Mastering Content Negotiation in Laravel: A Deep Dive into Request Content Types
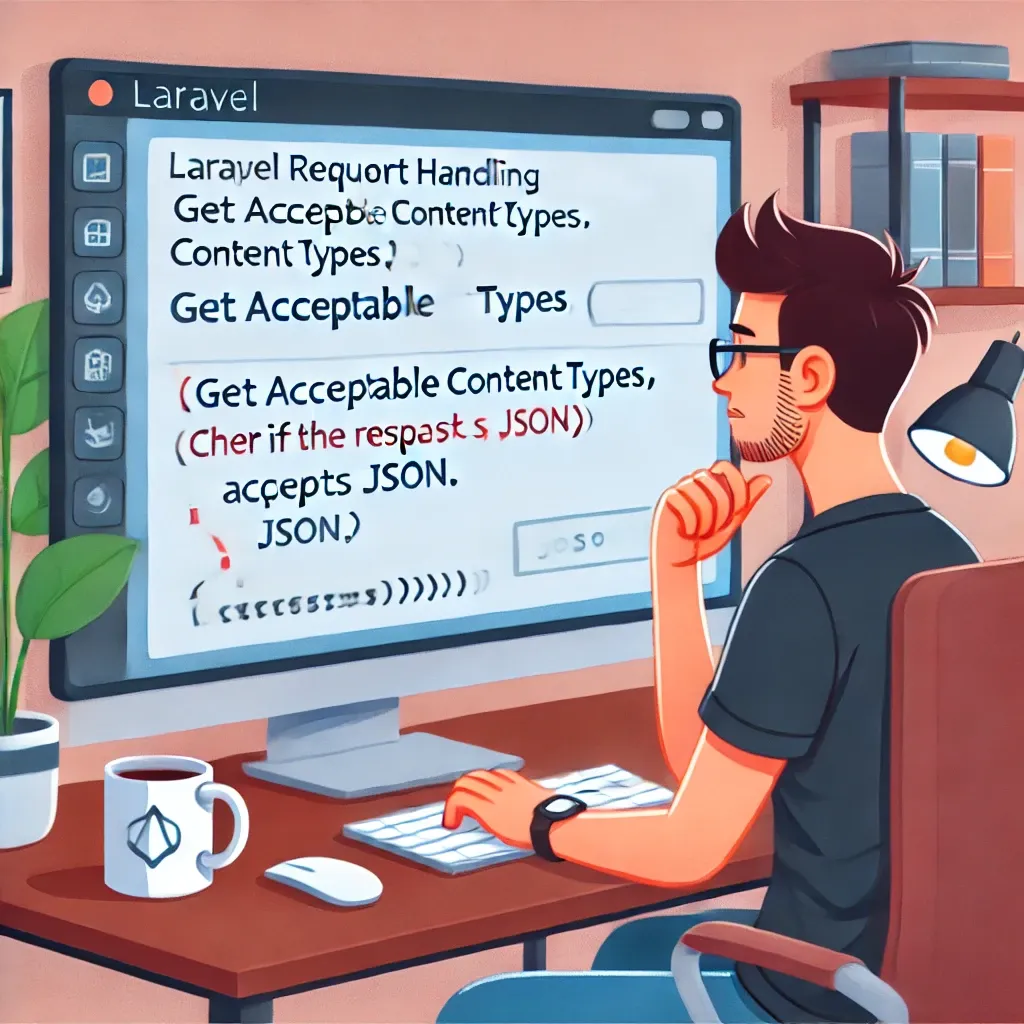
In the world of modern web development, serving content in various formats is often a requirement. Whether you're building an API that needs to respond with JSON or XML, or a web application that serves both HTML and PDF, understanding and handling the client's preferred content type is crucial. Laravel provides powerful tools to inspect and respond to the Accept
header in incoming requests. Let's explore how to leverage these tools effectively.
Understanding Content Type Inspection in Laravel
Laravel offers two primary methods for inspecting the accepted content types of an incoming request:
getAcceptableContentTypes()
: Returns an array of all content types accepted by the request.accepts()
: Checks if the request accepts any of the given content types.
Let's dive deeper into each method:
The getAcceptableContentTypes() Method
This method returns an array of all content types that the client is willing to accept:
$contentTypes = $request->getAcceptableContentTypes();
This can be useful when you need to inspect or log the full range of content types the client can handle.
The accepts() Method
The accepts()
method is more specific. It takes an array of content types and returns true
if the request accepts any of them:
if ($request->accepts(['text/html', 'application/json'])) {
// The request accepts either HTML or JSON
}
This method is particularly useful when you need to quickly check if the request can handle specific content types.
Real-Life Example
Let's consider a scenario where we're building an API that can respond with either JSON or XML, depending on the client's preference. We'll also include a fallback to HTML for web browsers.
Here's how we might implement this in a controller:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index(Request $request)
{
$users = User::all();
$contentTypes = $request->getAcceptableContentTypes();
if ($request->accepts(['application/json'])) {
return response()->json($users);
} elseif ($request->accepts(['application/xml'])) {
// Assuming we have a toXml() method on our collection
return response($users->toXml(), 200, ['Content-Type' => 'application/xml']);
} else {
// Fallback to HTML
return view('users.index', compact('users'));
}
}
}
Now, let's see how this might work with different Accept
headers:
// Accept: application/json
[
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
},
{
"id": 2,
"name": "Jane Doe",
"email": "jane@example.com"
}
]
// Accept: application/xml
<?xml version="1.0" encoding="UTF-8"?>
<users>
<user>
<id>1</id>
<name>John Doe</name>
<email>john@example.com</email>
</user>
<user>
<id>2</id>
<name>Jane Doe</name>
<email>jane@example.com</email>
</user>
</users>
// Accept: text/html
// Returns an HTML view
In this example, we're using both getAcceptableContentTypes()
(which we could use for logging or more complex decision-making) and accepts()
to determine the appropriate response format.
By mastering content type inspection in Laravel, you can build more flexible, robust applications that cater to a wide range of clients and use cases. Whether you're building an API or a multi-format web application, these tools will help you deliver the right content to the right client every time.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!