Mastering HTTP Method Handling in Laravel: A Deep Dive
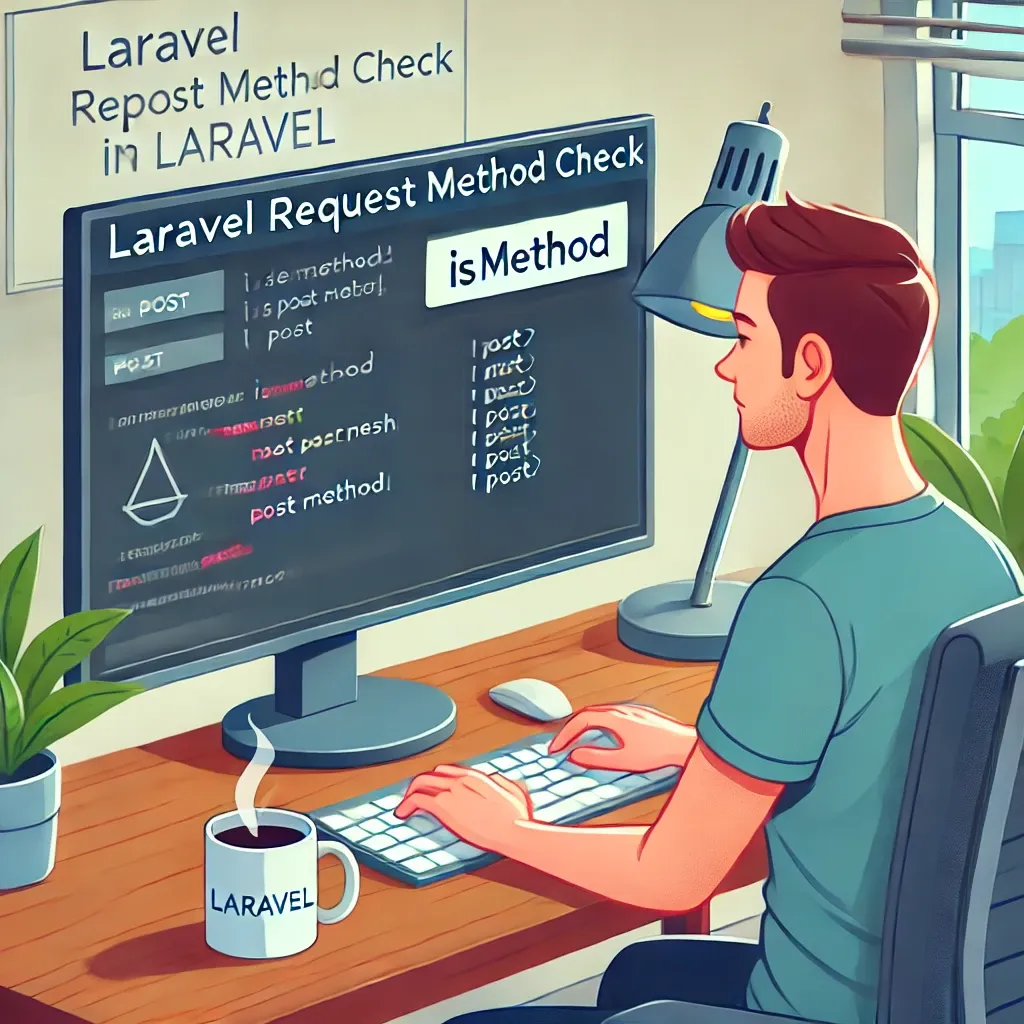
When building web applications, understanding and correctly handling different HTTP methods is crucial. Laravel provides simple yet powerful ways to work with HTTP methods in incoming requests. In this post, we'll explore how to retrieve the HTTP verb and verify specific methods using Laravel's Request object.
Understanding HTTP Method Handling in Laravel
Laravel's Request object offers two main methods for working with HTTP verbs:
method()
: Returns the HTTP verb for the request.isMethod($method)
: Checks if the HTTP verb matches a given string.
Let's dive deeper into each method:
The method() Method
The method()
method simply returns the HTTP verb of the current request:
$method = $request->method();
This will return strings like 'GET', 'POST', 'PUT', 'DELETE', etc.
The isMethod() Method
The isMethod()
method allows you to check if the current request matches a specific HTTP verb:
if ($request->isMethod('post')) {
// This code will only run for POST requests
}
Real-Life Example
Let's consider a scenario where we're building a RESTful API for a blog application. We'll use these methods to handle different operations based on the HTTP method.
Here's how we might structure a controller to handle various HTTP methods for a Post resource:
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function handle(Request $request, $id = null)
{
if ($request->isMethod('get')) {
return $this->handleGet($request, $id);
} elseif ($request->isMethod('post')) {
return $this->handlePost($request);
} elseif ($request->isMethod('put')) {
return $this->handlePut($request, $id);
} elseif ($request->isMethod('delete')) {
return $this->handleDelete($id);
}
return response()->json(['error' => 'Method not allowed'], 405);
}
private function handleGet(Request $request, $id = null)
{
if ($id) {
return Post::findOrFail($id);
}
return Post::all();
}
private function handlePost(Request $request)
{
$post = Post::create($request->all());
return response()->json($post, 201);
}
private function handlePut(Request $request, $id)
{
$post = Post::findOrFail($id);
$post->update($request->all());
return $post;
}
private function handleDelete($id)
{
Post::findOrFail($id)->delete();
return response()->json(null, 204);
}
}
Now, let's see how this might work in practice:
// In routes/api.php
Route::match(['get', 'post', 'put', 'delete'], '/posts/{id?}', [PostController::class, 'handle']);
Here's what the output might look like for different requests:
// GET /api/posts
[
{
"id": 1,
"title": "First Post",
"content": "This is the first post"
},
{
"id": 2,
"title": "Second Post",
"content": "This is the second post"
}
]
// POST /api/posts
{
"id": 3,
"title": "New Post",
"content": "This is a new post",
"created_at": "2023-06-15T10:00:00.000000Z",
"updated_at": "2023-06-15T10:00:00.000000Z"
}
// PUT /api/posts/3
{
"id": 3,
"title": "Updated Post",
"content": "This post has been updated",
"created_at": "2023-06-15T10:00:00.000000Z",
"updated_at": "2023-06-15T10:05:00.000000Z"
}
// DELETE /api/posts/3
// (204 No Content)
// PATCH /api/posts/3
{
"error": "Method not allowed"
}
In this example, we're using isMethod()
to route the request to the appropriate handler method based on the HTTP verb. This approach allows us to handle all operations for a resource in a single controller method, while still maintaining clean, separated logic for each operation.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!