Mastering Form Input Persistence in Laravel with Flash Methods
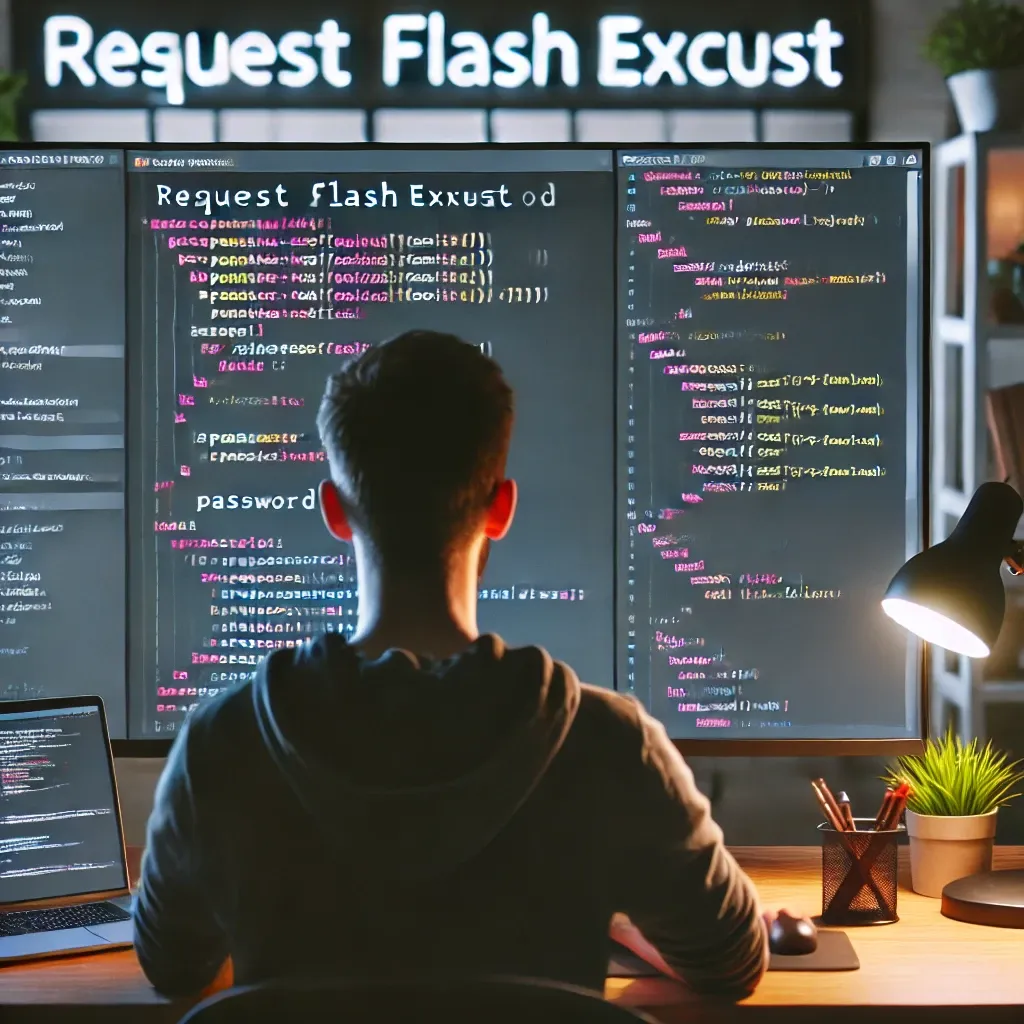
When building web applications, preserving form input across requests is a common requirement, especially when dealing with validation errors or multi-step forms. Laravel provides a set of powerful "flash" methods on the Request object to make this task effortless. Let's dive into how you can use flash()
, flashOnly()
, and flashExcept()
to enhance user experience in your Laravel applications.
Understanding Flash Methods
Laravel offers three main methods for flashing input data to the session:
flash()
: Flashes all current input to the session.flashOnly()
: Flashes only the specified input fields to the session.flashExcept()
: Flashes all input to the session except for the specified fields.
Using flash()
The flash()
method is the simplest way to persist all input:
$request->flash();
This will make all input data available in the next request via the old()
helper function.
Using flashOnly()
When you want to persist only specific fields, use flashOnly()
:
$request->flashOnly(['username', 'email']);
This is useful when you want to be explicit about which fields should be persisted.
Using flashExcept()
To persist all input except for certain fields (like passwords), use flashExcept()
:
$request->flashExcept('password');
This ensures sensitive information doesn't persist in the session.
Real-Life Example
Let's consider a scenario where we're handling a user registration form with validation. We want to persist the input if validation fails, but we don't want to flash the password field for security reasons.
Here's how we might implement this in a controller:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
class RegistrationController extends Controller
{
public function register(Request $request)
{
$validated = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8|confirmed',
]);
if ($validated) {
$user = User::create([
'name' => $validated['name'],
'email' => $validated['email'],
'password' => Hash::make($validated['password']),
]);
auth()->login($user);
return redirect()->route('dashboard');
}
// If we reach here, validation has failed
$request->flashExcept('password');
return back()->withErrors($validated);
}
}
In this example, if validation fails, we're using flashExcept()
to persist all input except the password field before redirecting back to the form.
In your Blade template, you can then use the old()
helper to repopulate the form:
<form method="POST" action="{{ route('register') }}">
@csrf
<input type="text" name="name" value="{{ old('name') }}" required autofocus>
<input type="email" name="email" value="{{ old('email') }}" required>
<input type="password" name="password" required>
<input type="password" name="password_confirmation" required>
<button type="submit">Register</button>
</form>
By mastering Laravel's flash methods, you can create more user-friendly forms that preserve input across requests while maintaining security. These methods are particularly useful for complex forms, multi-step processes, or any situation where you want to improve the user experience by not requiring users to re-enter information unnecessarily.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!