Mastering Date and Time Validation in Laravel with the "after" Rule
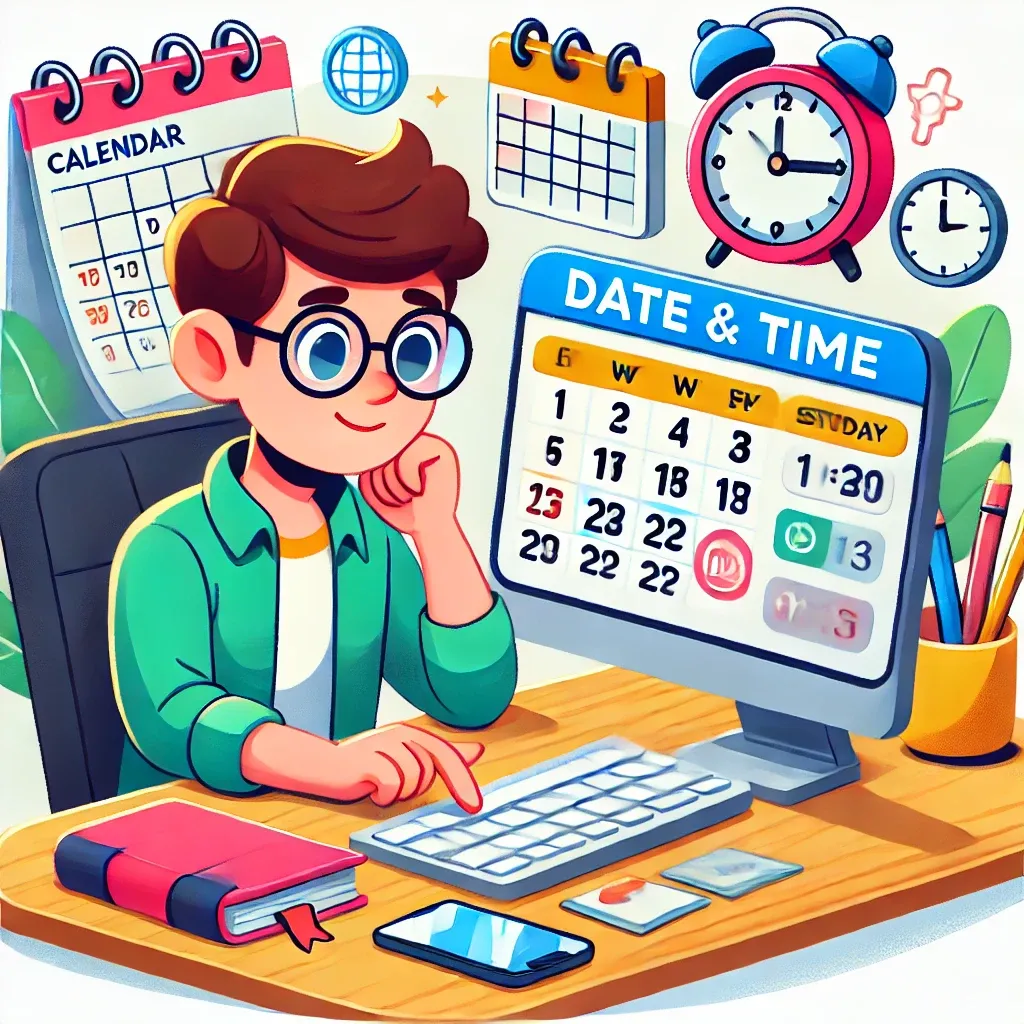
As Laravel developers, we often deal with date and time inputs in our applications. Ensuring the logical consistency of these inputs is crucial for maintaining data integrity. Laravel's "after" validation rule provides a powerful and flexible way to handle date and time comparisons. In this post, we'll explore how to leverage this rule to create more robust form validations.
Understanding the "after" Rule
The "after" rule in Laravel validation ensures that a date/time value comes after another specified date/time. This is particularly useful for scenarios like:
- Ensuring an end date is after a start date
- Validating that a date is in the future
- Creating logical sequences of events or deadlines
Basic Usage
Let's start with a simple example:
public function rules()
{
return [
'start_date' => 'required|date',
'end_date' => 'required|date|after:start_date',
];
}
In this case, the 'end_date' must be a date that comes after 'start_date'.
Advanced Techniques
Comparing Against Fixed Dates
You can compare against a specific date:
'future_event' => 'required|date|after:2023-12-31',
This ensures the 'future_event' date is sometime after December 31, 2023.
Using Date/Time Functions
Laravel allows you to use certain date/time functions with the "after" rule:
'appointment' => 'required|date|after:today',
'task_deadline' => 'required|date|after:tomorrow',
Combining with date_format
For more precise control, combine "after" with "date_format":
'meeting_time' => 'required|date_format:Y-m-d H:i|after:now',
This ensures the meeting time is both in a specific format and in the future.
Real-World Example: Event Planning System
Let's consider a more complex scenario for an event planning system:
class EventController extends Controller
{
public function store(Request $request)
{
$request->validate([
'event_name' => 'required|string|max:255',
'registration_start' => 'required|date_format:Y-m-d|after:today',
'registration_end' => 'required|date_format:Y-m-d|after:registration_start',
'event_start' => 'required|date_format:Y-m-d H:i|after:registration_end',
'event_end' => 'required|date_format:Y-m-d H:i|after:event_start',
]);
// Event creation logic here...
}
}
This validation ensures:
- Registration can't start in the past
- Registration end date is after the start date
- The event starts after registration closes
- The event end is after its start
Custom Error Messages
To provide more user-friendly error messages, you can customize them:
public function messages()
{
return [
'registration_start.after' => 'Registration cannot start in the past.',
'registration_end.after' => 'Registration must end after it starts.',
'event_start.after' => 'The event must start after registration closes.',
'event_end.after' => 'The event cannot end before it starts.',
];
}
Best Practices
- Be Specific: Use date_format when you need to enforce a particular date/time format.
- Think Ahead: Consider edge cases like timezone differences when working with dates.
- User-Friendly Messages: Provide clear error messages to guide users.
- Combine Rules: Use "after" in conjunction with other rules like "before" for range validations.
- Server-Side Validation: Always validate dates on the server-side, even if you have client-side validation.
Conclusion
The "after" validation rule in Laravel is a powerful tool for ensuring logical consistency in date and time inputs. By leveraging this rule, you can create more robust and user-friendly forms that prevent illogical date sequences and enhance data integrity in your applications. Remember, effective date validation is not just about preventing errors – it's about guiding users to provide meaningful and consistent information.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!