Mastering Input Absence in Laravel: missing() and whenMissing()
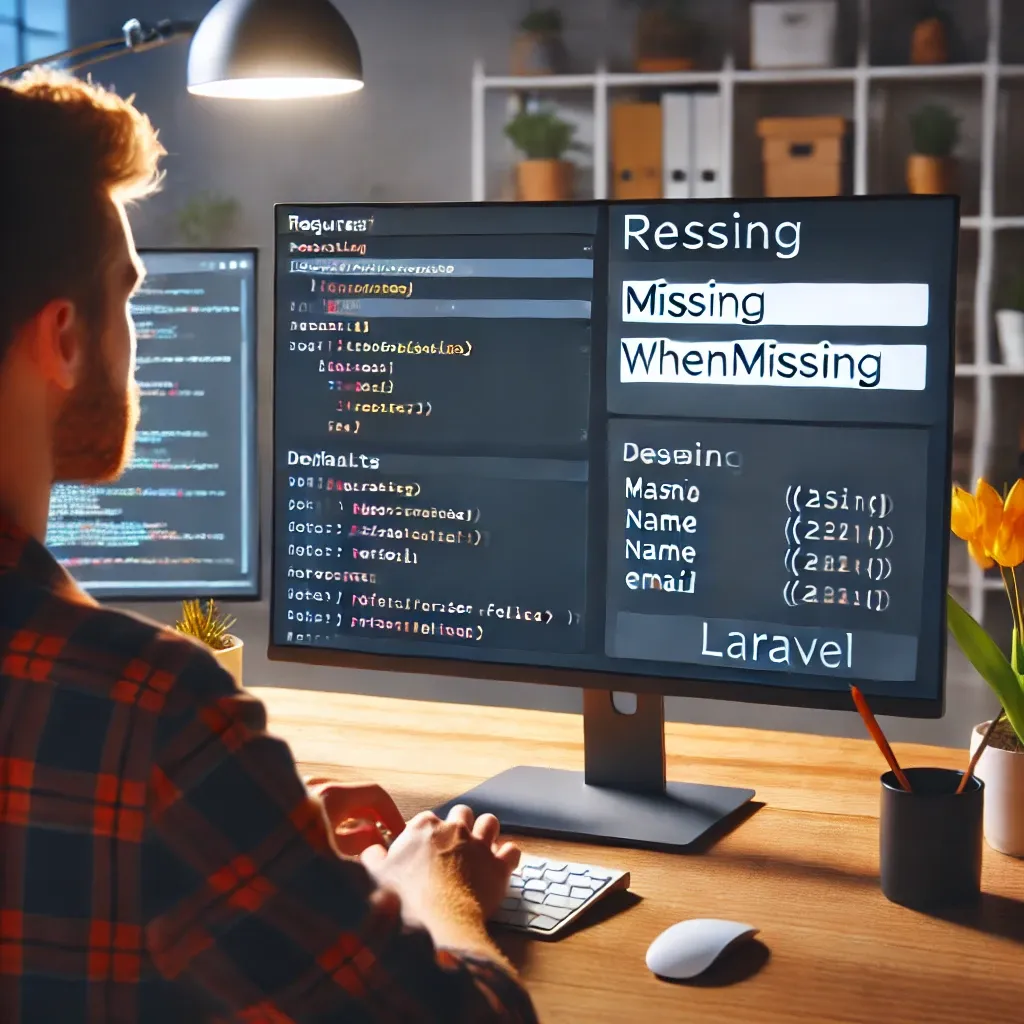
In web development, handling missing input fields is just as important as processing present ones. Laravel, with its developer-friendly approach, offers two powerful methods to deal with absent request data: missing()
and whenMissing()
. These methods provide a clean and expressive way to handle scenarios where certain inputs might not be provided. Let's explore how these features can enhance your Laravel applications.
Understanding missing() and whenMissing()
missing()
: This method checks if a given key is absent from the request.whenMissing()
: This method executes a closure if a specified key is missing from the request, with an optional second closure for when the key is present.
Using missing()
The missing()
method is straightforward:
if ($request->missing('name')) {
// The 'name' input is not present in the request
}
Using whenMissing()
The whenMissing()
method offers more flexibility:
$request->whenMissing('name', function () {
// This runs when 'name' is missing
}, function () {
// This runs when 'name' is present (optional)
});
Real-Life Example
Let's consider a scenario where we're creating a user profile. We want to set default values for missing fields and perform different actions based on whether certain fields are present or not.
Here's how we might implement this using missing()
and whenMissing()
:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function createProfile(Request $request)
{
$userData = [];
if ($request->missing('name')) {
$userData['name'] = 'Anonymous User';
} else {
$userData['name'] = $request->name;
}
$request->whenMissing('email',
function () use (&$userData) {
$userData['email'] = 'noemail@example.com';
$userData['email_verified'] = false;
},
function () use (&$userData, $request) {
$userData['email'] = $request->email;
$userData['email_verified'] = true;
}
);
$user = User::create($userData);
$request->whenMissing('bio',
fn() => $user->sendBioReminder(),
fn() => $user->update(['bio' => $request->bio])
);
return response()->json($user, 201);
}
}
In this example, we're using missing()
and whenMissing()
to:
- Set a default name if it's missing
- Handle email presence/absence, setting a default email and verification status
- Send a reminder or update the bio based on its presence
Here's what the input and output might look like:
// POST /api/users
// Input (minimal)
{
"name": "John Doe"
}
// Output
{
"id": 1,
"name": "John Doe",
"email": "noemail@example.com",
"email_verified": false,
"bio": null,
"created_at": "2023-06-15T17:30:00.000000Z",
"updated_at": "2023-06-15T17:30:00.000000Z"
}
// Input (complete)
{
"name": "Jane Smith",
"email": "jane@example.com",
"bio": "Web developer and coffee enthusiast"
}
// Output
{
"id": 2,
"name": "Jane Smith",
"email": "jane@example.com",
"email_verified": true,
"bio": "Web developer and coffee enthusiast",
"created_at": "2023-06-15T17:35:00.000000Z",
"updated_at": "2023-06-15T17:35:00.000000Z"
}
By leveraging missing()
and whenMissing()
, you can create more robust and flexible input handling in your Laravel applications. These methods shine when dealing with optional fields, allowing you to provide sensible defaults and perform conditional actions based on the presence or absence of specific inputs.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!