Mastering Eloquent: Eliminating Sneaky N+1 Queries with Chaperone
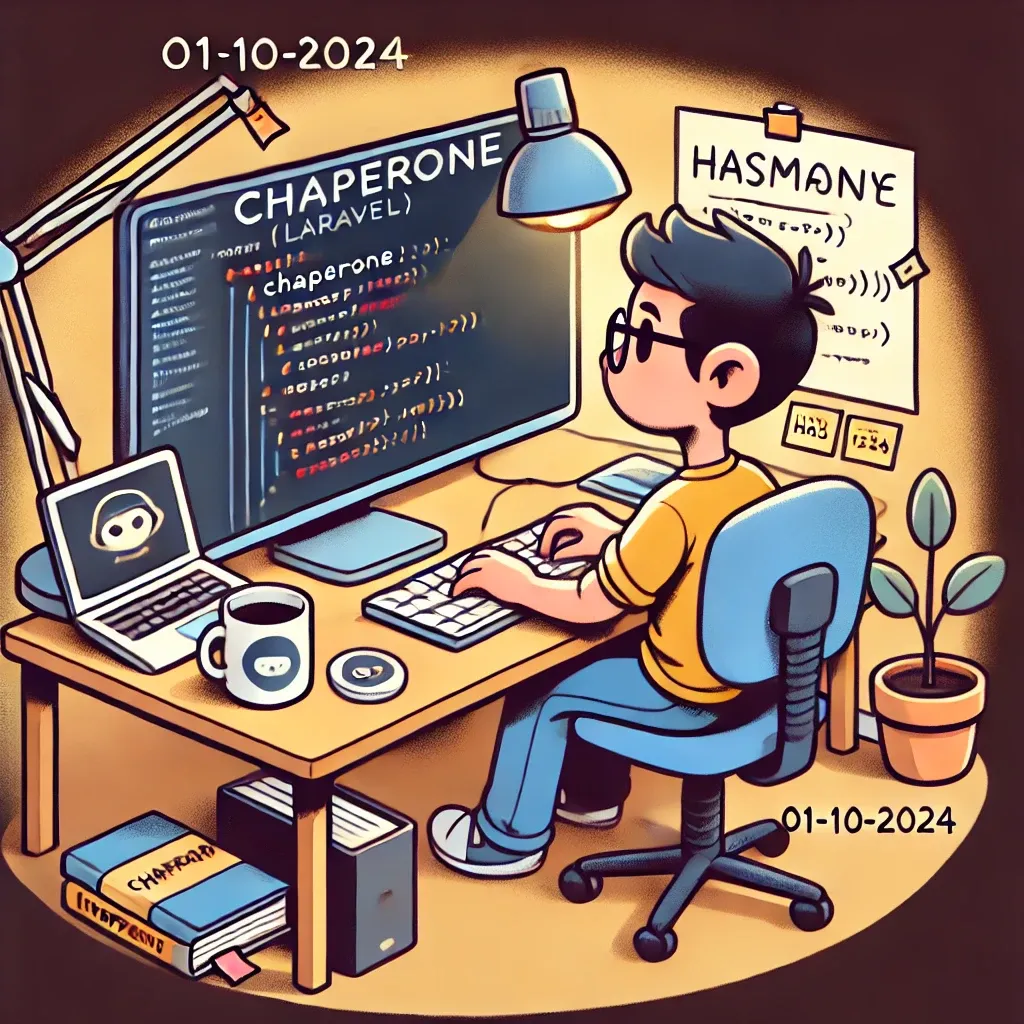
As Laravel developers, we're always on the lookout for ways to optimize our database queries. One common pitfall, even when using eager loading, is the sneaky N+1 query problem that can arise when accessing parent models from child models in nested loops. Today, we're diving into a powerful solution: the chaperone()
method.
The Problem: Hidden N+1 Queries
Consider this seemingly innocent code:
$posts = Post::with('comments')->get();
foreach ($posts as $post) {
foreach ($post->comments as $comment) {
echo $comment->post->title;
}
}
Even though we're eager loading comments, we've introduced an N+1 query problem. Eloquent doesn't automatically hydrate the parent Post
on each child Comment
model, resulting in additional queries.
The Solution: Enter Chaperone
Laravel provides an elegant solution with the chaperone()
method. Here's how to implement it:
class Post extends Model
{
public function comments(): HasMany
{
return $this->hasMany(Comment::class)->chaperone();
}
}
By adding chaperone()
to your relationship definition, Eloquent automatically hydrates the parent model on the children, eliminating those extra queries.
How It Works
When you use chaperone()
, Eloquent performs the following steps:
- Eager loads the relationship as usual.
- Automatically sets the parent model on each child model.
- Ensures that accessing the parent from a child doesn't trigger an additional query.
Real-World Example
Let's say you're building a blog system where you need to display posts with their comments and the author of each comment. Here's how you might structure your code:
class Post extends Model
{
public function comments(): HasMany
{
return $this->hasMany(Comment::class)->chaperone();
}
}
// In your controller
$posts = Post::with('comments.user')->get();
// In your view
@foreach ($posts as $post)
<h2>{{ $post->title }}</h2>
@foreach ($post->comments as $comment)
<p>{{ $comment->content }} - by {{ $comment->user->name }} on {{ $comment->post->title }}</p>
@endforeach
@endforeach
In this example, chaperone()
ensures that accessing $comment->post->title
doesn't trigger an additional query for each comment.
The chaperone()
method is a powerful tool in the Laravel developer's arsenal for combating N+1 query problems. By automatically hydrating parent models on their children, it allows you to write more efficient, performant code without sacrificing the convenience and readability of Eloquent relationships.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!