Mastering Currency Formatting in Laravel with the Number Helper
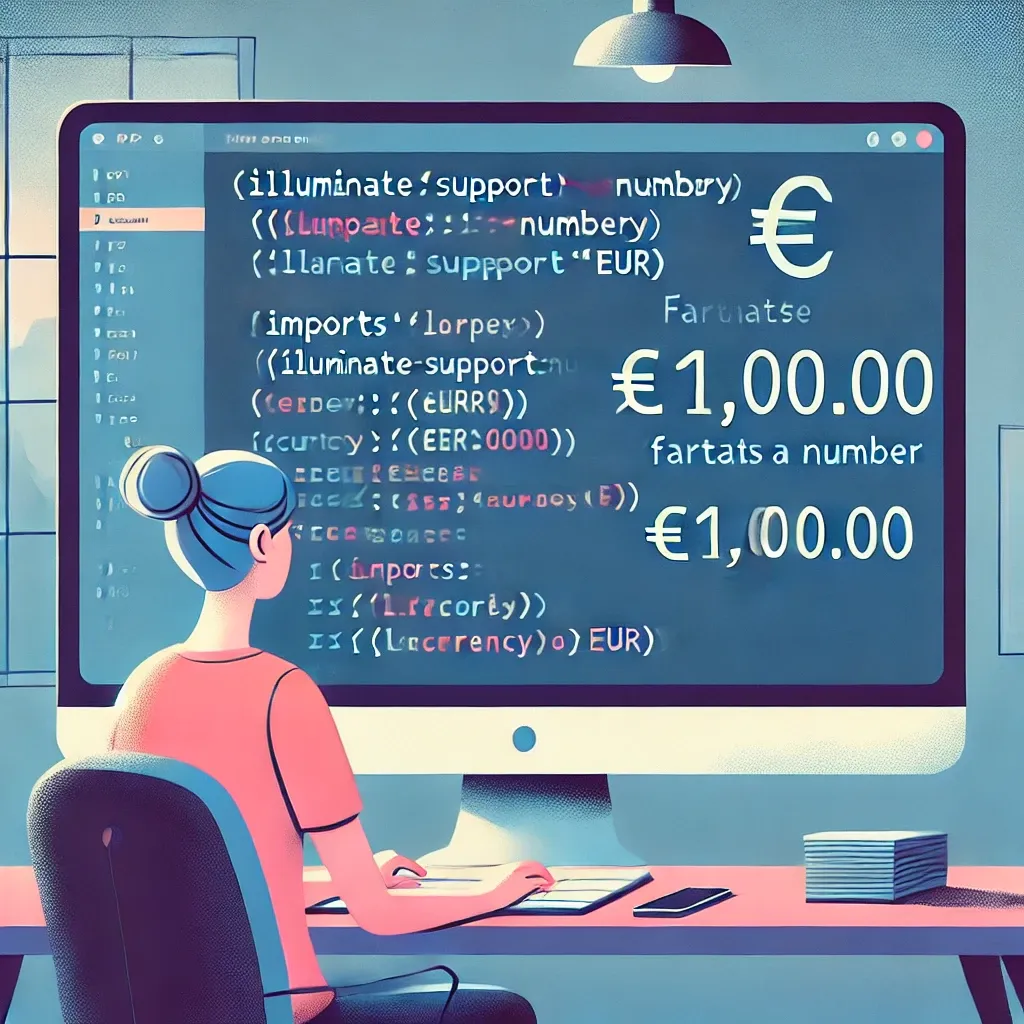
Need to handle multiple currencies in your Laravel app? The Number helper just got even better with configurable default currency settings! Let's dive into how this feature can streamline your currency formatting.
Setting a Default Currency
In the past, USD was the only default option. Now, you can easily set your preferred default currency:
use Illuminate\Support\Number;
// Set EUR as the default currency
Number::useCurrency('EUR');
// Format a number using the default (EUR)
$currency = Number::currency(1000);
// Output: €1,000.00
Temporary Currency Override
Need to use a different currency temporarily? No problem:
// Override the default just for this value
$currency = Number::currency(1000, in: 'USD');
// Output: $1,000.00
Using a Callback
You can also use a callback to handle multiple operations with a temporary currency:
Number::withCurrency('USD', function () {
$price1 = Number::currency(1000); // $1,000.00
$price2 = Number::currency(2000); // $2,000.00
// After this callback, the default (EUR) is restored
});
Real-World Example
Here's how you might use this in an e-commerce application:
class PriceController extends Controller
{
public function display(Request $request, Product $product)
{
// Set user's preferred currency as default
Number::useCurrency($request->user()->preferred_currency);
return view('products.show', [
'regular_price' => Number::currency($product->price),
'usd_price' => Number::currency($product->price, in: 'USD'),
'wholesale_prices' => Number::withCurrency('EUR', function () use ($product) {
return [
'bulk' => Number::currency($product->wholesale_price),
'distributor' => Number::currency($product->distributor_price)
];
})
]);
}
}
The Number helper's new currency features make it easier than ever to handle multiple currencies in your Laravel applications. Whether you're building an international e-commerce platform or just need to display prices in different currencies, these tools have got you covered.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!