Mastering Controller Action Redirects in Laravel
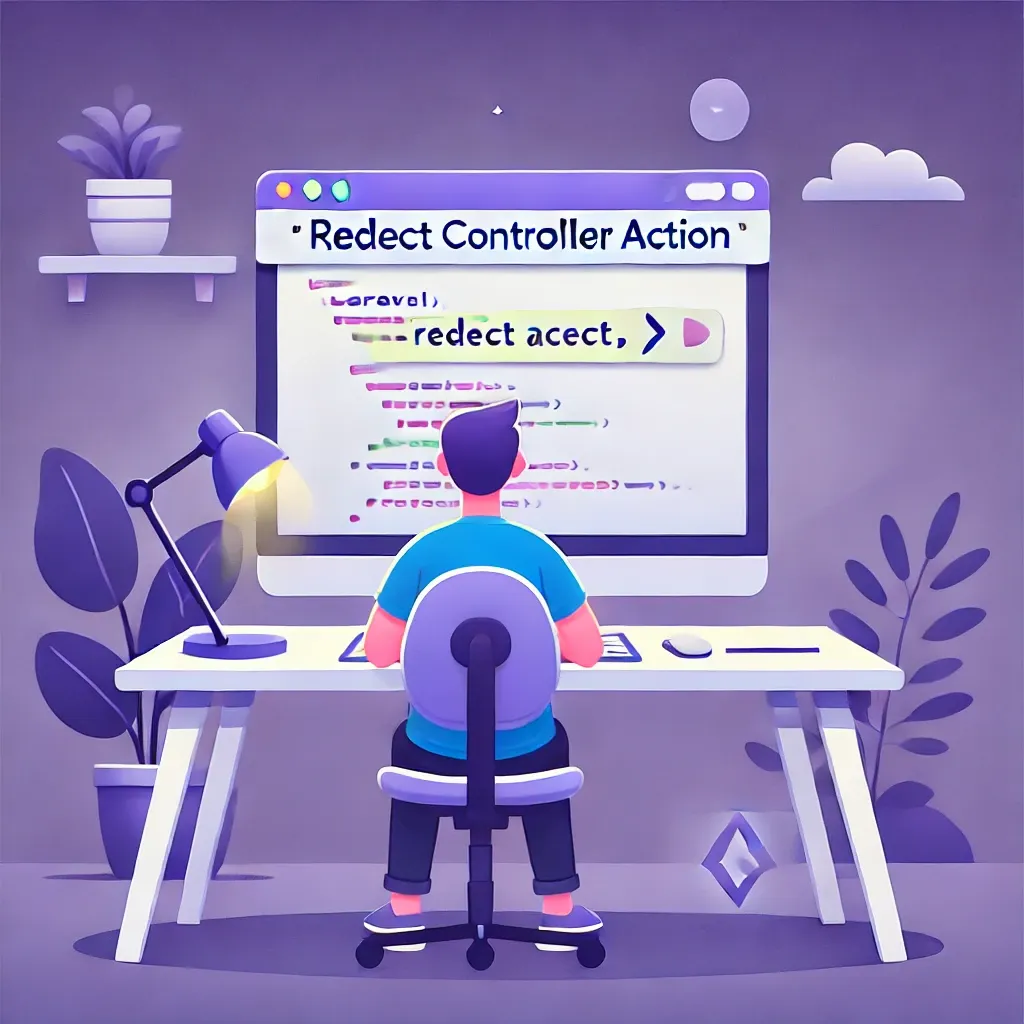
Need to redirect users to controller actions? Laravel makes it clean and simple with the action
method! Let's explore how to handle these redirects effectively.
Basic Action Redirect
Here's the simplest way to redirect to a controller action:
use App\Http\Controllers\UserController;
return redirect()->action([UserController::class, 'index']);
Redirecting with Parameters
Need to pass parameters? Just add them as the second argument:
return redirect()->action(
[UserController::class, 'profile'],
['id' => 1]
);
Real-World Example
Here's how you might use action redirects in a user management system:
class UserManagementController extends Controller
{
public function promoteUser(User $user)
{
try {
$user->promote();
return redirect()
->action([UserController::class, 'show'], ['id' => $user->id])
->with('success', 'User promoted successfully');
} catch (Exception $e) {
return redirect()
->action([UserController::class, 'index'])
->with('error', 'Failed to promote user');
}
}
public function transferDepartment(User $user, Request $request)
{
$user->transferToDepartment($request->department_id);
return redirect()->action(
[DepartmentController::class, 'members'],
['dept' => $request->department_id]
);
}
}
Action redirects provide a clean, type-safe way to redirect users within your Laravel application, especially when dealing with controller-based routing.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!