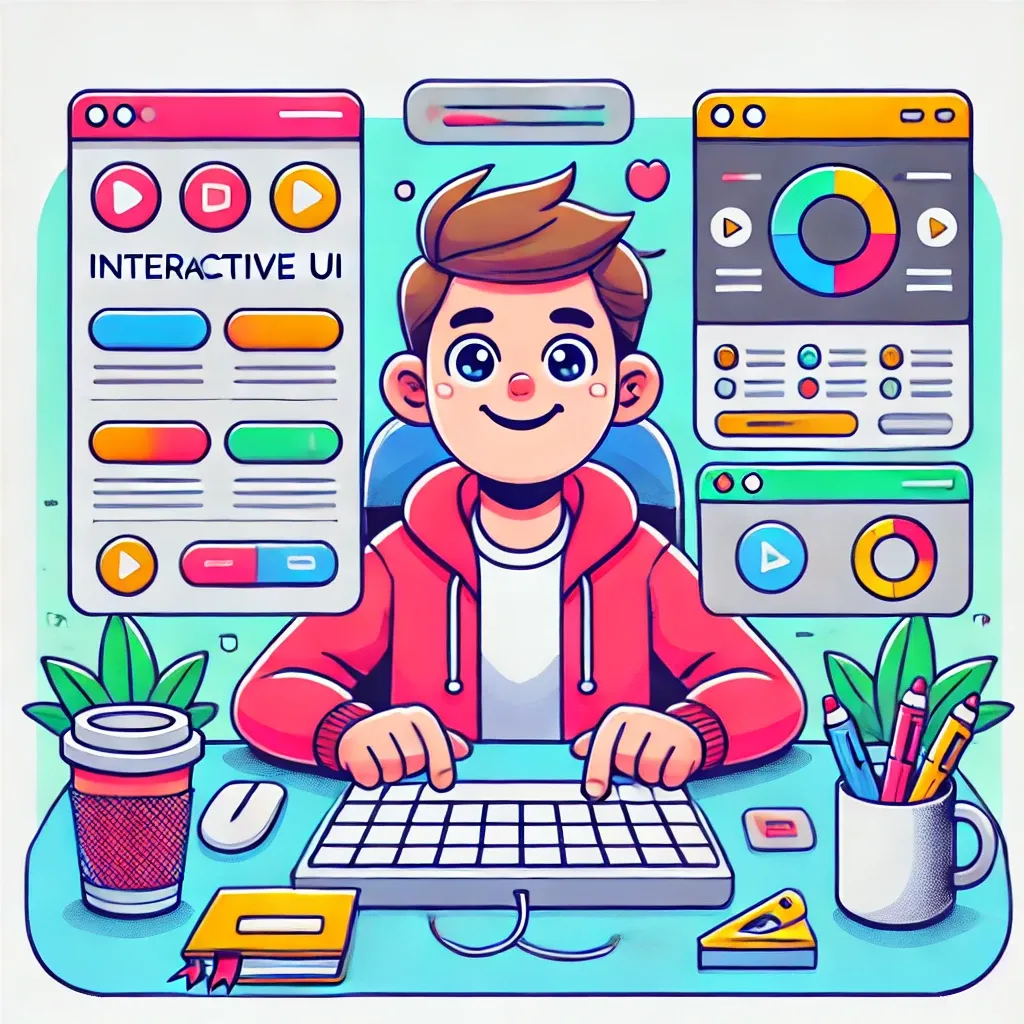
As Laravel developers, we're always looking for ways to create more interactive and responsive user interfaces. Livewire, Laravel's full-stack framework for building dynamic interfaces, offers a powerful feature that can help us do just that: wire:click.prevent. In this post, we'll explore how to use this directive to create smooth, JavaScript-free interactivity in your Laravel applications.
What is wire:click.prevent?
wire:click.prevent is a Livewire directive that allows you to handle click events on elements without triggering a full page reload. It's particularly useful for forms and interactive elements where you want to perform an action without navigating away from the current page.
How to Use wire:click.prevent
Here's a basic example of how to use wire:click.prevent:
// resources/views/livewire/user-form.blade.php
<form>
<input type="text" wire:model="name">
<button wire:click.prevent="saveName">Save Name</button>
</form>
// app/Http/Livewire/UserForm.php
class UserForm extends Component
{
public $name = '';
public function saveName()
{
$this->validate([
'name' => 'required|min:2'
]);
auth()->user()->update(['name' => $this->name]);
session()->flash('message', 'Name updated successfully!');
}
public function render()
{
return view('livewire.user-form');
}
}
Let's break this down:
- In the Blade template, we use wire:click.prevent on the button to trigger the saveName method when clicked.
- The prevent modifier stops the default form submission behavior.
- In the Livewire component, we define the saveName method that handles the validation and updating of the user's name.
- We use session()->flash to show a success message after the update.
Benefits of Using wire:click.prevent
- Improved User Experience: Actions are performed without full page reloads, making the application feel more responsive.
- Simplified JavaScript: You can create interactive interfaces without writing custom JavaScript.
- Server-Side Validation: Easily perform server-side validation before processing the action.
- Seamless Integration: Works perfectly with other Livewire features like real-time validation and data binding.
Real-World Example: Todo List Application
Let's consider a more complex real-world scenario where wire:click.prevent can be incredibly useful: a todo list application with inline editing and status toggling.
// resources/views/livewire/todo-list.blade.php
<div>
@foreach($todos as $index => $todo)
<div>
<input type="checkbox" wire:click.prevent="toggleComplete({{ $todo->id }})"
{{ $todo->completed ? 'checked' : '' }}>
<span wire:click.prevent="editTodo({{ $index }})">
{{ $todo->title }}
</span>
@if($editingIndex === $index)
<input type="text" wire:model="editingTitle"
wire:keydown.enter.prevent="saveTodo">
<button wire:click.prevent="saveTodo">Save</button>
@endif
</div>
@endforeach
</div>
// app/Http/Livewire/TodoList.php
class TodoList extends Component
{
public $todos;
public $editingIndex = null;
public $editingTitle = '';
public function mount()
{
$this->todos = auth()->user()->todos;
}
public function toggleComplete($id)
{
$todo = Todo::find($id);
$todo->completed = !$todo->completed;
$todo->save();
}
public function editTodo($index)
{
$this->editingIndex = $index;
$this->editingTitle = $this->todos[$index]->title;
}
public function saveTodo()
{
$this->validate([
'editingTitle' => 'required|min:3'
]);
$this->todos[$this->editingIndex]->update(['title' => $this->editingTitle]);
$this->editingIndex = null;
}
public function render()
{
return view('livewire.todo-list');
}
}
In this example:
- We use wire:click.prevent on the checkbox to toggle the completion status of a todo item.
- Clicking on a todo title allows inline editing, triggered by wire:click.prevent.
- The save button for editing also uses wire:click.prevent to update the todo title.
- We even use wire:keydown.enter.prevent to allow saving by pressing the Enter key.
This approach creates a highly interactive todo list where users can toggle completion and edit titles without any page reloads, all while maintaining server-side validation and data integrity.
Conclusion
Livewire's wire:click.prevent directive is a powerful tool for creating interactive, responsive user interfaces in your Laravel applications. By allowing you to handle click events without page reloads, it enables you to build complex, dynamic interfaces with ease.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!